Getting Started Ruby On Rails
Getting started Ruby on Rails is a journey into the fascinating world of web development. This guide provides a comprehensive overview, covering everything from initial setup to deployment, equipping you with the knowledge and skills needed to create dynamic web applications. Learn about models, controllers, views, and database interactions, all while understanding the core features and benefits of Ruby on Rails.
This comprehensive tutorial will walk you through the essential steps of setting up a Rails project. From understanding the project structure to utilizing gems, you’ll gain hands-on experience building a functional web application. We’ll also delve into crucial concepts like routing, handling user input, and implementing essential testing strategies. The guide also highlights the key differences between Rails and other popular web frameworks like Django and Flask, offering valuable context for your learning.
Introduction to Ruby on Rails
Ruby on Rails is a popular open-source web framework written in Ruby. It’s known for its convention-over-configuration approach, which streamlines development by providing a standardized structure and a set of pre-defined practices. This significantly reduces the amount of boilerplate code needed and allows developers to focus on application logic. Its strong emphasis on security, maintainability, and scalability makes it a reliable choice for building robust web applications.The framework’s core features, including its Model-View-Controller (MVC) architecture and active record, promote clean code and modularity.
This architecture facilitates efficient development and testing, contributing to higher quality applications. Rails also boasts a vast community, providing ample resources, tutorials, and support for developers.
Core Features of Ruby on Rails
Rails offers a structured approach to web development. Its Model-View-Controller (MVC) architecture neatly separates application logic, presentation, and data access. This design improves code organization and maintainability. The framework’s Active Record facilitates interaction with databases, simplifying data manipulation tasks. The robust scaffolding feature expedites project setup and prototyping.
Typical Development Workflow
The Rails development workflow typically begins with defining the application’s structure, using the scaffolding feature to create basic controllers, models, and views. Next, developers add specific functionality to each component, following the MVC pattern. Thorough testing is crucial throughout the process, using tools like RSpec to ensure the application’s correctness. Finally, deployment to a server, often using tools like Capistrano, ensures the application is accessible to users.
Essential Tools and Technologies
A basic Rails project typically requires Ruby, the programming language underlying Rails, and a database (like PostgreSQL or MySQL). A text editor or Integrated Development Environment (IDE) like RubyMine or VS Code is also necessary. Essential command-line tools like `rails` are vital for managing the project. Gems, or Ruby packages, extend functionality and provide extra tools for specific tasks.
Comparison with Other Web Frameworks
Feature | Ruby on Rails | Django | Flask |
---|---|---|---|
Philosophy | Convention over configuration, emphasizes rapid development and structure | Batteries included, comprehensive features out of the box | Minimalist, flexible, allows developers to choose their tools |
Learning Curve | Generally considered relatively easier to learn initially due to the structure | Often perceived as having a slightly steeper learning curve, given the broader feature set | Offers a low learning curve due to its lightweight nature, but deeper understanding is needed for complex projects |
Community Support | Large and active, with extensive resources available | Large and active community, particularly for enterprise-level applications | A supportive community, though perhaps less extensive than Rails or Django |
Scalability | Highly scalable, built with scalability in mind | Known for its scalability and ability to handle large-scale applications | Can be scaled effectively, often depending on the specific choices made |
The table above highlights key distinctions between Rails, Django, and Flask, providing a comparative overview of their philosophies, learning curves, and community support. Choosing the right framework depends on specific project needs and developer experience.
Setting up a Rails Project
Getting a Rails project up and running is straightforward. The process involves initializing a new application, structuring the project directory, installing necessary dependencies, and optionally choosing initialization methods. This section details the steps involved.Setting up a Rails project involves several key steps, from creating the initial directory structure to installing required libraries. Proper setup ensures a robust and functional Rails application.
Initializing a New Rails Application
The `rails new` command is the cornerstone of creating a new Rails application. It generates the fundamental files and directories required for the project. The basic command looks like this:
rails new my_app
This command creates a directory named `my_app` containing all the necessary initial files. You can customize the application by adding options to the `rails new` command. For instance, to create a project with specific database configurations or with a specific gem, you can use additional arguments.
Directory Structure of a Rails Application
A typical Rails application directory has a well-defined structure. The `my_app` directory, created by the `rails new` command, contains various essential subdirectories and files. Crucially, this structure enables the efficient organization of code and assets. A few key directories include:
app
: This directory holds the application’s core logic, including controllers, models, views, and helpers.config
: This directory houses configuration files, such as database settings, and other application-specific settings.db
: This directory manages the database schema and migrations.lib
: This directory stores custom Ruby code not directly related to core Rails functionality.public
: This directory holds static assets, such as images, stylesheets, and JavaScript files.test
: This directory contains unit tests and integration tests for the application.vendor
: This directory holds external libraries and gems used by the application.
Installing Dependencies and Gems
Rails relies on gems (Ruby libraries) for functionality beyond the core framework. Installing these gems is a crucial step. You can manage gems using the `bundle` command, typically within the project’s root directory. The process usually involves these steps:
- Run `bundle install` to install all the gems listed in the `Gemfile`.
- Verify the gems’ installation status by checking the `Gemfile.lock` file, which contains the precise versions of the installed gems.
Comparison of Project Initialization Options
The `rails new` command offers various options for initializing a project. Different options suit various project needs.
Option | Description | Use Case |
---|---|---|
rails new my_app --database postgresql |
Initializes a project with a PostgreSQL database. | Projects requiring a PostgreSQL database. |
rails new my_app --skip-bundle |
Skips the installation of the bundle. | Projects where the bundling process is handled manually. |
rails new my_app --webpack |
Includes Webpack support for JavaScript. | Projects using JavaScript frameworks and requiring Webpack. |
Understanding Rails Models
Models in Rails are the bridge between your Ruby code and your database. They represent the structure of your data and provide a way to interact with it. They define the attributes (like name, email, or age) and behaviors (like saving, updating, or deleting data) for each type of data you want to store.Models are fundamental to building robust applications.
They encapsulate the logic for managing data, ensuring data integrity, and facilitating efficient database interactions. This separation of concerns keeps your code organized and maintainable.
Defining Models with Active Record
Active Record is Rails’s object-relational mapper (ORM). It simplifies database interactions by allowing you to work with your data as Ruby objects. You define your models in Ruby classes, mapping attributes to columns in your database tables.
Relationship between Models and Databases
Models are closely tied to database tables. Each model corresponds to a specific table in your database. Attributes in your model become columns in the table, and each instance of the model corresponds to a row in the table. Active Record automatically handles the mapping between your Ruby objects and the database, simplifying the process of working with data.
Creating a User Model
Let’s create a simple `User` model. This model will represent users in our application. We’ll define attributes for a user’s name, email, and password.“`rubyclass User < ApplicationRecord has_secure_password validates :email, presence: true, uniqueness: true validates :name, presence: true end ``` This code snippet defines the `User` model. The `has_secure_password` method adds password security features, handling password hashing. The `validates` method enforces constraints on the `email` and `name` attributes, ensuring they are present and unique for email.
Migrating the Database
To create the corresponding table in the database, we use migrations. Migrations are classes that describe changes to the database schema.“`rubyrails generate migration CreateUsers users:name,email,password_digest“`This command generates a migration file that adds the `users` table with the columns specified in the command.
This command will automatically generate the necessary migration file.“`ruby# db/migrate/[date]_create_users.rbclass CreateUsers < ActiveRecord::Migration[7.0] def change create_table :users do |t| t.string :name t.string :email t.string :password_digest t.timestamps end end end ``` The `timestamps` column automatically adds created_at and updated_at columns to track when the record was created and last modified. After running the migration, you will have a `users` table in your database with the defined columns. ```bash rails db:migrate ``` This command runs the migration, creating the `users` table with the specified columns in your database.
Building Controllers and Views
Rails controllers act as intermediaries between user requests and your application’s logic. They receive requests, interact with models to fetch or modify data, and then instruct the view to display the results. Controllers are crucial for managing user interactions and data flow in a structured and organized manner.Controllers handle the “behind-the-scenes” work of processing user requests, making decisions based on the data, and ultimately presenting the appropriate view to the user.
Views, on the other hand, focus solely on presenting data to the user in a user-friendly format. This separation of concerns is fundamental to building maintainable and scalable web applications.
Controller Role in Handling Requests
Controllers are the central processing units of your Rails application. They receive incoming requests from users (e.g., clicking a link, submitting a form) and determine the appropriate action to take. This includes fetching data from the database, performing calculations, or updating records. They then pass the results to the view for display.
Creating Controllers in Ruby
Creating a controller in Rails involves defining a class that inherits from `ApplicationController` (or a more specific controller base class). This class contains methods that correspond to specific actions or functionalities within your application. Each method handles a particular request type.
View Structure and Relationship to Controllers
Views are templates that display data to the user. They are closely tied to controllers, receiving data from them and rendering it in a user-friendly format. Views typically use HTML, CSS, and JavaScript to create the user interface. Controllers decide which view to render based on the request.
Example: Creating a Simple Controller and View
“`ruby# app/controllers/products_controller.rbclass ProductsController < ApplicationController def index @products = Product.all end end ``` ```html+erb # app/views/products/index.html.erb
-
<% @products.each do |product| %>
- <%= product.name %>
-$ <%= product.price %>
<% end %>
“`This example shows a `ProductsController` with an `index` action. This action fetches all products from the `Product` model and assigns them to the `@products` instance variable. The corresponding view iterates through these products and displays them as a list. This clear separation of concerns allows for easier maintenance and modification of both the data logic and presentation.
Rendering Different Layouts in Views
Rails allows you to use different layouts for different views or parts of your application. A layout is a template that defines the overall structure of a page, including headers, footers, and other common elements. Views can inherit these elements, ensuring consistency across the application.“`html+erb# app/views/layouts/application.html.erb
Working with Databases
Interacting with databases is a crucial aspect of web application development. Rails provides a powerful and intuitive way to manage data using Active Record, abstracting away much of the complex database interaction details. This streamlined approach allows developers to focus on application logic rather than low-level database queries.Active Record provides a robust set of methods for performing common database operations.
This includes handling the creation, retrieval, updating, and deletion of data, often referred to as CRUD operations. It also simplifies defining relationships between different data entities, such as linking users to their posts or products to their categories.
Active Record CRUD Operations
Active Record handles CRUD operations in a developer-friendly manner. Instead of writing raw SQL queries, developers interact with the database through Ruby objects. This approach enhances code readability and maintainability. For example, to create a new record, you interact with the corresponding model object, rather than directly querying the database.
- Creating (Create): To create a new record, you typically use the `create` or `new` methods on the model object. These methods allow you to populate attributes of the record with values. For example, to create a new `User` record, you’d use the `User.create` method with a hash containing the user’s details.
- Reading (Read): Retrieving data from the database is easily achieved using Active Record’s query methods. You can use methods like `find`, `where`, `first`, `last`, or `all` to fetch specific records or sets of records. For instance, finding a specific user by ID would use `User.find(1)`. To fetch all users, use `User.all`.
- Updating (Update): Modifying existing records involves using the `update` method or assigning new values to attributes. For example, updating a user’s email address involves finding the user and updating the `email` attribute. The `update_attributes` method can also be used.
- Deleting (Delete): Deleting records is straightforward. The `destroy` method on the model object efficiently removes the record from the database. For example, deleting a user can be accomplished using `User.destroy(1)`.
Defining Model Relationships
Rails facilitates defining relationships between models using associations. Common relationships include one-to-one, one-to-many, and many-to-many. These associations simplify complex data structures and operations, automating the management of related data. For instance, a `Post` model might have a `User` model as a foreign key, linking the post to the user who created it.
- One-to-One Relationships: A single record in one model corresponds to a single record in another model. This is often implemented using the `belongs_to` and `has_one` associations.
- One-to-Many Relationships: A single record in one model can relate to multiple records in another model. The `has_many` and `belongs_to` associations are frequently used.
- Many-to-Many Relationships: Multiple records in one model can relate to multiple records in another model. A join table is often used to manage this type of relationship, often handled automatically by Rails.
Database Interactions in Rails
The following table demonstrates common database interactions using Active Record.
Operation | Ruby Code | Description |
---|---|---|
Create a new user | User.create(name: 'John Doe', email: 'john.doe@example.com') |
Creates a new user record in the database. |
Find a user by ID | user = User.find(1) |
Retrieves a user with ID 1 from the database. |
Update a user’s email | user.update(email: 'new.email@example.com') |
Updates the email address of the user. |
Delete a user | user.destroy |
Deletes the user record from the database. |
Basic Routing and URLs
Routing in Rails is the mechanism that maps URLs to specific actions within your application. This crucial component allows users to navigate your website and interact with different parts of the application. Understanding how routing works is fundamental to building dynamic and user-friendly web applications.Routing in Rails acts as a dispatcher, directing incoming requests to the appropriate controller and action.
This centralized system keeps your application organized and efficient. It simplifies the process of handling various user interactions, such as viewing a product page, submitting a form, or accessing a specific user profile.
Route Structure in a Rails Application
Rails uses a configuration file, `config/routes.rb`, to define how URLs are mapped to controllers and actions. This file acts as a central hub for managing all routes within the application. It uses a declarative syntax, making it readable and maintainable.
Defining Routes for Different Actions
Routes are defined using the `resources` method in the `config/routes.rb` file. This method automatically generates routes for common actions like `index`, `show`, `new`, `create`, `edit`, and `update`. For example, `resources :products` generates routes for viewing all products (`products`), showing an individual product (`products/:id`), creating a new product (`new`), and so on.
Creating Routes for Various Web Pages
The following examples illustrate how to create routes for different web pages.
- Viewing a list of all products: The route `products` maps to the `index` action of the `ProductsController`. This allows users to see a list of all products on a page.
- Viewing a specific product: The route `products/:id` maps to the `show` action of the `ProductsController`. This displays details for a specific product based on the product’s ID.
- Creating a new product: The route `products/new` maps to the `new` action of the `ProductsController`. This presents a form for creating a new product.
- Creating a new user: The route `users/new` maps to the `new` action of the `UsersController`. This displays a form for creating a new user.
- Editing a specific product: The route `products/:id/edit` maps to the `edit` action of the `ProductsController`. This allows users to modify details of a specific product.
These are just a few examples. Rails provides a flexible system for defining custom routes to accommodate various application needs. You can customize the routes to match specific URLs and actions required for your project.
Example Route Definitions
Here are some examples of how to define routes using the `resources` method and custom routes:“`ruby# config/routes.rbRails.application.routes.draw do resources :products resources :users get ‘/contact’, to: ‘pages#contact’ root to: ‘products#index’ #sets the home pageend“`The `get ‘/contact’, to: ‘pages#contact’` line defines a specific route for a contact page. The `root to: ‘products#index’` line sets the default home page route.
By combining `resources` and custom routes, you can manage different URLs effectively.
Handling User Input and Validation: Getting Started Ruby On Rails
User input is a crucial aspect of any web application. It’s vital to ensure that the data received from users is accurate, consistent, and adheres to the application’s requirements. Proper validation is paramount to preventing unexpected behavior, security vulnerabilities, and data corruption.
Handling User Input in Rails
Rails provides robust mechanisms for handling user input, primarily through form helpers and model validations. Forms, which are the primary interface for receiving user input, are created using these helpers. These helpers generate the HTML form elements and automatically handle data submission to the server.
Validating User Input
Input validation is a critical step in securing and maintaining the integrity of data within a Rails application. Validating user input helps prevent unexpected behavior, errors, and security vulnerabilities. It ensures the input matches the expected format, type, and range, safeguarding the application from malicious or poorly formatted data. This is achieved through a combination of client-side validation (using JavaScript) and server-side validation (using Ruby code in your models).
Validation Technique | Description | Example (in Ruby) |
---|---|---|
Presence | Ensures a field is not left empty. | validates :name, presence: true |
Length | Specifies the minimum or maximum length of a string. | validates :password, length: minimum: 8 |
Format | Ensures input conforms to a specific pattern. | validates :email, format: with: URI::MailTo::EMAIL_REGEXP |
Numericality | Validates that a field contains a number within a specific range. | validates :age, numericality: greater_than_or_equal_to: 0 |
Uniqueness | Guarantees that a field value is unique across all records. | validates :email, uniqueness: true |
Inclusion | Validates that a value is present in a specified list. | validates :status, inclusion: in: %w[active pending] |
Using Form Helpers
Rails form helpers simplify the creation of forms. These helpers generate the HTML form elements for you, making the process of creating forms more streamlined and less error-prone. They also automatically handle data submission to the server, making the interaction between the user interface and the application backend more efficient. This automation helps developers focus on the logic and functionality of their applications rather than getting bogged down in the technical details of form creation.
For example, to create a form for a user’s name and email:
<%= form_with(model: @user) do |form| %>
<%= form.label :name, "Name" %>
<%= form.text_field :name %>
<%= form.label :email, "Email" %>
<%= form.email_field :email %>
<%= form.submit %>
<% end %>
This code snippet demonstrates how to use the form_with
helper to create a form for a user object. The helper automatically generates the necessary HTML elements, including input fields for name and email, and a submit button. This significantly reduces the amount of boilerplate code required.
Introduction to Testing in Rails
Testing is crucial for building robust and reliable web applications. It helps identify bugs early in the development process, ensures code quality, and facilitates easier maintenance as the application grows. Thorough testing minimizes the risk of unexpected behavior and improves the overall user experience.
Importance of Testing in Web Applications
Testing in web application development ensures the application functions as intended, preventing unexpected behavior or errors that might affect user experience or data integrity. Comprehensive testing is a crucial aspect of the software development lifecycle, enabling developers to confidently deploy and maintain their applications.
Testing Frameworks in Rails
Rails offers various testing frameworks, but RSpec (Ruby Specification) is a popular choice due to its expressive syntax and rich features. RSpec allows for writing clear and concise tests, making the testing process more efficient and readable. It promotes a behavior-driven development (BDD) approach, focusing on defining what the code should do rather than how it should do it.
Writing Unit Tests for Models
Unit tests isolate individual components of the application, such as models, to verify their functionality in isolation. This approach helps in detecting bugs early and improves code maintainability. A well-structured unit test ensures that the model methods respond correctly to different inputs and edge cases.
Example: Creating a Simple Model Test Case in RSpec
“`rubyrequire ‘rails_helper’RSpec.describe User, type: :model do describe ‘validations’ do it ‘requires a non-empty username’ do user = User.new(username: ”, email: ‘test@example.com’) expect(user).not_to be_valid expect(user.errors[:username]).to include(“can’t be blank”) end it ‘requires a valid email format’ do user = User.new(username: ‘testuser’, email: ‘invalid_email’) expect(user).not_to be_valid expect(user.errors[:email]).to include(“is invalid”) end endend“`This example demonstrates a basic RSpec test for the `User` model.
It checks for validation rules, specifically ensuring the username is not blank and the email address is in a valid format. The `rails_helper` is a necessary component for setting up the testing environment. The `type: :model` option specifies that the test is for the model. The `expect` method is used to assert the expected behavior. The `describe` block groups related tests, making the code more organized.
Writing Unit Tests for Controllers
Controller tests verify that controllers handle requests appropriately, interacting with models and views. These tests help ensure that the application’s logic works as expected when receiving user input or making database queries. Comprehensive controller tests validate the controller’s responses, ensuring data consistency and avoiding errors that might affect the user experience.
Example: Creating a Simple Controller Test Case in RSpec
“`rubyrequire ‘rails_helper’RSpec.describe UsersController, type: :controller do describe ‘GET #index’ do it ‘returns a success response’ do get :index expect(response).to have_http_status(:ok) end endend“`This concise example shows a basic RSpec test for a `UsersController`’s `index` action. It verifies that the controller returns a successful HTTP response (status code 200) when the user requests the index page.
The `get` method simulates a GET request. The `expect` method is used to verify the response status. The `describe` block groups related tests, providing clear structure.
Essential Gems and Libraries
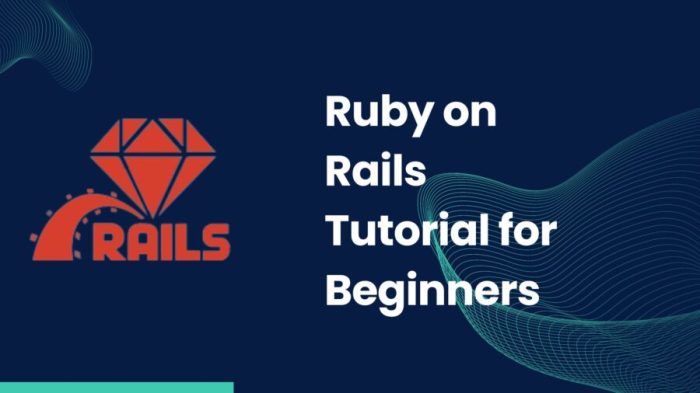
Source: shopaccino.com
Rails projects often benefit from adding extra functionality through gems. These gems are essentially pre-built packages of code that extend the core Rails framework. Using gems can significantly speed up development and provide specialized features, saving you time and effort.Gems provide a structured way to manage external libraries and dependencies within your Rails application. Proper gem management ensures your application’s stability and compatibility with other parts of the project.
It allows you to easily upgrade and update dependencies as needed, maintaining a clean and organized project structure.
Identifying Essential Gems, Getting started Ruby on Rails
Rails projects frequently leverage a collection of essential gems for various tasks. These gems provide specialized functionalities, such as database migrations, authentication, email handling, and more. Choosing and incorporating these gems is a key part of optimizing and enhancing your application’s functionality.
Installing Gems with Bundler
Bundler is a crucial tool for managing gem dependencies in a Rails project. It creates and manages a Gemfile, a file that lists all the gems your application needs. This file ensures that all necessary gems are installed and that their versions are compatible with each other.To install Bundler, you can run the following command in your terminal:“`gem install bundler“`Once Bundler is installed, create a Gemfile in your project’s root directory.
This file will list the gems your application requires. For example:“`rubygem ‘activerecord’gem ‘bcrypt’gem ‘rails’“`Then, run the command:“`bashbundle install“`This command installs all the gems listed in your Gemfile and their dependencies.
Managing Dependencies with Bundler
Bundler ensures that all your project’s gems are installed and that their versions are compatible. This is critical for maintaining a stable and predictable project environment.Updating dependencies is essential to benefit from bug fixes and new features. Use the command:“`bashbundle update“`This command checks for newer versions of the listed gems and updates them if available.
Essential Gems for Common Tasks
Using gems effectively streamlines the development process. The following list presents common tasks and the corresponding essential gems for each.
- Authentication: Gems like Devise simplify user authentication and authorization. They provide a framework for managing user accounts, logins, and permissions.
- Email Handling: Action Mailer facilitates sending emails from your application. It provides a structured approach for composing and sending emails, ensuring reliable and efficient communication.
- Testing: Rspec and Factory Bot are vital for writing comprehensive tests for your application. They help ensure that your code functions as expected and that new features don’t introduce bugs.
- Database Interactions: ActiveRecord is a powerful ORM that simplifies database interactions. It provides a way to interact with your database without writing complex SQL queries.
Task | Gem | Description |
---|---|---|
User Authentication | Devise | Provides a complete framework for user authentication and authorization. |
Email Handling | Action Mailer | Manages sending emails within your application. |
Testing | RSpec, Factory Bot | Tools for writing comprehensive tests for your application. |
Database Interactions | ActiveRecord | Simplifies database interactions through an object-relational mapper. |
Deployment and Hosting
Deploying a Rails application involves getting it up and running on a server accessible to users. This process involves several steps, from choosing the right platform to configuring the server environment. Successful deployment ensures your application is available and accessible to the target audience.Deploying a Rails application goes beyond just writing code; it requires understanding server infrastructure, security measures, and scalability.
Different deployment platforms offer various features and levels of support, which are crucial factors in selecting the best fit for your project.
Deployment Options
Several options are available for deploying Rails applications, each with its own strengths and weaknesses. These options vary in complexity and cost, offering varying levels of support and features.
- Heroku: A popular cloud platform for deploying and scaling applications quickly and easily. It offers a free tier for smaller projects, making it ideal for beginners and startups. It handles infrastructure management automatically, allowing developers to focus on the application itself. Heroku’s intuitive interface and readily available support make it a common choice for Rails development.
- AWS (Amazon Web Services): A comprehensive cloud platform with vast resources and flexibility. It provides a greater degree of control over the deployment process and allows customization for more demanding projects. AWS allows for detailed configuration, which can be crucial for high-traffic applications or those requiring specialized setups.
- DigitalOcean: A cloud platform known for its affordability and control over server infrastructure. It provides VPS (Virtual Private Servers) that allow for more fine-grained control and customization. DigitalOcean is a good choice for projects that require a more hands-on approach to server management.
- Other platforms: Other platforms such as Google Cloud Platform (GCP) and Netlify also offer Rails deployment options, catering to specific needs and requirements. Each platform comes with its own pricing model and level of service, and developers need to carefully consider their needs to choose the best one.
Common Deployment Platforms
Deployment platforms streamline the process of getting a Rails application live. Understanding their features helps in selecting the best fit.
- Heroku: Known for its ease of use and rapid deployment, Heroku automates much of the infrastructure setup. It’s popular for its intuitive interface, extensive documentation, and wide range of add-ons for enhanced functionality.
- AWS (Amazon Web Services): AWS offers complete control over the deployment environment, giving developers the flexibility to tailor configurations to their specific needs. This control is particularly valuable for complex applications with high scalability requirements or specific security needs.
Setting Up a Deployment Environment
The process of setting up a deployment environment depends heavily on the chosen platform. Each platform has its own set of instructions and tools.
- Heroku: The process typically involves creating an account, pushing the application code to Heroku using Git, and configuring relevant settings. Heroku guides the user through these steps, making it a relatively simple setup.
- AWS: Setting up an AWS deployment environment involves creating an account, setting up EC2 instances, configuring a database, and installing the necessary tools for the Rails application. This often requires more technical expertise compared to Heroku.
Deployment Platform Comparison
This table provides a concise comparison of various deployment platforms.
Platform | Ease of Use | Control | Scalability | Cost |
---|---|---|---|---|
Heroku | High | Low | Good | Variable (Free tier available) |
AWS | Low | High | Excellent | Variable (Pay-as-you-go) |
DigitalOcean | Medium | Medium | Good | Variable (Pay-per-resource) |
Ultimate Conclusion
In conclusion, this guide has provided a solid foundation for getting started with Ruby on Rails. By understanding the fundamental concepts and practical implementation details, you’re well-equipped to embark on your own web development journey using this powerful framework. This introduction covers the necessary steps from project setup to deployment, and serves as a springboard for further exploration and project creation.
Post Comment