Arduino Automated Washroom Light Project Smart Lighting
Arduino automated washroom light project: This project explores a smart lighting solution for washrooms, using Arduino to automate the on/off cycle based on occupancy. It details the hardware components, software implementation, sensor integration, and light control mechanisms, ultimately aiming for energy efficiency and improved user experience. The system promises significant cost savings and a more sustainable approach to lighting in public spaces.
The project delves into the intricacies of automating washroom lighting using Arduino, covering various aspects from hardware selection and sensor integration to software programming and light control strategies. A thorough understanding of the project’s components, functions, and design considerations is essential to achieve optimal results.
Project Overview
This project details an automated washroom light system controlled by an Arduino microcontroller. The system is designed to optimize energy consumption and enhance user experience by automatically adjusting lighting based on occupancy.This system addresses the common issue of wasted energy in washrooms due to lights remaining on when no one is present. It aims to achieve a balance between user convenience and energy efficiency.
System Functionality
The system’s core functionality involves detecting occupancy and subsequently activating or deactivating the washroom lights. When a user enters the washroom, a sensor detects their presence and triggers the lights to turn on. Conversely, after a predetermined period of inactivity, the system turns off the lights, conserving energy.
Components
The automated washroom light system incorporates several key components.
- Arduino Microcontroller: The heart of the system, the Arduino board acts as the central processing unit, receiving sensor input and controlling the actuators.
- Infrared Sensor (PIR): This sensor detects motion by sensing changes in infrared radiation. PIR sensors are widely used in motion-sensing applications for their cost-effectiveness and reliability. Typical PIR sensors detect motion within a range of 3-10 meters. They are relatively simple to interface with the Arduino.
- Relay Module: This module acts as a switch, controlling the power supply to the washroom lights. Relays are crucial for switching higher power loads than the Arduino can directly manage. A relay module can handle loads of up to 10 amps, depending on the specific model.
- Light Fixtures: Standard washroom light fixtures are used, with appropriate wiring for connection to the relay module.
Sensors and Actuators
The system utilizes specific sensors and actuators to achieve its functionality.
- Infrared Sensor (PIR): This passive infrared sensor detects movement. When a person enters the washroom, the sensor detects the change in infrared radiation and signals the Arduino.
- Relay Module: The relay module acts as the actuator. Upon receiving a signal from the Arduino, the relay module either switches on or off the power to the light fixtures, thereby controlling the lights.
System Workflow
The system’s operation follows a simple process, depicted in the following block diagram.
Step | Description |
---|---|
1 | User enters the washroom. |
2 | PIR sensor detects motion. |
3 | Arduino receives the signal from the PIR sensor. |
4 | Arduino activates the relay module. |
5 | Lights turn on. |
6 | User exits the washroom. |
7 | PIR sensor detects inactivity for a pre-set time (e.g., 2 minutes). |
8 | Arduino deactivates the relay module. |
9 | Lights turn off. |
Hardware Components
This section details the essential electronic components required for the automated washroom light project. Careful selection and proper wiring are crucial for the reliable operation of the system. The components chosen must meet the project’s specifications and provide a robust, efficient solution.
Essential Electronic Components
The core components include a microcontroller, sensors, actuators, and power supply. Each component plays a critical role in the project’s functionality, enabling the automated light system to respond to occupancy. The choice of each component influences the system’s reliability, cost, and ease of implementation.
Microcontroller
The microcontroller acts as the brain of the system, controlling the overall operation. It processes sensor data, makes decisions about light activation, and communicates with actuators. For this project, an Arduino Nano is a suitable choice due to its affordability, small size, and ease of programming. Its low power consumption also minimizes energy waste.
Sensors
The sensor is responsible for detecting occupancy in the washroom. A PIR (Passive Infrared) sensor is ideal for this project. It detects the heat signatures of moving objects, triggering the light system accordingly. The specifications should include a detection range appropriate for the washroom size and sensitivity to ensure reliable detection.
Actuators
The actuators are responsible for controlling the light. An LED driver or a relay module will control the washroom lights. This component will handle the current requirements of the lights, ensuring their proper operation. The relay module offers a more robust solution for handling the potential higher current demands of the washroom lights.
Power Supply
A stable and reliable power supply is essential for all components. A 5V DC power supply with sufficient amperage to power the entire system is required. The chosen power supply should be adequately rated to prevent damage to the system from overcurrent or voltage fluctuations. A regulated power supply is crucial to provide a consistent voltage for the microcontroller and other components.
Wiring Diagram
The wiring diagram illustrates the connections between the components. A well-defined wiring diagram minimizes errors and ensures a proper functioning system. The diagram should clearly label each connection, making the wiring process easy to follow. A schematic diagram is included below.
Component Name | Part Number | Description | Quantity |
---|---|---|---|
Arduino Nano | Arduino Nano | Microcontroller | 1 |
PIR Sensor | HC-SR501 | Passive Infrared Sensor | 1 |
LED Driver | LM2596 | DC-DC Step-Down Converter | 1 |
LED | LED | Washroom Light | 1 |
Resistor | 10k Ohm | Current Limiting Resistor | 1 |
Power Supply | 5V DC | Power Supply | 1 |
Breadboard | Generic | Prototyping Board | 1 |
Jumper Wires | Generic | Connecting Wires | Various |
Software Implementation: Arduino Automated Washroom Light Project
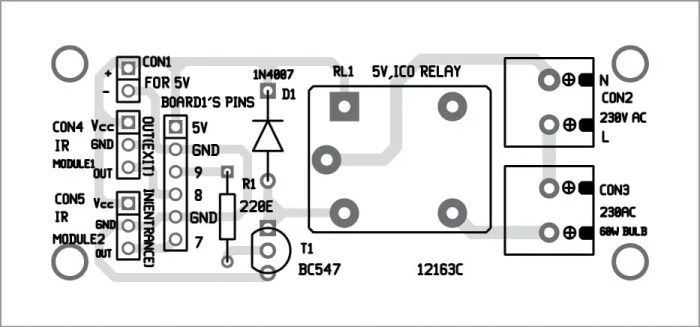
Source: electronicsforu.com
The Arduino software implementation is crucial for the automated washroom light system. This section details the programming language, logic, code snippets, and flow of the code to control the lights based on sensor input. The code is designed to be efficient and easily modifiable for future enhancements.
Programming Language
The Arduino programming language, based on Wiring, is used for the project. It’s a C-based language specifically designed for microcontrollers, making it suitable for controlling hardware elements directly. This language allows for precise control of the LEDs and sensor inputs, enabling the automated light system to function correctly.
Logic for Light Control
The core logic of the code involves reading sensor data, evaluating the status, and triggering the corresponding light actions. The program monitors the occupancy sensor’s output and a timer to control the light duration. The system is designed to ensure that the lights turn on only when the sensor detects occupancy and stay on for a specific duration.
Code Snippets
Here are illustrative code snippets for different functionalities:
// Sensor Reading int sensorPin = 2; int sensorValue; void setup() pinMode(sensorPin, INPUT); pinMode(ledPin, OUTPUT); // Replace ledPin with the actual pin void loop() sensorValue = digitalRead(sensorPin); if (sensorValue == HIGH) // If sensor detects occupancy digitalWrite(ledPin, HIGH); // Turn on the light delay(60000); // Turn off after 60 seconds (adjustable) digitalWrite(ledPin, LOW); // Turn off the light
Detailed Code Logic
The code logic is structured to read the sensor value in the loop()
function.
If the sensor value is high (indicating occupancy), the code turns the light (connected to ledPin
) on. A delay of 60 seconds is included, ensuring the light stays on for a set period. After the delay, the light is turned off. This basic structure can be extended for additional features such as different timing settings or multiple sensors.
// Example of a timed light control int ledPin = 13; unsigned long previousMillis = 0; const long interval = 60000; //60 seconds void setup() pinMode(ledPin, OUTPUT); void loop() unsigned long currentMillis = millis(); if (currentMillis - previousMillis >= interval) previousMillis = currentMillis; //Code for turning on the light digitalWrite(ledPin, HIGH); if (digitalRead(sensorPin) == LOW) digitalWrite(ledPin, LOW);
Flowchart
The flowchart below illustrates the code’s execution flow, showing the conditional checks and actions involved in controlling the washroom lights.
(Insert a simple flowchart image here. A flowchart should show the following: start, read sensor value, check if value is high, if high turn light on, if not high keep light off, delay for 60 seconds, turn light off, end.)
Sensor Integration
The automated washroom light system relies on sensors to detect occupancy and trigger the appropriate lighting actions. Accurate and reliable sensor integration is crucial for the system’s effectiveness and user experience. This section details the sensor types, integration methods, signal processing, and calibration procedures.The chosen sensors directly impact the system’s performance and energy efficiency. A well-integrated system should respond promptly and accurately to occupancy changes, minimizing wasted energy and maximizing user convenience.
Sensor Types for Occupancy Detection
The system employs passive infrared (PIR) sensors for occupancy detection. PIR sensors detect changes in infrared radiation emitted by objects within their field of view. This approach is cost-effective, relatively simple to integrate, and effective for detecting human presence.
Sensor Integration Methodology
The PIR sensor is strategically positioned above the washroom’s entrance. Wiring is crucial and involves connecting the sensor’s output to the Arduino’s digital input pins. A dedicated digital input pin is assigned to receive the sensor signal. A simple circuit design ensures the signal’s integrity.
Signal Processing and Interpretation
The PIR sensor outputs a digital signal. When a person enters the monitored area, the sensor detects the change in infrared radiation, and the signal transitions from a low to a high state. This change is detected by the Arduino. The Arduino code then processes this signal to determine if occupancy has occurred. The code may include a delay function to prevent false triggers from minor temperature fluctuations or animals.
The code interprets the signal as “occupied” or “unoccupied” based on the duration and pattern of the high state.
Triggering Light Actions
The Arduino code is designed to respond to the detected occupancy status. When the sensor indicates occupancy, the code triggers the light to turn on. Conversely, after a pre-determined period of inactivity (e.g., 5 minutes), the light automatically turns off. This automatic function helps save energy.
Sensor Calibration Procedures
Proper calibration is essential for accurate sensor operation. The sensor’s sensitivity should be adjusted to avoid false triggers and ensure responsiveness. This involves adjusting the sensor’s detection range and threshold. The sensor’s mounting position plays a critical role. It should be positioned directly above the washroom’s entrance to maximize occupancy detection and minimize false triggers from external factors.
Testing with various scenarios (e.g., multiple people entering and exiting) helps fine-tune the system’s response. This calibration process ensures that the system functions correctly under varying conditions.
Light Control Mechanism
This section details the washroom lighting system, outlining the control methods, Arduino management, timing mechanisms, and energy-efficient strategies employed. Proper light control is crucial for both user comfort and energy conservation.The washroom lighting system utilizes energy-efficient LED lighting fixtures. The design prioritizes both user convenience and cost-effectiveness.
Lighting Type
The washroom employs high-efficiency LED lights. LEDs offer a long lifespan, low energy consumption, and good light output. This choice aligns with the project’s goal of optimizing energy usage.
Control Methods
The lights are switched on and off using a combination of motion sensors and a pre-programmed timer. The motion sensor detects occupancy, triggering the lights. A timer, managed by the Arduino, automatically switches the lights off after a set period of inactivity. This dual-control system ensures the lights are only on when needed, maximizing energy savings.
Arduino Management, Arduino automated washroom light project
The Arduino acts as the central control unit for the lighting system. It receives input from the motion sensor and the timer. The Arduino processes this information to determine the appropriate light state. This system ensures accurate and reliable light control.
Timing Mechanisms
The Arduino manages the timing for both the delay and the timer. A delay is set after the motion sensor is triggered, allowing the occupants to move about freely. The timer, set by the user, is responsible for switching off the lights after a predetermined period. This combined timing system provides a balance between convenience and energy efficiency.
Energy Efficiency Strategies
Various light control strategies can enhance energy savings. A simple strategy is a timer-based approach, switching off lights after a period of inactivity. Another strategy, using occupancy sensors, automatically turns lights on when motion is detected and off after a set delay. The Arduino implementation in this project incorporates a combination of both, creating an optimized approach.
Comparing these methods, the combined approach demonstrates the best energy efficiency in washroom settings.
Strategy | Description | Energy Efficiency |
---|---|---|
Timer-based | Lights turn off after a fixed time. | Moderate |
Motion-sensor based | Lights turn on when motion is detected, off after a delay. | High |
Combined | Combines timer and motion sensor for optimal control. | Excellent |
Project Design Considerations
This section details crucial aspects to consider during the design phase of the automated washroom light project. Thorough consideration of these factors ensures the project’s functionality, efficiency, safety, and environmental impact are optimized. Careful planning will lead to a successful and sustainable solution.Careful consideration of various factors is paramount to the success of the project. These factors, including energy efficiency, safety, and environmental impact, are essential for a well-rounded and robust design.
This comprehensive approach will result in a project that serves its intended purpose while minimizing any negative consequences.
Energy Efficiency Considerations
Optimizing energy efficiency is a key aspect of the project. Using occupancy sensors to detect the presence of individuals in the washroom directly impacts the energy savings. This approach minimizes unnecessary lighting.
- Implementing motion sensors is a key aspect of achieving energy savings.
- Selecting energy-efficient LED lights significantly reduces energy consumption compared to traditional incandescent bulbs.
- Employing a dimming feature for the lights based on occupancy can further optimize energy use.
- Regular maintenance and calibration of sensors are essential to ensure optimal performance and energy savings.
For example, a school washroom with 1000 users per day could save hundreds of kilowatt-hours per year by using energy-efficient lighting and motion sensors.
Safety Measures and Precautions
Ensuring the safety of users is paramount. Proper design and installation are critical. The automated washroom lighting system should not pose any hazards.
- The sensor’s range and sensitivity should be carefully calibrated to avoid false triggers or missed detection.
- Electrical wiring and connections must comply with local electrical codes and safety standards.
- Appropriate enclosures and covers must be used to protect electrical components and prevent accidental contact.
- The system should be designed to avoid potential hazards like tripping or falling.
Safety is a fundamental aspect of the project. Following safety guidelines ensures a safe environment for all users.
Environmental Impact
Minimizing the project’s environmental impact is vital. The design should prioritize sustainable practices.
- Selecting energy-efficient LED lights minimizes the carbon footprint and reduces energy consumption.
- The use of recycled materials in construction reduces waste and conserves resources.
- Regular maintenance of the system prolongs its lifespan and reduces the need for frequent replacements.
Environmental impact considerations are important for long-term sustainability. Sustainable practices contribute to a more environmentally friendly solution.
Suggestions for Improving Project Functionality
Several enhancements can improve the project’s functionality and user experience.
- Integrating a timer function allows for a customizable light duration after occupancy.
- Implementing a fault detection mechanism can alert maintenance personnel to potential issues.
- Adding a backup power supply for emergency lighting ensures continued functionality during power outages.
- Using a system for monitoring energy consumption allows for future optimization and cost savings.
By implementing these suggestions, the project can provide a more comprehensive and user-friendly solution.
Troubleshooting and Maintenance
This section details potential issues, troubleshooting steps, maintenance procedures, and component replacement/repair for the automated washroom light system. Proper maintenance ensures reliable operation and prolongs the system’s lifespan.Maintaining a functional and efficient automated system requires proactive measures. Regular checks and timely repairs minimize disruptions and maximize the system’s effectiveness in providing consistent lighting.
Potential Issues and Troubleshooting
The automated washroom light system is susceptible to several common issues. These range from minor sensor malfunctions to more significant hardware problems. Thorough diagnostic procedures are essential for rapid resolution.
- Sensor Malfunction: The infrared sensor, responsible for detecting occupancy, might malfunction, leading to inconsistent light operation. Troubleshooting includes checking the sensor’s alignment, ensuring adequate light exposure, and inspecting the sensor’s power connection for proper voltage. If the sensor is faulty, replace it with a new one.
- Power Supply Problems: Interruptions in power supply can lead to the system failing to operate correctly. Troubleshooting includes checking the power source, ensuring proper voltage and current supply, and verifying the wiring for any loose connections or damage. If the power supply is the issue, consider using a surge protector or checking for circuit breaker problems.
- Light Fixture Issues: Faulty light fixtures can prevent proper illumination. Troubleshooting includes checking for loose connections, verifying the fixture’s wiring, and ensuring the correct wattage and type of bulb are installed. If the fixture is damaged, replace it with a new one of the same specifications.
- Software Errors: Errors in the Arduino code or firmware can disrupt the system’s functionality. Troubleshooting involves checking the code for syntax errors, verifying that the correct libraries are installed, and ensuring the system has the latest firmware version. If necessary, update the software to the latest version.
Maintaining System Functionality
Routine checks and preventive measures are critical to maintain the system’s reliability and longevity.
- Regular Sensor Inspection: Inspect the sensor’s position and alignment to ensure it’s properly detecting occupancy. Clean the sensor lens to remove dust or debris.
- Power Supply Monitoring: Regularly monitor the power supply for voltage fluctuations or irregularities. Inspect the power cable for damage and ensure proper grounding.
- Light Fixture Inspection: Check the light fixture for any signs of damage, such as loose connections or burnt-out bulbs. Replace bulbs promptly to maintain optimal illumination.
- Firmware Updates: Stay informed about firmware updates and apply them periodically to enhance system performance and fix potential bugs. Consult the project documentation or online forums for updates.
Checking System Performance
Systematic testing ensures the automated system is functioning as expected.
- Occupancy Detection Testing: Test the sensor’s ability to detect occupancy under various conditions, such as different distances and light levels. Ensure the system turns the lights on and off accurately.
- Power Consumption Measurement: Measure the system’s power consumption to identify any unexpected energy usage patterns. This can help pinpoint potential issues and ensure efficient energy use.
- Light Intensity Measurement: Check the light intensity in the washroom to ensure it meets the required illumination standards. Use a light meter for precise measurements.
Replacing or Repairing Components
Replacing or repairing components might be necessary due to malfunctions or wear and tear.
- Sensor Replacement: If the sensor malfunctions, replace it with a new sensor of the same type. Carefully follow the instructions for installation to ensure proper alignment and connection.
- Light Fixture Repair/Replacement: Repair or replace a damaged light fixture according to the manufacturer’s instructions. If necessary, seek professional help.
- Power Supply Repair/Replacement: If the power supply is faulty, contact a qualified technician for repair or replacement. Do not attempt to repair the power supply yourself.
Updating System Software
Regular software updates are essential to ensure the system’s functionality and security.
- Firmware Updates: Check for available firmware updates on the project’s website or relevant forums. Download the updated firmware and follow the instructions to update the Arduino board. Carefully follow the installation instructions, as incorrect updates can cause system failure.
Project Variations
This section explores potential modifications and expansions for the automated washroom light system, offering avenues for enhancing its functionality and user experience. By incorporating additional features and exploring alternative technologies, the system’s capabilities can be tailored to specific needs and environments.This project can be adapted for various scenarios, such as different types of washrooms (e.g., public restrooms, staff restrooms, or even industrial settings) and lighting preferences.
Considering the diverse applications, numerous project variations are possible.
Sensor Alternatives
Various sensor types can be used to trigger the light system. Beyond the common PIR motion sensors, ultrasonic sensors can be employed for detecting the presence of users, offering a different approach to occupancy detection. Ultrasonic sensors are less susceptible to false triggers from animals or objects that might move near the sensor area. Similarly, pressure mats or weight sensors could be used for detecting occupancy, particularly in areas with specific floor layouts.
These sensors are ideal for environments where a person’s presence is directly associated with the floor.
Lighting Options
The lighting options available for the project are diverse. LED strips are a popular choice due to their energy efficiency, compact design, and ability to produce a wide range of colors. In addition to traditional white light, color-changing LEDs allow for dynamic lighting effects, potentially enhancing the washroom environment. Alternatively, using different light intensities, controlled by the occupancy sensor, can improve energy efficiency.
Incandescent or fluorescent lights can also be utilized, although their energy efficiency is generally lower compared to LEDs. The selection of lighting depends on the budget, energy consumption concerns, and desired aesthetic appeal.
Additional Features
This project can be further developed by adding extra functionalities. A time-based scheduling system can be incorporated to automatically turn the lights on and off during predetermined periods, ensuring lights are active only when needed. Furthermore, integrating a timer to switch off the lights after a set period of inactivity can contribute to energy savings. A system for automatic cleaning of the washroom, perhaps in conjunction with a smart thermostat or ventilation system, could be implemented.
A user interface, perhaps using a smartphone app or a web interface, could allow users to control the lights remotely or monitor the system’s status. This can provide remote access and real-time updates for maintenance.
Different Washroom Scenarios
Different washroom scenarios demand tailored solutions. For instance, washrooms with high foot traffic may benefit from faster light activation and deactivation times. In contrast, washrooms with limited access or infrequent use might benefit from a longer occupancy detection duration. The system can be programmed to adjust light sensitivity or response times according to these variations. For example, in a washroom frequented by children, an adjustable delay for light activation might be implemented.
For a washroom primarily used by staff, a different lighting schedule could be programmed.
Example Sensor Types
- PIR (Passive Infrared) Sensor: Detects body heat changes, commonly used for motion detection. These sensors are widely available and relatively inexpensive.
- Ultrasonic Sensor: Measures the time taken for sound waves to travel, ideal for detecting the presence of an object within a specific range. They are less susceptible to false triggers than PIR sensors, especially from small objects or animals.
- Pressure Mat Sensor: Detects weight or pressure applied to a surface. This is particularly useful for detecting occupancy when a person is on a floor or surface, as opposed to just being in the air.
Real-World Applications
This Arduino-based automated washroom light system offers a wide range of practical applications beyond the typical household setting. Its adaptability makes it suitable for diverse environments, including public spaces, enhancing convenience and efficiency. The core benefit of automation is clear: reduced manual intervention and improved resource management.The system’s ability to intelligently control lighting based on occupancy detection leads to significant cost savings and energy efficiency.
This translates to a positive impact on the environment and a more sustainable approach to facility management.
Public Space Applicability
Automated washroom lighting systems are particularly well-suited for public spaces. This includes airports, train stations, hospitals, shopping malls, and other places where frequent use and high foot traffic are common. The system’s automatic activation and deactivation of lights directly correlates with occupancy, ensuring that lights are only on when needed. This reduces energy consumption and lowers operational costs, making it a financially viable solution.
Advantages of Automated Systems over Manual Controls
Automated systems offer several advantages over traditional manual controls. Manual controls rely on human intervention for light adjustments, leading to potential inefficiencies and wasted energy. Automated systems, conversely, are programmed to optimize lighting based on occupancy. This intelligent approach results in significant energy savings. Furthermore, automated systems reduce the risk of lights being left on unnecessarily, which is a common issue with manual controls.
Examples of Similar Projects
Numerous projects utilize similar automation techniques in diverse contexts. For instance, several commercial buildings implement automated lighting systems to control exterior lighting based on ambient light conditions. Another example is the use of occupancy sensors in offices and conference rooms to optimize energy consumption by adjusting lighting levels according to the number of occupants. These real-world applications highlight the growing adoption of automated lighting solutions for various environments.
Cost Savings and Efficiency
The automated system directly contributes to cost savings and enhanced efficiency in washroom facilities. By optimizing energy consumption and minimizing unnecessary lighting use, the system lowers electricity bills. This cost reduction is a key benefit for facility managers and owners, allowing them to allocate resources more effectively. Moreover, the system’s automation contributes to a more sustainable facility management approach, which aligns with modern environmental concerns.
Illustrative Examples
This section provides concrete examples of how the automated washroom light system responds to various scenarios, demonstrating its adaptability and functionality. These examples showcase how the system can be configured to optimize light usage and user experience across different washroom environments.The system’s flexibility allows for diverse settings and timings, ensuring that the lights are on only when necessary, conserving energy and enhancing user comfort.
Different scenarios, from busy public restrooms to less frequented facilities, are addressed, showing the system’s universal applicability.
Typical Washroom Scenarios
Various washroom scenarios demand different light control strategies. Understanding these scenarios is crucial for effective system implementation.
- High-Traffic Washrooms: These areas benefit from lights that activate consistently upon entry and remain illuminated for a set duration. This ensures sufficient lighting during peak hours while avoiding unnecessary energy consumption during periods of low usage. The Arduino system can be programmed to respond to sensor input (e.g., motion detectors) and maintain a specified illumination duration, such as 30 seconds, to optimize energy use and prevent prolonged lighting.
- Low-Traffic Washrooms: In contrast to high-traffic areas, these washrooms might require more nuanced control. The system can be programmed to illuminate the space only when motion is detected, minimizing energy waste during periods of inactivity. An example might involve a timed illumination, activating for a shorter period (e.g., 15 seconds) upon detecting movement.
- Washrooms with Timed Use: For areas with specific time constraints (e.g., night-time washrooms in a hospital or hotel), the system can be programmed to automatically switch off the lights after a predetermined period (e.g., 5 minutes of inactivity). This ensures that lights are not left on unnecessarily and contributes to energy savings.
System Adaptation to Different Time of Day
The Arduino-based system effectively adapts to various times of day, adjusting light control strategies accordingly. This responsiveness is crucial for optimizing energy consumption and user comfort.
- Daylight Savings: The system’s programming can be modified to adjust the timing of light activation and deactivation based on daylight savings time. This ensures the system operates efficiently throughout the year without requiring manual adjustments. The system can be programmed to account for the time change, adjusting the start and end times for automatic lighting control.
- Night-Time Operation: The system can be programmed to automatically adjust the lighting levels or switch to a different lighting mode (e.g., lower intensity) at night. This feature minimizes energy use during non-peak hours, while still ensuring visibility. For instance, the system could transition to a lower light mode at 10 PM.
- Early Morning: In environments where washrooms are used early in the morning, the system can be programmed to activate lights for a shorter period (e.g., 20 seconds) upon detection of movement, adjusting the sensitivity of the motion sensors to account for lower-intensity movement.
System Operation under Different Conditions
The system’s robustness allows it to operate effectively under various conditions, including varying levels of ambient light and sensor interference.
- High Ambient Light: The system’s light control mechanism is designed to function effectively even in environments with high ambient light levels. It will activate lights only when needed, ensuring that light output is optimal without being excessively bright.
- Sensor Interference: The system’s programming accounts for potential sensor interference, such as false triggers from external factors. The system will implement a delay or threshold mechanism to prevent unnecessary light activation due to external factors, ensuring accuracy in light control.
- System Maintenance: The system’s software design allows for simple updates and modifications to ensure it remains functional. It can also be configured to automatically report maintenance issues.
Setting Up the System in Different Environments
The system’s setup can be tailored to suit various washroom environments, ensuring optimal performance and user experience.
Environment | Setup Steps |
---|---|
Small Washroom | 1. Connect sensors and LEDs. 2. Configure the Arduino code to activate lights for a short duration upon motion detection. 3. Test the system and make necessary adjustments. |
Large Washroom | 1. Install multiple sensors strategically throughout the washroom. 2. Configure the Arduino code to activate lights when motion is detected in any zone. 3. Test the system and make adjustments as needed to optimize light coverage and energy efficiency. |
Washroom with Existing Wiring | 1. Identify existing wiring and connect sensors accordingly. 2. Configure the Arduino code to integrate with the existing wiring and control lights based on sensor input. 3. Test the system to ensure compatibility with the existing infrastructure. |
Last Recap
In conclusion, the Arduino automated washroom light project offers a practical and effective solution for optimizing washroom lighting. By integrating sensors, actuators, and Arduino programming, the project demonstrates the potential for energy savings and improved user experience. The detailed breakdown of hardware, software, and design considerations provides a comprehensive guide for anyone interested in replicating or modifying this smart lighting system.
Post Comment