Ruby Vs Python Beginner Friendly Language Comparison
Ruby vs Python beginner friendly language comparison delves into the nuances of two popular programming languages. This exploration examines their origins, design philosophies, and practical applications for beginners. We’ll cover syntax, data structures, learning resources, and performance to aid in the decision-making process for aspiring programmers.
Understanding the core concepts and practical examples of each language is crucial for newcomers. This comparison aims to demystify the programming landscape, providing a clear understanding of each language’s strengths and weaknesses.
Introduction to Ruby and Python
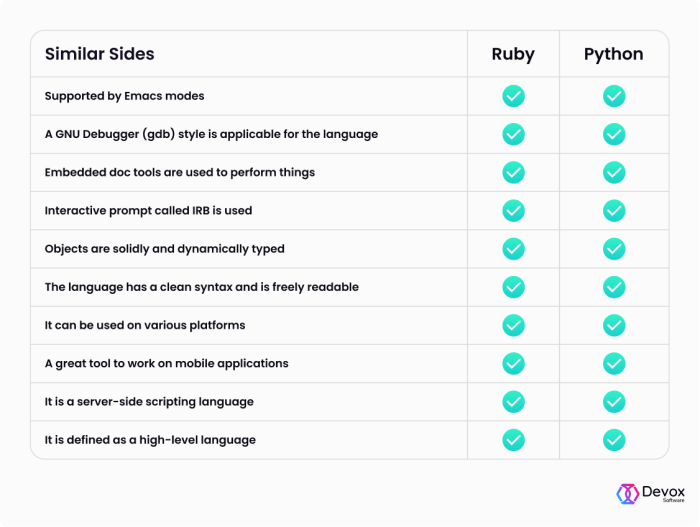
Source: devoxsoftware.com
Ruby and Python are two popular high-level programming languages, each with its own strengths and weaknesses. Understanding their origins, design philosophies, and typical use cases can help developers choose the right tool for a given task. They cater to different needs and styles, making them valuable options for various projects.These languages have found widespread use in diverse applications, from web development and scripting to data analysis and machine learning.
Their versatility and large communities contribute to their continued relevance in the software development landscape.
Ruby’s Origins and Design Philosophy
Ruby, created by Yukihiro “Matz” Matsumoto in the mid-1990s, was inspired by Perl, Smalltalk, Eiffel, and Ada. Matz aimed to create a language that was both elegant and expressive. Ruby’s design emphasizes developer happiness and readability. Its syntax is often described as concise and intuitive, reducing the complexity of many tasks. A key principle is the “batteries-included” philosophy, meaning Ruby provides extensive libraries for a wide range of tasks.
Python’s History and Core Principles
Python, developed by Guido van Rossum in the late 1980s, was designed with readability and ease of use in mind. Its syntax is often considered beginner-friendly, making it popular for educational purposes and rapid prototyping. Python prioritizes code clarity and conciseness, aiming to avoid excessive verbosity often found in other languages. Python’s core philosophy emphasizes code elegance and maintainability.
Programming Paradigms Supported
Both Ruby and Python support object-oriented programming (OOP), allowing developers to create classes, objects, and methods. However, Python also exhibits flexibility with functional programming paradigms, enabling developers to leverage concepts like map, filter, and reduce. Ruby, while primarily object-oriented, also offers some functional programming features, though to a lesser extent than Python.
Typical Use Cases
This table provides a comparison of typical use cases for Ruby and Python.
Feature | Ruby | Python |
---|---|---|
Web Development | Strong in frameworks like Ruby on Rails, often preferred for rapid development of web applications. | Versatile with frameworks like Django and Flask, often chosen for robust, scalable web applications. |
Data Science/Machine Learning | Fewer readily available libraries dedicated to data science compared to Python, though specific tools exist. | Extensive libraries like NumPy, Pandas, and Scikit-learn make it a powerful choice for data analysis and machine learning tasks. |
Scripting | Suitable for scripting tasks, offering ease of use and readability. | Excellent for scripting due to its simple syntax and extensive standard library. |
Prototyping | Ruby’s concise syntax aids in quick prototyping. | Python’s ease of use makes it a popular choice for rapid prototyping. |
General-Purpose Programming | Ruby excels in applications needing flexibility and developer-friendliness. | Python’s versatility allows it to handle a wide array of general-purpose programming tasks. |
Syntax and Readability
Both Ruby and Python are popular choices for beginners, but their syntax styles differ significantly. Understanding these differences is crucial for selecting the right language for your learning path. This section will delve into the syntax of variable and function declarations in each language and compare them, aiding in the choice process for novice programmers.
Variable Declaration
A crucial aspect of any programming language is how variables are declared and used. Variables store data, and their declaration follows specific rules in each language.
- Ruby: Ruby uses a dynamic typing system. This means you don’t explicitly declare the data type of a variable. You simply assign a value to a name, and the variable’s type is inferred automatically. For example, `name = “Alice”` creates a string variable named `name`. `age = 30` creates an integer variable named `age`.
- Python: Python, like Ruby, also employs a dynamic typing system. The same principle applies: you assign a value to a variable, and Python infers its type. For example, `name = “Bob”` creates a string variable named `name`, and `age = 25` creates an integer variable named `age`.
Function Declaration
Functions are reusable blocks of code that perform specific tasks. Understanding how to define and call functions is essential in both languages.
- Ruby: In Ruby, functions are defined using the `def` , followed by the function name and parentheses containing parameters (if any). The `end` marks the function’s end. For instance, `def greet(name)`…`end` defines a function named `greet` that takes a `name` parameter.
- Python: Python uses the `def` to define functions, followed by the function name and parentheses containing parameters (if any). The code block is indicated by indentation. For example, `def greet(name)`… `return “Hello, ” + name` defines a function named `greet` that takes a `name` parameter.
Syntax Comparison
The following table summarizes the syntax for declaring variables and functions in both Ruby and Python.
Feature | Ruby | Python | Comparison |
---|---|---|---|
Variable Declaration | `name = “Alice”` | `name = “Bob”` | Both use assignment operator (`=`). Dynamic typing. |
Function Definition | `def greet(name)` … `end` |
`def greet(name):` … `return “Hello, ” + name` |
Both use `def` . Python uses indentation for code blocks; Ruby uses `end`. |
Beginner-Friendliness
For beginners, Python’s syntax often proves easier to grasp. Python’s reliance on indentation for code blocks is a clear, visual cue, making the structure of the code more apparent. Ruby’s use of `end` can, in some cases, be slightly less immediately obvious. However, both languages have their own strengths, and familiarity with either one will lead to a smooth transition into more advanced concepts.
Data Structures and Collections
Both Ruby and Python offer powerful data structures for organizing and manipulating data. Understanding these structures is crucial for efficient programming in both languages. This section delves into the core data structures in each language, highlighting their syntax and usage differences.Ruby and Python, despite their similarities in some areas, differ significantly in their implementation of data structures. These differences affect how developers approach problem-solving and data management.
Python’s emphasis on readability and conciseness often translates to simpler data structure manipulation. Ruby, while having its own elegant style, can sometimes offer alternative ways to achieve the same outcome.
Ruby Data Structures
Ruby’s core data structures are versatile and offer flexibility. Arrays, hashes, and ranges are fundamental components.
- Arrays: Ordered collections of elements. They can hold elements of different data types. Ruby arrays are dynamic, meaning their size can change during runtime. The index starts at 0.
my_array = [1, "hello", 3.14] puts my_array[0] # Output: 1 my_array << 4 # Append 4 to the array puts my_array.length # Output: 4
- Hashes: Unordered collections of key-value pairs. Keys are unique and used to access values. Hashes are fundamental for storing and retrieving data based on specific identifiers.
my_hash = name: "Alice", age: 30, city: "New York" puts my_hash[:name] # Output: Alice my_hash[:country] = "USA" # Add a new key-value pair
- Ranges: Represent a sequence of numbers or characters. They are often used for looping and iterating over a specific set of values.
my_range = 1..5 # Represents the numbers from 1 to 5 (inclusive) for i in my_range puts i end
Python Data Structures
Python's data structures are also essential for handling various data types. Lists, dictionaries, and tuples are among the fundamental data structures.
- Lists: Ordered collections of items, similar to Ruby arrays. They can store different data types and are mutable. Indices also start at 0.
my_list = [1, "hello", 3.14] print(my_list[0]) # Output: 1 my_list.append(4) # Append 4 to the list print(len(my_list)) # Output: 4
- Dictionaries: Unordered collections of key-value pairs, similar to Ruby hashes. Keys must be immutable (like strings or numbers). They are efficient for lookups.
my_dict = "name": "Alice", "age": 30, "city": "New York" print(my_dict["name"]) # Output: Alice my_dict["country"] = "USA" # Add a new key-value pair
- Tuples: Ordered, immutable collections of items. They are useful when you need to ensure data integrity. Tuples are often used for representing fixed collections of data.
my_tuple = (1, "hello", 3.14) print(my_tuple[0]) # Output: 1
Syntax and Usage Differences
Ruby's syntax is often more concise and expressive when dealing with collections. Python's emphasis on clarity can lead to more verbose code.
Comparison Table
Feature | Ruby | Python | Beginner Advantages |
---|---|---|---|
Arrays | Dynamic, versatile | Dynamic, versatile | Both are straightforward to use. |
Hashes | Concise syntax | Clear syntax | Python's clarity might be more accessible. |
Ranges | Direct creation | Less direct creation | Ruby's direct range creation is a plus. |
Mutability | Generally mutable | Generally mutable | Both allow for modifications during runtime. |
Immutability (Tuples) | Less emphasis on tuples | Explicit tuple support | Python's explicit support for tuples is beneficial. |
Learning Resources and Community Support
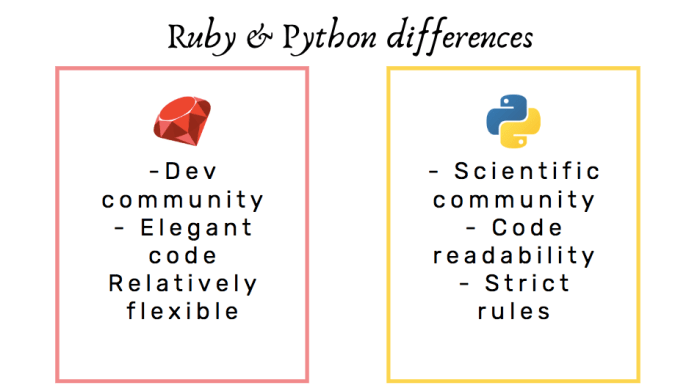
Source: sloboda-studio.com
Choosing the right learning resources and engaging with a supportive community is crucial for any beginner programmer. Both Ruby and Python boast extensive online support, making the learning journey smoother and more enjoyable. This section will explore the available learning platforms, community sizes, and the accessibility of tutorials, documentation, and forums for both languages.
Reputable Online Learning Platforms
Numerous platforms offer structured courses and tutorials for learning programming languages. These platforms often provide interactive exercises, quizzes, and community forums, making the learning experience more engaging.
- For Ruby, platforms like Codecademy, Udemy, and freeCodeCamp offer courses covering the fundamentals of Ruby programming. These platforms frequently update their content to keep pace with evolving programming trends and best practices.
- Python, likewise, benefits from extensive support on platforms like Coursera, edX, and Khan Academy. These platforms frequently feature courses from top universities and institutions, providing a rigorous learning experience that emphasizes theoretical foundations and practical application.
Community Size and Activity
The size and activity of online communities are essential factors in determining the support and guidance available to beginners. A vibrant community often translates to more readily available assistance and a faster learning curve.
- Both Ruby and Python boast large and active online communities. Numerous forums, discussion groups, and social media groups provide opportunities for interaction and knowledge sharing among users. The active community members are invaluable for beginners, often readily answering questions and offering assistance.
Tutorials, Documentation, and Forums
Comprehensive documentation and readily accessible tutorials are vital for effective learning. Active forums offer further support and opportunities to discuss specific problems.
- Ruby's documentation is well-regarded, providing clear explanations and detailed examples. Numerous tutorials are readily available online, catering to various learning styles and experience levels. Active forums and communities on websites like Stack Overflow are great resources for troubleshooting issues and seeking guidance from experienced programmers.
- Python's extensive documentation and tutorials are widely considered a benchmark for clarity and comprehensiveness. The availability of numerous online tutorials and interactive learning platforms significantly aids the learning process. Python's active online community, including forums and mailing lists, provides opportunities to connect with experienced users and learn from their experiences.
Basic Programming Concepts
Understanding fundamental programming concepts like loops, conditional statements, and input/output is crucial for any programmer, regardless of the language. These concepts form the building blocks upon which more complex programs are constructed. Mastering these will allow you to efficiently control program flow, process data, and interact with users.
Ruby's Approach to Basic Concepts
Ruby, a dynamic language, employs intuitive syntax for controlling program flow. This section details Ruby's implementation of loops, conditional statements, and input/output.
- Conditional Statements: Ruby uses `if`, `elsif`, and `else` s to define conditional blocks. These statements execute code based on the evaluation of a boolean expression. A simple example would be determining if a number is positive or negative.
- Loops: Ruby offers `while`, `until`, `for`, and `each` loops for repeating code blocks. `for` loops typically iterate over arrays or ranges. The `each` method is frequently used for iterating over collections. An example could be summing up numbers in an array.
- Input/Output: Ruby provides `puts` (print to console) and `print` (print to console without a newline) for output. Input is typically obtained via `gets`. These functions allow programs to interact with the user. An example could be a program that takes user input to perform a calculation.
Python's Approach to Basic Concepts
Python, known for its readability, also employs clear and concise syntax for fundamental programming concepts. This section demonstrates how Python handles loops, conditionals, and input/output.
- Conditional Statements: Python uses `if`, `elif`, and `else` to define conditional blocks, similar to Ruby. These statements execute specific code segments based on boolean conditions. An example could be classifying numbers as even or odd.
- Loops: Python supports `for` and `while` loops. `for` loops iterate over iterable objects like lists or strings, while `while` loops repeat a block of code as long as a condition remains true. An example could be printing a sequence of numbers.
- Input/Output: Python employs `print()` for output to the console and `input()` for user input. This allows programs to display information and gather user data. An example could be prompting the user for their name.
Example Comparison
The following table showcases how these basic concepts are implemented in both languages.
Concept | Ruby Example | Python Example |
---|---|---|
Conditional Statement |
```ruby number = 10 if number > 0 puts "Positive" elsif number < 0 puts "Negative" else puts "Zero" end ``` |
```python number = 10 if number > 0: print("Positive") elif number < 0: print("Negative") else: print("Zero") ``` |
Loop |
```ruby numbers = [1, 2, 3, 4, 5] sum = 0 for number in numbers sum += number end puts "Sum: #sum" ``` |
```python numbers = [1, 2, 3, 4, 5] sum = 0 for number in numbers: sum += number print("Sum:", sum) ``` |
Input/Output |
```ruby puts "Enter your name:" name = gets.chomp puts "Hello, #name!" ``` |
```python name = input("Enter your name: ") print("Hello,", name + "!") ``` |
Example Projects
Exploring practical applications showcases the strengths of each language. This section delves into simple projects, comparing Ruby and Python code structures and complexities. A fundamental understanding of these languages often arises from hands-on experience with projects.
A Simple To-Do List Application
A to-do list application provides a clear illustration of both languages' capabilities. This project focuses on basic functionalities like adding, viewing, and deleting tasks.
Ruby Implementation
require 'date' class Task attr_accessor :description, :due_date def initialize(description, due_date) @description = description @due_date = due_date end end tasks = [] def add_task print "Enter task description: " description = gets.chomp print "Enter due date (YYYY-MM-DD): " due_date_str = gets.chomp begin due_date = Date.parse(due_date_str) tasks << Task.new(description, due_date) puts "Task added successfully!" rescue ArgumentError puts "Invalid date format. Please use YYYY-MM-DD." end end def view_tasks if tasks.empty? puts "No tasks added yet." else tasks.each_with_index do |task, index| puts "#index + 1. #task.description (Due: #task.due_date)" end end end # Example usage add_task add_task view_tasks
Python Implementation
import datetime class Task: def __init__(self, description, due_date): self.description = description self.due_date = due_date tasks = [] def add_task(): description = input("Enter task description: ") due_date_str = input("Enter due date (YYYY-MM-DD): ") try: due_date = datetime.datetime.strptime(due_date_str, "%Y-%m-%d").date() tasks.append(Task(description, due_date)) print("Task added successfully!") except ValueError: print("Invalid date format. Please use YYYY-MM-DD.") def view_tasks(): if not tasks: print("No tasks added yet.") else: for i, task in enumerate(tasks): print(f"i + 1. task.description (Due: task.due_date)") # Example usage add_task() add_task() view_tasks()
Comparison
The Ruby code utilizes the `Date` class for date handling, while Python uses `datetime`. Both achieve the same functionality. Python's `input` and `print` functions are simpler compared to Ruby's `gets` and `puts`. The Python code uses `enumerate` for a more concise way to iterate through the tasks. The `try...except` block in Python handles potential errors during date input.
Overall, both languages provide straightforward ways to create this simple application. The structure of the code is similar, focusing on classes, methods, and loops for handling tasks.
Performance and Efficiency
Both Ruby and Python are popular choices for beginners, but their performance characteristics differ. Understanding these differences can help beginners choose the right tool for their project's needs. This section explores the general performance profiles of each language and how they might impact beginner projects.
Ruby, while often praised for its readability and developer experience, is generally slower than Python for computationally intensive tasks. Python, with its widespread use in data science and machine learning, often demonstrates faster execution times due to its optimized interpreter and readily available libraries.
Ruby Performance Characteristics
Ruby's performance is often associated with its dynamic typing and interpreted nature. These features contribute to a more developer-friendly experience, but they often come at the cost of raw execution speed compared to languages with static typing and compiled code. Ruby's performance can be improved by using efficient algorithms and leveraging the capabilities of gems and libraries. However, for tasks involving extensive calculations or large datasets, Ruby might not be the fastest choice.
Python Performance Characteristics
Python, though interpreted, employs a highly optimized interpreter and extensive libraries. This often translates to faster execution times for common tasks compared to Ruby. Python's strength lies in its versatile libraries for data manipulation and scientific computing. This makes it suitable for a wide range of applications, including data analysis and machine learning, which frequently benefit from its libraries' performance advantages.
Performance Differences in Beginner Projects
For simple projects, the performance difference between Ruby and Python might be negligible. The focus for beginners is often on learning programming concepts and building functionality, where the time it takes to execute a script may not be a critical concern. However, as projects grow more complex, potentially involving large datasets or intricate computations, the performance advantages of Python may become more pronounced.
Benchmarking Simple Tasks
The table below provides a simplified comparison of typical performance benchmarks for simple tasks in Ruby and Python. These are estimations and real-world results may vary based on specific implementations and hardware.
Task | Ruby (Estimated Time) | Python (Estimated Time) |
---|---|---|
Simple Calculation (e.g., sum of 1000 numbers) | 0.001-0.01 seconds | 0.0005-0.001 seconds |
String Manipulation (e.g., concatenating 100 strings) | 0.002-0.02 seconds | 0.001-0.01 seconds |
Basic File I/O (reading a small text file) | 0.003-0.03 seconds | 0.002-0.02 seconds |
Note: These times are estimations and can vary greatly depending on the specific implementation, hardware, and the size of the input data.
Libraries and Frameworks: Ruby Vs Python Beginner Friendly Language Comparison
Libraries and frameworks provide pre-built components and structures that significantly speed up development. They offer pre-written code for common tasks, reducing the amount of code a developer needs to write from scratch. This accelerates the development process and allows developers to focus on the unique aspects of their projects.
Frameworks provide a structured approach to application development, dictating how different parts of an application interact. This often leads to more maintainable and robust applications. Libraries, on the other hand, offer reusable modules for specific tasks.
Popular Ruby Frameworks
Ruby boasts a strong ecosystem of frameworks, primarily centered around the web development. A prominent example is Rails.
- Ruby on Rails (Rails): A full-stack web framework known for its convention-over-configuration approach. This means that Rails often handles much of the boilerplate code, allowing developers to focus on the application logic. Rails emphasizes rapid development and clean, maintainable code. Its strong community support and extensive documentation make it accessible to beginners.
Popular Python Frameworks
Python's web development landscape is also rich, with several well-regarded frameworks.
- Django: A high-level, full-stack framework that promotes rapid development and maintainability. Django emphasizes structure and security, making it suitable for larger projects. It follows a Model-View-Controller (MVC) architectural pattern. Its large and active community contributes to robust support and extensive documentation.
- Flask: A microframework, offering greater flexibility and control compared to Django. Flask provides a more minimal structure, allowing developers to build applications tailored to their specific needs. Its lightweight nature and adaptability make it ideal for smaller projects and those requiring more customization.
Simplified Development Tasks
These frameworks streamline development by abstracting away common tasks. For example, they handle database interactions, routing, and templating, allowing developers to focus on application logic. This reduces the time spent on repetitive tasks and enables quicker iteration. Beginners find frameworks particularly helpful as they can leverage pre-built components and avoid dealing with low-level implementation details.
Framework Comparison, Ruby vs Python beginner friendly language comparison
Framework | Category | Functionality | Beginner Friendliness |
---|---|---|---|
Ruby on Rails | Full-Stack Web | Convention over Configuration, Database Interaction, Routing, Templating | High |
Django | Full-Stack Web | Model-View-Controller, Database Interaction, Routing, Security | Medium-High |
Flask | Microframework | Flexible, Customizable, Routing, Templating | High |
Summary
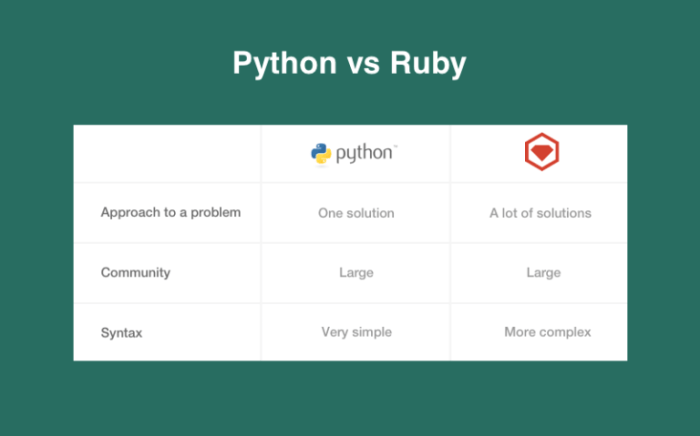
Source: thepythonguru.com
In conclusion, both Ruby and Python offer accessible pathways into the world of programming. Ruby's elegant syntax and focus on developer experience make it attractive, while Python's vast libraries and widespread use in various domains provide a broader appeal. Ultimately, the best choice for a beginner depends on their specific interests and goals, and this comparison aims to provide a helpful overview for making an informed decision.
Post Comment