How To Write Clean Maintainable Code
How to write clean maintainable code is crucial for any developer aiming to build robust and scalable applications. This guide delves into the essential principles, practices, and tools needed to craft code that’s not only functional but also easy to understand, modify, and extend over time. From naming conventions to version control, we’ll explore every facet of clean code development.
Understanding the fundamentals of clean code, including its principles and benefits, is paramount. This guide provides a structured approach, covering naming conventions, design patterns, testing, refactoring, and collaboration. We’ll also touch on the importance of choosing the right tools and technologies to further enhance code quality and maintainability. This practical guide will equip you with the knowledge and skills to write cleaner, more maintainable code.
Understanding Clean Code Principles
Clean code is a cornerstone of software development, ensuring that code is readable, maintainable, and testable. It’s not just about writing code that works, but about writing code that’s easy to understand, modify, and extend over time. This is crucial for projects of any size, from small personal projects to large enterprise applications. Clear and consistent code reduces errors and makes future development much smoother.Clean code principles are vital for producing high-quality software.
By adhering to these principles, developers can create code that is more robust, easier to maintain, and less prone to errors. This ultimately leads to greater efficiency and reduced costs in the long run.
Definition of Clean Code
Clean code is characterized by its readability, maintainability, and adherence to established coding standards. It prioritizes simplicity, clarity, and efficiency. A primary goal is to minimize complexity, making the codebase easier to understand and modify. This is achieved through clear variable names, well-structured functions, and modular design. The emphasis is on making the code easy to comprehend for both the original author and other developers who might work with it in the future.
Core Principles of Clean Code
Several key principles underpin clean code. Readability, maintainability, and testability are essential. These characteristics allow for efficient collaboration, easy debugging, and effortless updates to the code. Furthermore, consistent coding styles enhance the overall clarity and professionalism of the project.
- Readability: Code should be easily understood by others. This involves using meaningful variable names, concise functions, and clear comments where necessary. A well-structured codebase will have a significantly lower cognitive load for anyone who reads it. This is crucial for collaboration and maintenance. For instance, using “customerName” instead of “cName” immediately conveys the variable’s purpose, making the code more transparent.
- Maintainability: Clean code is designed to be easily modified and updated. This is achieved through modular design, clear separation of concerns, and adherence to established coding conventions. The codebase will be more resilient to changes and updates, reducing the likelihood of introducing bugs during modifications.
- Testability: Well-structured, modular code is significantly easier to test. This means it’s more reliable and less prone to bugs. This aspect also contributes to code maintainability, making it more resistant to errors and easier to update.
Importance of Consistent Coding Style
A consistent coding style enhances readability and maintainability. It creates a unified visual presentation for the codebase, making it more intuitive for anyone who reads it. This consistency is crucial for understanding the code’s logic and structure. Using consistent formatting (e.g., indentation, spacing) makes the codebase more visually appealing and easier to comprehend. This contributes to the overall professional image of the project.
Examples of Violating Clean Code Principles
Poorly written code can severely hinder the maintainability and readability of a project. Examples include:
- Long, complex functions: Functions that perform multiple unrelated tasks are harder to understand and maintain. This is problematic because a complex function may have more than one reason to change. Such functions should be broken down into smaller, more focused functions to enhance readability and testability.
- Unclear variable names: Using cryptic or generic names for variables makes it difficult to understand their purpose. This leads to confusion and potential errors when modifying the code.
- Lack of comments: A lack of comments can make code difficult to comprehend. Comments are vital for explaining complex logic or the purpose of a particular section of code. This is especially important when dealing with non-trivial code or collaborating with others.
Benefits of Clean, Maintainable Code
Clean, maintainable code yields significant benefits. These benefits encompass improved efficiency, reduced errors, and enhanced collaboration.
- Reduced Development Time: Clean code is easier to understand and modify, leading to reduced development time. This is particularly true in projects with multiple developers.
- Improved Code Quality: Clean code is less prone to errors, leading to higher quality software.
- Enhanced Collaboration: Clean code makes it easier for developers to collaborate on a project.
Clean Code Principles and Their Impact on Code Quality
The table below illustrates the impact of adhering to clean code principles on code quality.
Clean Code Principle | Impact on Code Quality |
---|---|
Readability | Improved understanding, reduced errors, and enhanced collaboration. |
Maintainability | Easier modifications, updates, and bug fixes, reduced development time. |
Testability | Enhanced reliability, reduced defects, and improved code quality. |
Consistent Coding Style | Improved readability, reduced cognitive load, and enhanced professionalism. |
Naming Conventions and Structure
Effective naming conventions and code structure are crucial for maintaining clean, readable, and understandable codebases. Consistent and descriptive naming practices, coupled with a well-organized code structure, significantly improve collaboration and reduce the time spent on debugging and maintenance. This section dives into these essential aspects of clean code.Well-structured code, coupled with meaningful names, is a cornerstone of maintainability.
A robust naming convention coupled with logical code organization minimizes the cognitive load on developers working with the codebase, ultimately reducing errors and improving overall project success.
Variable Naming Conventions
Consistent variable naming improves readability and reduces the chance of errors. Clear and concise names, reflecting the variable’s purpose, contribute significantly to the maintainability of the code. Using a standardized naming convention enhances collaboration and reduces misunderstandings among team members.
- Use descriptive names that clearly indicate the variable’s role. Avoid abbreviations unless they are widely understood within the project context.
- Employ camelCase for variables, starting with a lowercase letter. For example, `userName`, `productPrice`, `customerID`.
- Avoid single-letter variable names except for very simple cases (e.g., loop counters). `i`, `j`, and `k` are common examples for loop counters.
Function Naming Conventions
Function names should clearly express the function’s purpose. Meaningful names enhance code comprehension and reduce the need for extensive comments. Employ verbs or verb phrases to indicate the action performed by the function.
- Use verb phrases or verbs for function names, indicating the action the function performs. Examples include `calculateTotal`, `displayMessage`, `validateInput`.
- Employ camelCase for function names, starting with a lowercase letter. For example, `calculateSum`, `displayError`, `processRequest`.
- Be precise. The name should accurately reflect the function’s behavior. Avoid vague or ambiguous names.
Class Naming Conventions
Class names should clearly reflect the class’s purpose. They should use nouns or noun phrases to denote the concept represented by the class.
- Use nouns or noun phrases to describe the class. For example, `Customer`, `Product`, `Order`.
- Employ PascalCase for class names, starting with an uppercase letter. For example, `CustomerOrder`, `ProductInventory`, `ShoppingCart`.
- Use a consistent naming style for related classes to maintain a cohesive structure.
Code Structure and Organization
Well-organized code is essential for maintainability. Employing modularity, separating concerns, and using appropriate structures (e.g., classes, functions) enhances code readability and reusability. A structured codebase is easier to debug and adapt to future changes.
- Employ functions to encapsulate specific tasks. This promotes modularity and reduces code duplication.
- Organize classes logically based on their responsibilities. Separate concerns into distinct classes or modules.
- Use meaningful file and directory names to reflect the code’s structure.
Comments and Documentation
Comments and documentation are vital for explaining complex logic or non-obvious aspects of the code. Clear documentation helps other developers understand and maintain the code.
- Use comments to explain complex algorithms or non-obvious code sections. Avoid excessive commenting.
- Use documentation to explain the purpose, parameters, return values, and usage of functions and classes. Employ tools like Javadoc or similar documentation generators.
Example: Effective Code Documentation
“`java/ * Calculates the area of a rectangle. * @param length The length of the rectangle. * @param width The width of the rectangle. * @return The area of the rectangle. */public double calculateRectangleArea(double length, double width) return length – width;“`
Naming Conventions Table
Name Type | Guidelines | Examples |
---|---|---|
Variables | Descriptive, camelCase, avoid single-letter names (except loop counters). | `userName`, `productPrice`, `customerID`, `totalOrders` |
Functions | Verb phrases, camelCase, precise, indicative of action. | `calculateTotal`, `displayMessage`, `validateInput` |
Classes | Nouns/noun phrases, PascalCase. | `Customer`, `Product`, `Order`, `ShoppingCart` |
Code Design Patterns and Best Practices
Effective software development hinges on the ability to create maintainable, scalable, and reusable code. Design patterns provide a structured approach to tackling common programming challenges. Understanding and applying these patterns empowers developers to build robust applications with less effort and higher quality. This section explores common design patterns, their application, and the benefits they offer.Applying design patterns to create clean and maintainable code is a key skill for developers.
This section provides a structured approach to understanding and implementing these patterns. The discussion will cover the benefits of using these patterns, how to avoid common code smells, and the critical role of code modularity.
Common Design Patterns
Design patterns represent proven solutions to recurring software design problems. They provide a common language and framework for developers, leading to more consistent and predictable code. Recognizing and applying these patterns leads to cleaner, more maintainable codebases.
- Singleton Pattern: This pattern ensures that a class has only one instance and provides a global point of access to it. Useful for managing resources that should be accessed and modified only once, like database connections or configuration settings. For instance, a logging service might be implemented as a singleton to ensure only one instance exists for handling log entries.
- Factory Pattern: This pattern creates objects without specifying the exact class of object that will be created. This enhances code flexibility and maintainability. Imagine a system needing various types of report generators. Using a factory pattern decouples the report generation logic from the specific report type, enabling adding new report types without altering the core application logic.
- Observer Pattern: This pattern defines a one-to-many dependency between objects so that when one object changes state, all its dependents are notified and updated automatically. A typical example is a stock ticker application where multiple clients subscribe to updates for specific stocks. Any change in stock price will automatically notify all subscribers.
- Strategy Pattern: This pattern lets you define a family of algorithms, encapsulate each one, and make them interchangeable. This allows the algorithm to be selected at runtime without changing the client code. For instance, in a payment processing system, you could have different payment gateways (e.g., credit card, PayPal) implemented as separate strategies. The system could choose the appropriate strategy based on the user’s selection.
Applying Design Patterns
Successfully implementing design patterns requires careful consideration of the specific context and problem they address. Choosing the right pattern is crucial for achieving clean and maintainable code.
- Identify the Problem: Before applying a pattern, clearly define the recurring design problem you want to solve. Analyze the existing code for areas where the pattern can enhance maintainability and reduce complexity.
- Encapsulation: Encapsulate the core logic within the pattern to promote code modularity. This isolates components and minimizes the impact of changes. This approach enhances the maintainability and reduces potential conflicts.
- Decoupling: Design patterns often help decouple components, making the code more flexible and less dependent on specific implementations. This approach enhances the overall maintainability and reduces dependencies between modules.
Avoiding Code Smells
Code smells are indicators of potential problems in the codebase. Identifying and addressing them early can prevent future maintenance headaches.
- Long Methods: Methods that perform multiple unrelated tasks should be broken down into smaller, more focused methods. This improves readability and maintainability.
- Large Classes: Classes that contain excessive responsibilities should be refactored into smaller, more focused classes. This approach enhances the overall code organization and makes the codebase more maintainable.
Code Modularity
Modularity is a cornerstone of clean code. Breaking down a system into smaller, independent modules improves code organization, reusability, and maintainability.
Comparison of Design Patterns
Pattern | Use Case | Advantages |
---|---|---|
Singleton | Managing resources with a single instance | Global access, reduced resource consumption |
Factory | Creating objects without specifying the exact class | Flexibility, maintainability, easy extension |
Observer | One-to-many dependency between objects | Real-time updates, loose coupling |
Strategy | Defining a family of algorithms, interchangeable | Flexibility, maintainability, runtime selection |
Testing and Debugging
Thorough testing and effective debugging are crucial for producing robust and maintainable software. They act as quality gates, ensuring that code functions as intended and that any issues are addressed swiftly and efficiently. This process not only prevents bugs from reaching production but also helps to identify potential design flaws early on, ultimately improving the code’s overall quality.
Importance of Unit Testing in Maintaining Clean Code
Unit testing, focusing on the smallest testable units of code (functions or methods), is essential for maintaining clean code. It promotes modularity and reduces the ripple effect of changes. When units are tested in isolation, it’s easier to identify and fix problems without disrupting other parts of the system. This promotes maintainability, as the impact of modifications is more predictable.
Moreover, comprehensive unit tests serve as valuable documentation, explaining how each unit should function.
Different Testing Methodologies for Clean Code
Several testing methodologies can be employed to ensure clean code. These include:
- Unit Testing: Testing individual components in isolation. This is crucial for verifying the correctness of each function or method, minimizing the impact of changes on other parts of the codebase.
- Integration Testing: Testing how different units interact and work together. This is vital for ensuring that the components integrate correctly, and for identifying any conflicts or inconsistencies between modules.
- System Testing: Testing the entire system as a whole. This helps to identify broader issues and ensure the system behaves as expected under various conditions.
- Acceptance Testing: Testing the system from the user’s perspective. This involves evaluating whether the system meets the requirements and expectations of end-users.
Effective Debugging Techniques for Clean Code
Debugging is an integral part of the development process. Effective debugging techniques include:
- Isolating the Problem: Identifying the specific part of the code causing the issue. This often involves using print statements or logging to trace the execution flow.
- Reproducing the Issue: Creating a consistent way to reproduce the bug. This ensures that the issue is not a fluke and can be analyzed thoroughly.
- Analyzing Error Messages: Carefully reviewing error messages to understand the root cause. These messages often provide valuable clues about the problem’s origin.
- Using Debugging Tools: Leveraging debugging tools like breakpoints, step-by-step execution, and variable inspection to understand the code’s behavior during runtime.
Examples of Well-Structured Test Cases
Well-structured test cases follow a clear pattern. They specify the input, expected output, and assertions to verify the outcome. Consider this example for a function that calculates the area of a rectangle:“`java// Test case for rectangle area calculation@Testpublic void testCalculateRectangleArea() Rectangle rect1 = new Rectangle(5, 10); assertEquals(50, rect1.calculateArea()); Rectangle rect2 = new Rectangle(0, 2); assertEquals(0, rect2.calculateArea()); Rectangle rect3 = new Rectangle(-2, 5); assertEquals(0, rect3.calculateArea()); //Handles invalid input“`These examples clearly define the inputs and the corresponding expected outcomes.
How to Handle Errors and Exceptions Gracefully
Robust code anticipates and handles errors and exceptions. Employ try-catch blocks to catch potential exceptions, providing informative error messages to the user and preventing program crashes. This practice ensures a more user-friendly experience and improves the overall reliability of the application. Logging exceptions is a crucial step for debugging and monitoring.
Comparison of Testing Frameworks
Framework | Suitability for Clean Code | Pros | Cons |
---|---|---|---|
JUnit | High | Mature, widely used, extensive documentation | Can be verbose for simple tests |
Mockito | High | Excellent for mocking dependencies | Steeper learning curve |
TestNG | Medium | Flexible annotations, good reporting | Less widely used than JUnit |
Refactoring and Code Improvement: How To Write Clean Maintainable Code
Refactoring is a crucial aspect of software development. It involves restructuring existing code without altering its external behavior. This process enhances code maintainability, readability, and efficiency, making future modifications and enhancements easier. Proper refactoring is essential for long-term project success and allows developers to adapt to evolving requirements without compromising code quality.Refactoring aims to improve the internal structure of the codebase, making it more robust, understandable, and easier to maintain.
This is achieved by identifying and addressing code smells – patterns that indicate potential problems or areas for improvement. Identifying and fixing these issues early on prevents them from escalating into larger problems down the line.
Code Smells and Refactoring Opportunities
Code smells are indicators of potential problems within the code. Identifying and addressing them proactively during refactoring helps to prevent issues from escalating and ensures maintainable code. Common code smells include excessive nesting, long methods, duplicated code, and complex conditional logic. These are often subtle signs that require careful examination and systematic analysis to identify the root cause.
Strategies for Identifying Code for Refactoring
Systematic analysis and examination of the codebase are essential for pinpointing areas needing improvement. A methodical approach is necessary for a successful refactoring process.
- Code Reviews: Peer reviews can provide valuable insights. Experienced developers can often spot potential problems or areas for improvement that might be missed by the original author.
- Static Analysis Tools: These tools can automatically scan the codebase, flagging potential code smells and areas of concern, like excessive complexity, or violations of coding standards. Tools like SonarQube and PMD offer valuable assistance in this regard.
- Code Metrics: Analyzing code metrics like cyclomatic complexity, lines of code, and method length can highlight code sections that are overly complex or difficult to maintain. This data provides valuable information on the structural health of the code.
Refactoring Techniques, How to write clean maintainable code
Various refactoring techniques can be employed to improve the code’s structure and maintainability. Applying the appropriate technique is critical for ensuring a smooth and effective refactoring process.
- Extract Method: This technique involves extracting a portion of code from a larger method into a new, smaller, and more focused method. This enhances readability and reduces redundancy.
- Extract Variable: This involves extracting a complex expression into a named variable. This makes the code more readable and easier to understand.
- Introduce Parameter Object: This refactoring technique is used to reduce the number of parameters passed to a method by encapsulating related parameters into a new object. This makes the method signature cleaner and more understandable.
- Replace Conditional with Polymorphism: This approach replaces complex conditional logic with a more object-oriented approach using polymorphism, which leads to cleaner and more maintainable code. This is especially beneficial for code with many conditions and conditional branches.
Code Analysis Tools
Code analysis tools are powerful aids in the refactoring process. They automate the detection of code smells and potential problems, thereby improving the overall quality of the codebase.
- SonarQube: This open-source platform provides a comprehensive set of tools for code quality analysis, including code smells detection, complexity analysis, and code coverage measurement. SonarQube can also integrate with various IDEs and CI/CD pipelines.
- PMD (Program Comprehension Metrics): PMD is a tool that identifies potential problems in Java, JavaScript, and other languages. It helps to improve the code’s quality and maintainability by detecting code smells, and code style violations.
- FindBugs: This tool identifies bugs and potential problems in Java code, assisting in preventing future issues.
Example of Refactoring
Imagine a method that calculates the total price of an order with several complex discounts. This method could be refactored using the Extract Method pattern. The original method would be broken down into smaller, more focused methods that each handle a specific aspect of the calculation. This modular approach significantly enhances readability and allows for better understanding of each step in the calculation.
Version Control and Collaboration
Version control systems are crucial for managing code effectively, especially in collaborative environments. They provide a historical record of changes, enabling easy tracking of modifications, reverting to previous versions, and facilitating collaboration among developers. This crucial aspect of software development significantly improves code maintainability and reduces errors.
Importance of Version Control Systems for Clean Code
Version control systems (VCS) are essential for maintaining clean code. They track changes to the codebase, allowing developers to revert to previous versions if needed. This historical record is invaluable for debugging, identifying the source of errors, and understanding the evolution of the code. A well-maintained VCS history demonstrates the development process’s transparency and clarity, contributing significantly to maintainability.
Best Practices for Using Git and Other Version Control Systems
Following best practices when using VCS like Git ensures smooth collaboration and code integrity. Regular commits with descriptive messages are essential for understanding the changes made. Commits should be small, focused on a specific task or change, rather than large, encompassing commits. This granular approach enhances code readability and facilitates tracking of modifications. Pushing code frequently to a central repository enables others to access the latest version and allows for early detection of potential issues.
Effective Collaboration on Code Projects
Effective collaboration relies on clear communication and shared understanding of the codebase. Establishing clear communication channels, such as project management tools, email, or dedicated chat groups, promotes seamless collaboration. Regular code reviews are essential for identifying potential issues, ensuring code quality, and fostering knowledge sharing among team members. A well-defined workflow, outlining the steps for code contribution and review, streamlines the development process and promotes consistency.
Effective Branching Strategies
Branching strategies in Git are vital for managing different features or bug fixes independently. The Gitflow model, for example, employs feature branches to develop new features and hotfixes to address urgent issues, maintaining a clear separation between development and production code. A well-defined branching strategy minimizes the risk of merging conflicts and ensures that the main branch remains stable.
Resolving Conflicts
Conflict resolution is a common occurrence in collaborative coding. When conflicts arise, using the Git command-line tools and understanding the conflict markers is crucial. Developers should carefully review the changes in the conflicting areas, understanding the different versions and choosing the appropriate one. Effective communication and collaboration between team members during conflict resolution are essential to resolve them quickly and efficiently.
Comparison of Version Control Systems
Version Control System | Features | Pros | Cons |
---|---|---|---|
Git | Distributed, branching, merging, staging, remote repositories | Highly flexible, powerful branching model, distributed nature, extensive community support | Steeper learning curve for beginners, complex command-line interface |
Subversion (SVN) | Centralized, versioning, branching (limited) | Simple to use, good for small teams, robust centralized structure | Limited branching capabilities, less flexibility for large projects, single point of failure |
Mercurial | Distributed, lightweight, branching, merging | Easy to use, lightweight, good for smaller projects | Smaller community support compared to Git, less mature features |
Code Reviews and Feedback
Code reviews are a crucial step in the software development lifecycle, ensuring code quality, maintainability, and adherence to established standards. They provide a structured environment for developers to learn from each other, identify potential issues early, and foster a shared understanding of the codebase. Effective code reviews significantly improve the overall quality and robustness of the software.Code reviews, when conducted systematically and with thoughtful feedback, help to minimize errors, enhance code clarity, and improve maintainability.
This process, when properly implemented, cultivates a culture of shared responsibility and collective improvement.
Code Review Process
Code reviews typically involve a developer sharing their code with one or more colleagues for review. This can take place using a version control system, where the code is submitted for review, or through direct sharing of files. The reviewers then examine the code for compliance with coding standards, potential errors, and overall effectiveness. Reviews should aim to uncover any areas where the code could be improved, whether it be in terms of clarity, efficiency, or adherence to established design principles.
Benefits of Code Reviews
Code reviews offer multiple benefits to the development process. They foster collaboration among team members, leading to a deeper understanding of the codebase and improved communication. Early identification of potential bugs or design flaws helps prevent costly fixes later in the development cycle. By providing constructive feedback, reviews help improve code quality and maintainability, leading to a more robust and sustainable software product.
Finally, reviews serve as a valuable learning opportunity for all developers involved, promoting knowledge sharing and skill enhancement.
Effective Feedback Mechanisms
Providing constructive feedback is crucial to the success of code reviews. The goal is not to criticize but to offer suggestions and improvements. Focus on specific issues, rather than general statements. Use precise language to explain what’s not ideal and propose clear, actionable solutions. Examples include “This function could be more efficient by using a loop instead of recursion” or “The variable names are not very descriptive, consider renaming them to something more meaningful.” Using specific examples and references to relevant code guidelines helps to make the feedback more concrete and actionable.
Soliciting and Providing Feedback
Soliciting feedback involves clearly defining the purpose and scope of the review. Providing clear and concise feedback involves using specific examples, supporting your suggestions with reasoning, and offering alternative solutions. Avoid making sweeping statements or generalizations. When providing feedback, use a respectful tone and focus on improvement rather than criticism. Constructive criticism is essential to help developers understand areas where their code can be enhanced.
Creating a Culture of Code Reviews
Establishing a culture of code reviews requires commitment from all team members. The process should be integrated into the development workflow, making it a routine and expected part of the development process. Training and guidance on effective code review techniques can help developers understand the importance of the process and how to contribute effectively. Encourage open communication and collaboration to foster a supportive environment where developers feel comfortable offering and receiving feedback.
Regular meetings and discussions about the code review process can help to address issues and improve the effectiveness of the review process.
Code Review Metrics
| Metric | Importance ||—|—|| Number of defects found per review | High – Indicates the effectiveness of the review process in identifying potential issues early. A high number suggests a need to improve training or review processes. || Time taken per review | Moderate – Balancing review thoroughness with efficient use of time. Excessive review time may indicate a need to streamline the process or improve code readability.
|| Number of actionable suggestions per review | High – Reflects the quality and usefulness of the feedback provided. A low number may indicate a need to improve feedback clarity and guidance. || Average time to fix defects | Moderate – Indicates how quickly identified issues are addressed. High times could signal a need to prioritize bug fixes or improve communication between developers. || Code review completion rate | High – Demonstrates team commitment and adherence to the review process.
Low completion rates could signal issues in workflow or scheduling. |
Choosing Appropriate Tools and Technologies
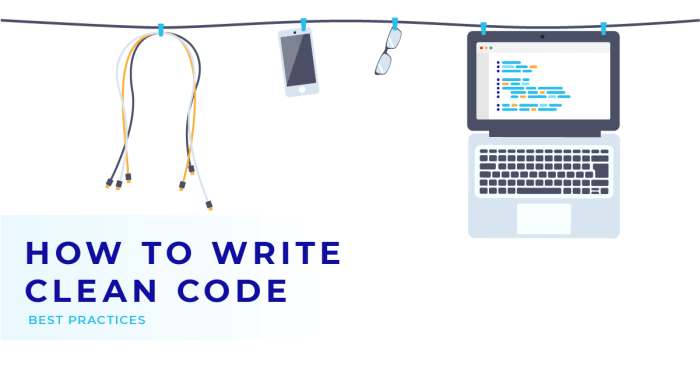
Source: waverleysoftware.com
Selecting the right tools and technologies is crucial for building high-quality, maintainable code. The choice directly impacts development efficiency, code readability, and long-term project success. Properly chosen tools can streamline tasks, enhance collaboration, and facilitate the application of best practices.Appropriate tools and technologies provide a solid foundation for clean code. They enable developers to leverage existing solutions, promoting code reuse and reducing the risk of introducing errors.
The selection process requires careful consideration of project requirements, team expertise, and available resources. Choosing tools and libraries that align with these factors contributes significantly to the overall quality of the software.
Significance of Suitable Tools and Libraries
Tools and libraries provide pre-built functionalities, saving development time and effort. This allows developers to focus on higher-level design and logic rather than reinventing the wheel. Libraries also often come with extensive documentation and support communities, which can be invaluable for troubleshooting and learning best practices. Using well-maintained, robust libraries minimizes potential errors and enhances code reliability.
Examples of Tools Enhancing Code Quality
Various tools enhance code quality and maintainability. Linters, for instance, automatically detect potential errors, style inconsistencies, and code smells. Formatters ensure consistent code style across projects, improving readability and reducing maintenance headaches. Code analysis tools can pinpoint areas for improvement and identify potential bugs early in the development cycle.
Advantages of Appropriate Technologies
Choosing appropriate technologies aligns with project needs, ensuring optimal performance and scalability. Employing technologies tailored to specific tasks allows for efficient development and minimizes the risk of performance bottlenecks. The right technologies can enhance security and compliance, reducing vulnerabilities and meeting industry standards.
Choosing Programming Languages Supporting Clean Code Principles
Languages like Python, with its clear syntax and emphasis on readability, support clean code principles. Languages with strong typing systems can help catch errors early, preventing potential bugs from reaching production. Languages with built-in features for testing and debugging can further facilitate clean code development.
Tools Assisting in Refactoring Code
Refactoring tools automate many aspects of code improvement, helping developers restructure existing code without altering its functionality. These tools can simplify complex logic, improve code organization, and eliminate redundancy. Tools like IDEs (Integrated Development Environments) with refactoring capabilities can simplify the code-improvement process.
Table of Popular Tools and Technologies for Clean Code
Tool/Technology | Advantages |
---|---|
Linters (e.g., ESLint, JSHint) | Automated code analysis, identify potential errors, enforce coding style |
Formatters (e.g., Prettier, Black) | Maintain consistent code style, improve readability, reduce visual noise |
Version Control Systems (e.g., Git) | Track changes, facilitate collaboration, manage code history |
Integrated Development Environments (e.g., VS Code, IntelliJ IDEA) | Comprehensive development environment, support for various languages, refactoring tools |
Code Coverage Tools (e.g., JaCoCo, Istanbul) | Measure code coverage, ensure comprehensive test suites, highlight untested areas |
Closing Summary
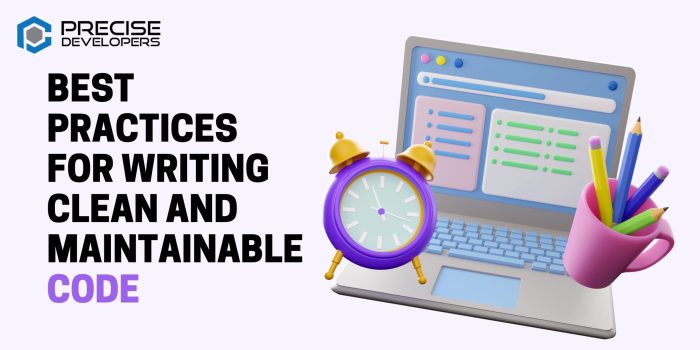
Source: precisedevelopers.com
In conclusion, writing clean, maintainable code is a journey of continuous improvement. By mastering the principles and techniques Artikeld in this guide, developers can significantly enhance the quality and longevity of their code. From understanding fundamental principles to utilizing advanced tools and techniques, the emphasis on readability, maintainability, and testability will contribute to the overall success of any project.
Remember, clean code is not just about following rules; it’s about fostering a culture of collaboration and continuous improvement in your development workflow.
Post Comment