How To Make Android App Java
How to make Android app Java: Dive into the exciting world of Android app development using Java. This comprehensive guide will walk you through the essentials, from setting up your development environment to deploying your finished app to the Google Play Store. We’ll cover everything, from the fundamentals of Java programming to advanced techniques like multithreading and networking.
This detailed exploration will equip you with the knowledge and skills needed to build compelling Android applications. You’ll learn how to design intuitive user interfaces, handle user input, and manage data efficiently. Prepare to embark on a rewarding journey into Android app development.
Introduction to Android App Development with Java
Android app development has become a significant field in software engineering, enabling the creation of a wide range of applications for various devices. The demand for skilled Android developers is consistently high, driven by the ubiquitous nature of smartphones and tablets. This field offers numerous opportunities for innovation and problem-solving, with the potential to create applications that improve users’ lives and experiences.Developing Android applications using Java involves leveraging the Android Software Development Kit (SDK) and the Android Studio integrated development environment (IDE).
This approach allows developers to create robust, user-friendly applications that cater to a diverse range of user needs and preferences. The Android platform’s open-source nature further fosters innovation and community collaboration.
Fundamental Concepts of Android Development
The core of Android app development rests on understanding the Android SDK and Android Studio. The Android SDK provides a comprehensive set of tools, libraries, and APIs that developers use to build and deploy Android applications. Android Studio, a powerful IDE, simplifies the development process by offering features such as code editing, debugging, and testing.
Types of Android Applications
Android supports a wide variety of applications. These include games, productivity tools, social media platforms, and communication apps. The flexibility of the platform allows for the development of custom applications tailored to specific needs and requirements. The diverse range of applications reflects the breadth of use cases supported by the Android operating system.
Setting Up a Development Environment
Setting up a development environment for Android app creation involves several key steps. First, ensure that the necessary hardware and software components are installed. Next, download and install the Android SDK and Android Studio. Finally, configure the development environment to connect with the Android emulator or a physical device. These steps provide a solid foundation for subsequent development efforts.
Android SDK Components
The Android SDK encompasses numerous components, each serving a specific function. These components are crucial for building and deploying Android applications.
Android SDK Component | Function | Significance |
---|---|---|
Android Platform | Provides the core APIs and libraries for developing Android applications. | Essential for building functional applications. |
Android Support Library | Offers compatibility with older Android versions and provides cross-platform functionality. | Ensures application functionality across various Android versions. |
Android Tools | Includes tools for building, debugging, and testing Android applications. | Facilitates the development and testing process. |
Android Build System | Manages the compilation and packaging of Android applications. | Ensures efficient application creation and deployment. |
Android Emulator | Allows developers to test applications in a simulated Android environment without needing a physical device. | Provides a cost-effective and efficient testing platform. |
Essential Java Programming Concepts for Android
Mastering Java is fundamental to creating robust and effective Android applications. This section delves into the core Java concepts crucial for Android development, emphasizing object-oriented principles and the significance of libraries and frameworks. Understanding these concepts will empower you to build high-quality Android apps with a strong foundation.A comprehensive understanding of Java’s object-oriented programming paradigm is vital for Android app development.
This involves effectively utilizing classes, objects, methods, and data structures to design modular and maintainable applications.
Classes and Objects
Classes are blueprints for creating objects. An object is an instance of a class, possessing specific attributes and behaviors. In Android, classes define components like Activities, Services, and Views, each embodying unique functionalities. For example, a `Button` class creates `Button` objects that respond to user interactions. Understanding the relationship between classes and objects is essential for structuring your Android applications effectively.
Methods
Methods are blocks of code within a class, encapsulating specific tasks. They define the actions an object can perform. Methods in Android applications handle user input, update data, and manage various application logic. For instance, a method within a `TextView` class might update the text displayed on the screen. Understanding method signatures, parameters, and return types is crucial for writing efficient and maintainable Android code.
Data Structures
Data structures are fundamental to organizing and managing data within an application. They impact performance and efficiency. Common data structures include arrays, lists, maps, and sets. The selection of the appropriate data structure for a given task significantly affects the speed and efficiency of your Android application. A good understanding of how to choose and use data structures is crucial for optimized Android development.
Object-Oriented Programming (OOP) Principles
Object-oriented programming (OOP) principles are essential for Android app development. These principles include encapsulation, inheritance, and polymorphism. Encapsulation bundles data and methods together, promoting modularity and maintainability. Inheritance allows classes to inherit properties and behaviors from parent classes, promoting code reusability. Polymorphism enables objects of different classes to respond to the same method call in their own specific ways.
Implementing OOP principles in Android development leads to cleaner, more organized, and more maintainable code.
Java Libraries and Frameworks
Java libraries and frameworks provide pre-built functionalities, accelerating development and reducing code duplication. The Android SDK provides numerous libraries crucial for Android development, including UI components, networking capabilities, and data persistence. These libraries simplify complex tasks and ensure consistent application behavior across different Android devices. Learning to leverage these libraries is essential for efficient Android development.
Comparison of Data Structures
| Data Structure | Description | Suitability for Android ||—|—|—|| Arrays | Fixed-size collection of elements | Suitable for scenarios where the size of the data is known beforehand. || ArrayList | Dynamically sized array | Useful for collections where the size might change during runtime, common in Android. || HashMap | Key-value pairs | Ideal for storing and retrieving data based on keys, excellent for managing configurations or user data.
|| LinkedList | Sequence of elements, each linked to the next | Less common in Android development but may be useful for specific scenarios requiring frequent insertions or deletions in the middle of the list. |
Exception Handling and Error Management
Exception handling is critical for building robust Android applications. Exceptions represent errors or exceptional conditions that occur during program execution. Using `try-catch` blocks allows you to handle exceptions gracefully, preventing crashes and providing informative error messages to the user. Proper error management is crucial for creating user-friendly and reliable Android applications.
UI Design and Layout in Android Apps
Android app development hinges on creating user-friendly interfaces. Effective UI design significantly impacts user experience and app adoption. This section delves into the fundamental components of Android UI, exploring various layout structures and interactive elements. We’ll also cover how to implement different UI styles and themes, ensuring your apps are visually appealing and consistent.Understanding how to structure layouts is crucial for building well-organized and functional Android applications.
Appropriate layout choices contribute to a smooth user flow and a visually pleasing experience. This section details the core components, layout structures, and techniques for creating interactive UI elements, offering practical insights into creating polished and user-friendly Android apps.
Fundamental UI Components
Android UI components are the building blocks of your application’s visual interface. These elements allow you to display information, receive user input, and interact with the app. Key components include buttons, text fields, images, and more complex widgets. A comprehensive understanding of these components is essential for designing effective and engaging user interfaces.
Different UI Layout Structures
Android offers several layout structures to organize UI elements effectively. These layouts manage the positioning and arrangement of components, ensuring your app’s visual appeal and usability. Common layouts include LinearLayouts, RelativeLayouts, and ConstraintLayouts.
LinearLayout
A LinearLayout arranges components in a single row or column. It’s simple to implement and suitable for basic layouts where elements need to follow a predictable order. Its primary use case is to arrange elements in a sequential manner, where the order matters.
RelativeLayout
A RelativeLayout allows precise positioning of components based on other elements within the layout. This provides greater flexibility than a LinearLayout, enabling complex and dynamic layouts. It’s ideal for layouts where precise positioning and relationships between elements are essential.
ConstraintLayout
A ConstraintLayout enables complex layout designs by specifying constraints between elements. It offers greater control and flexibility than the previous two layouts, particularly in more intricate layouts with multiple components. It’s well-suited for intricate layouts requiring precise positioning and interactions.
Table of Layout Types
Layout Type | Characteristics | Use Cases |
---|---|---|
LinearLayout | Arranges elements in a single row or column. | Basic layouts, simple ordering. |
RelativeLayout | Positions elements relative to other elements. | Complex layouts, precise positioning. |
ConstraintLayout | Positions elements using constraints. | Complex layouts, precise positioning, dynamic changes. |
Creating Interactive UI Elements
To create a dynamic and interactive app, UI elements must respond to user input. Buttons, text fields, and images are crucial for building engaging user experiences.
Buttons
Buttons allow users to trigger actions within your application. Their appearance and behavior can be customized to fit the overall design.
Text Fields
Text fields enable users to input text data, a fundamental interaction in many applications. You can customize their appearance and functionality.
Images
Images enhance visual appeal and can be used to illustrate or convey information. Efficient image handling is vital for a smooth user experience.
Implementing UI Styles and Themes
Android allows for implementing styles and themes to ensure a consistent visual experience across your app. This approach promotes a cohesive look and feel.
Implementing Features and Functionality
Building a functional Android app requires careful implementation of core functionalities. This involves handling user interactions, displaying information effectively, and managing data persistently. A robust approach to these aspects is crucial for a user-friendly and reliable application.A well-designed app prioritizes user experience by providing intuitive feedback mechanisms and seamless data management. This section explores these critical aspects, detailing the process and various strategies for success.
Handling User Input and Feedback
User interactions are the lifeblood of any application. Efficient handling of user input is paramount to responsiveness and usability. Various methods exist for capturing and processing user input, such as text entry, touch gestures, and button clicks. The app must then provide appropriate feedback, ensuring users understand the system’s response to their actions. This could involve visual cues, audio alerts, or contextual messages.
Displaying Data
Displaying data effectively is key to conveying information clearly and engagingly to the user. Android provides powerful tools for structuring and presenting data in a visually appealing and informative way. These include various layout components for organizing elements like text, images, and lists. Customizing layouts enhances the app’s visual appeal and user experience.
Managing Data Persistence
Data persistence is essential for applications that need to retain user information, settings, or other data between sessions. Different methods exist to achieve this. Choosing the right method depends on the application’s specific needs. For instance, simple settings can be stored in shared preferences. More complex data often necessitates database solutions like SQLite.
Using SQLite Databases, How to make Android app Java
SQLite is a popular choice for storing structured data in Android applications. It offers a lightweight and robust database solution integrated seamlessly with Android’s development environment. Using SQLite involves defining tables, inserting data, querying data, and updating records. Developers need to manage the database schema carefully to ensure data integrity and efficient retrieval. Example queries for selecting and updating data are crucial for efficient data management.
Example: Integrating External Libraries
Integrating external libraries or APIs can extend the functionality of an Android application. Libraries provide pre-built components that address specific tasks, enhancing development speed and efficiency. For instance, a library for image processing can significantly improve the visual aspects of the app. Libraries for networking allow seamless communication with remote servers or APIs. A practical example of integrating a library would involve a map display library like Google Maps to add location services to an app.
Example: Handling Data Persistence in a Practical Context
Consider a simple to-do list app. To save the user’s tasks, SQLite could store the task descriptions, due dates, and priorities. The app would allow users to add, edit, and delete tasks, with the changes persistently saved in the database. This example demonstrates how a database facilitates the app’s functionality, ensuring data is readily available and accessible to the user.
Testing and Debugging Android Applications: How To Make Android App Java
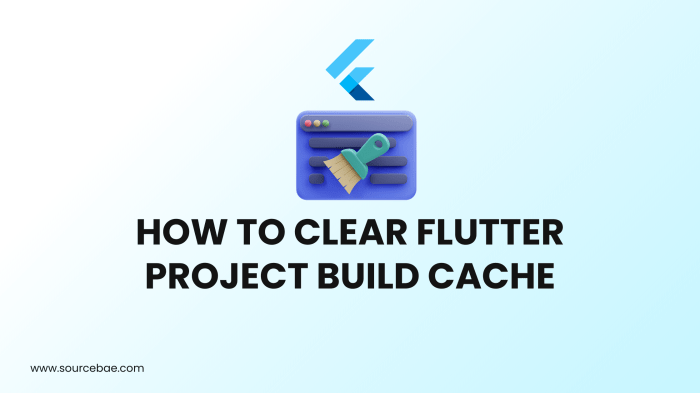
Source: sourcebae.com
Thorough testing and effective debugging are crucial for building robust and reliable Android applications. These processes ensure that the application functions as intended, handles various inputs gracefully, and is free from unexpected behavior. Rigorous testing and debugging minimize the likelihood of user frustration and potential app store rejection.The process of developing Android applications involves numerous stages, from designing the user interface to implementing core functionalities.
Each stage requires careful examination to guarantee correctness and maintainability. This meticulous approach ensures that the final product meets the specified requirements and delivers a seamless user experience.
Importance of Testing and Debugging
Comprehensive testing and debugging strategies are vital to the success of any Android application. They enable developers to identify and rectify potential issues early in the development cycle, saving significant time and resources in the long run. High-quality applications are built on a foundation of thorough testing and debugging. A robust testing process helps prevent bugs from reaching users, preserving the application’s reputation and maintaining user trust.
Types of Testing Strategies
Several testing strategies are employed in Android application development. Unit testing isolates individual components of the application, verifying their functionality in isolation. This approach ensures that each part operates correctly before integrating them into larger modules. Integration testing verifies the interaction between different components, checking if they work together seamlessly. This strategy is critical to ensure that the application’s different parts communicate and function together as intended.
Debugging Techniques
Debugging is the process of identifying and resolving errors in an Android application. Effective debugging techniques include carefully reviewing the application’s code, utilizing debugging tools provided by Android Studio, and employing logging mechanisms to track application behavior. Logging helps to identify the sequence of events that lead to an error.
Using Logging Mechanisms
Logging mechanisms are powerful tools for tracking application behavior. They provide detailed information about the application’s execution, including variables, function calls, and execution flow. Logging can help pinpoint the exact location and cause of an error, enabling swift resolution. Proper logging helps in identifying unexpected behavior and understanding the flow of the application.
Testing Methodologies in Android App Development
Testing Methodology | Description | Purpose |
---|---|---|
Unit Testing | Testing individual units (e.g., functions, classes) in isolation. | Ensuring that each unit of code functions correctly. |
Integration Testing | Testing the interaction between different units or modules. | Verifying that different components work together seamlessly. |
System Testing | Testing the entire system as a whole. | Validating that the complete application meets its requirements. |
User Acceptance Testing (UAT) | Testing by end-users to ensure the application meets their needs and expectations. | Validating the application from the user’s perspective. |
Performance Testing | Testing the application’s responsiveness and resource utilization. | Ensuring the application performs efficiently under various conditions. |
Deployment and Distribution
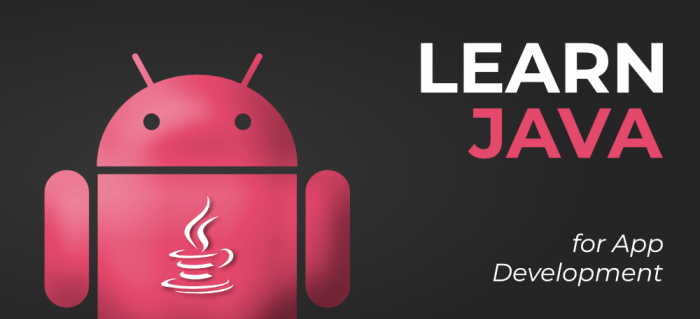
Source: geeksforgeeks.org
Publishing your Android app to the Google Play Store is a crucial step in its lifecycle. A well-structured and strategic approach to distribution, combined with app optimization, significantly impacts its visibility and success. This section details the process and key considerations.The success of an Android app hinges on its discoverability and user engagement on the Google Play Store. Effective distribution strategies, coupled with a well-optimized app, are essential for achieving this.
Publishing to the Google Play Store
The Google Play Console serves as the central hub for managing your app’s presence on the platform. It facilitates the entire publishing process, from listing your app to tracking its performance.
Distribution Strategies
Effective app promotion strategies extend beyond simply uploading your app. Utilizing marketing channels like social media, email marketing, and app store optimization (ASO) is critical for driving downloads.
- App Store Optimization (ASO): Optimizing app listings for relevant s and providing compelling descriptions can significantly improve visibility and attract potential users. This involves thorough research and precise description writing.
- Social Media Marketing: Promoting your app on relevant social media platforms like Twitter, Instagram, or Facebook can increase awareness and drive downloads. Targeted advertising campaigns can also be beneficial.
- Public Relations and Partnerships: Collaborating with influencers or media outlets in your niche can generate buzz and user interest. Partnerships with complementary apps or services can expose your app to new audiences.
App Optimization
Optimizing your app for performance and user experience is paramount. This involves reducing app size, improving loading times, and ensuring a seamless user interface.
- Code Optimization: Optimizing the underlying code can significantly reduce the app’s size and enhance performance. Employing efficient algorithms and data structures can lead to improved responsiveness and reduced resource consumption.
- Image Optimization: Optimizing images for efficient loading times and reducing file sizes. Using appropriate image formats and compression techniques are crucial for maintaining a positive user experience.
- Performance Testing: Thorough testing across various devices and network conditions is essential to ensure the app functions smoothly and efficiently under different usage scenarios. Tools like profiling and monitoring can aid in identifying and resolving performance bottlenecks.
Preparing for Publishing
Before submitting your app, ensure it meets Google Play Store guidelines. This involves meticulous attention to detail, thorough testing, and addressing potential issues.
- Complying with Guidelines: Understanding and adhering to Google Play Store policies and guidelines is critical for a successful app submission. The Play Console provides comprehensive details on these guidelines.
- App Icon and Screenshots: High-quality, visually appealing app icons and screenshots are essential for attracting users. The images should clearly showcase the app’s functionality and features.
- App Description and s: Provide a comprehensive and accurate description of your app’s functionality, features, and target audience. Use relevant s to enhance search visibility.
Step-by-Step Publishing Guide
This table Artikels the key steps in deploying an Android app to the Google Play Store:
Step | Description |
---|---|
1. Create a Google Play Console Account | Register for a Google Play Console account. |
2. Set up your App Listing | Define your app’s details, including name, description, and icon. |
3. Prepare the APK File | Package your app into an APK file and ensure it complies with guidelines. |
4. Upload APK File | Upload the APK to the Google Play Console. |
5. Review and Submit | Review the app details and submit for review by Google Play. |
6. Manage Post-Submission | Monitor and address any feedback received during the review process. |
Advanced Android Development Techniques
Mastering advanced techniques like multithreading, background tasks, and networking is crucial for building robust and responsive Android applications. These features allow your apps to perform complex operations without freezing the user interface, ensuring a smooth and engaging user experience. Efficient handling of asynchronous operations and proper network communication protocols are essential for a high-performing and reliable application.Advanced techniques are vital to scale applications and manage large amounts of data and tasks, enabling seamless background processes without affecting the user interface.
These techniques contribute significantly to the performance and stability of an application, allowing it to handle various operations effectively.
Multithreading
Multithreading is a powerful technique to perform multiple tasks concurrently. It allows different parts of your application to execute independently, improving responsiveness and performance. However, incorrect implementation can lead to data races and other concurrency issues. Careful management is critical to avoid these problems.
- Understanding Thread Safety: Ensuring that shared resources are accessed safely and consistently by multiple threads is essential. Proper synchronization mechanisms (locks, semaphores, etc.) prevent race conditions and data corruption. This prevents unexpected behavior and data inconsistencies in your application.
- Implementing Thread Pools: Using thread pools for managing threads can improve performance and resource utilization. They effectively control the number of active threads, preventing excessive overhead. This optimizes resource management and avoids overwhelming the system with too many threads.
- Handling Thread Interruption: Implement proper mechanisms to interrupt threads when needed. This prevents threads from running indefinitely, ensuring your application remains responsive and efficient.
Background Tasks
Background tasks are essential for performing operations that don’t require immediate user interaction. Examples include fetching data from a server, processing images, or performing complex calculations. Correctly handling background tasks prevents blocking the main thread and ensures smooth user interaction.
- Using AsyncTask: The AsyncTask class provides a simple way to perform background tasks without blocking the main thread. It handles the lifecycle of the background task and automatically updates the UI when necessary. This approach is effective for basic background operations.
- Employing Handler Threads: The Handler class allows you to post messages to a different thread, enabling you to perform operations on a separate thread and update the UI on the main thread. This offers more control over background tasks, especially when complex tasks are involved.
- Utilizing Services: For long-running background tasks, Android services are a robust solution. They allow your app to run in the background even when the user isn’t interacting with it. This ensures continuous background operations without affecting the user experience.
Networking
Network communication is vital for modern Android applications. Implementing reliable and efficient network communication protocols is crucial for seamless data exchange.
- Employing Volley: The Volley library provides an efficient way to handle network requests, improving performance and reliability. It simplifies network interactions by handling common network tasks. This is beneficial for applications requiring frequent network access.
- Using Retrofit: Retrofit is a powerful library for creating RESTful APIs. It allows you to easily interact with APIs and handle different HTTP methods (GET, POST, PUT, DELETE). This is a robust approach for applications with complex API integrations.
- Understanding Network Protocols: Knowledge of HTTP, HTTPS, and other network protocols is vital for effective network communication. This allows for the handling of various network configurations and ensuring data integrity.
Handling Asynchronous Operations
Asynchronous operations are crucial for responsive applications. Efficiently handling asynchronous tasks is critical for maintaining a smooth user interface.
- Using callbacks: Callbacks are simple functions that are invoked when an asynchronous operation completes. They provide a way to handle results from background tasks without blocking the main thread. This is a fundamental approach for asynchronous operations.
- Employing Promises or RxJava: Promises and RxJava offer a more structured way to handle asynchronous operations. They allow you to chain operations and manage complex asynchronous workflows. This is ideal for applications requiring more advanced asynchronous task management.
Common Multithreading Pitfalls and Solutions
Pitfall | Solution |
---|---|
Data races | Synchronization mechanisms (locks, semaphores) |
Deadlocks | Careful thread coordination and resource management |
Starvation | Prioritize tasks, use thread pools |
Incorrect thread switching | Ensure proper handling of thread contexts |
Last Point
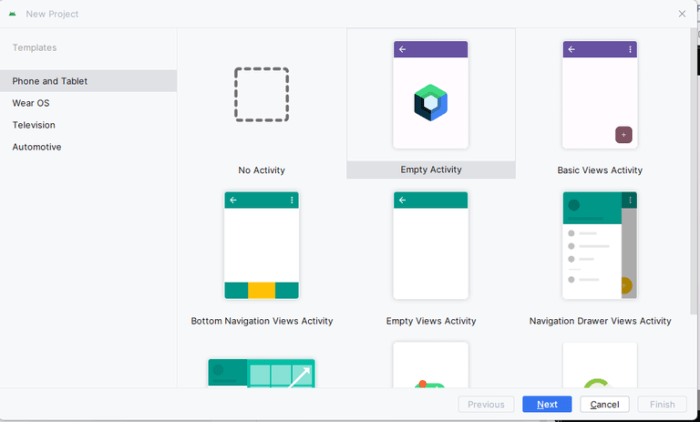
Source: geeksforgeeks.org
In summary, building Android apps with Java is a rewarding process. From initial setup to final deployment, this guide has provided a thorough roadmap. Remember to practice consistently, explore various resources, and don’t hesitate to seek help when needed. With dedication and the right resources, you’ll be well on your way to creating amazing Android applications.
Post Comment