Kotlin Android Beginner’S Guide
Kotlin Android beginner’s guide provides a structured approach to learning Android development using Kotlin. This comprehensive guide covers everything from setting up a basic Android project to advanced Kotlin features like coroutines and data handling. You’ll learn how to create interactive user interfaces, handle data efficiently, and integrate with APIs. Ready to dive into the world of Android development?
This guide is your starting point.
This beginner’s guide to Kotlin Android development will equip you with the fundamental knowledge and practical skills needed to build robust and engaging Android applications. We will cover a wide range of topics, from basic Kotlin syntax to advanced concepts like coroutines and testing. Each section is designed to be progressively challenging, enabling you to build upon your knowledge with practical examples and exercises.
Introduction to Kotlin for Android Beginners: Kotlin Android Beginner’s Guide
Kotlin has rapidly become the preferred language for Android development, offering a modern approach with enhanced safety and conciseness. This guide provides a foundational understanding of Kotlin for Android beginners, covering essential features, setup, and practical examples. It emphasizes the benefits of Kotlin over Java and illustrates key syntax differences with practical examples.Kotlin’s concise syntax and robust features make it a powerful tool for Android development.
This guide will demonstrate how to leverage these advantages in building functional and efficient Android applications.
Kotlin’s Advantages for Android
Kotlin brings several benefits to Android development. Its concise syntax often reduces code size, making it more readable and maintainable. Kotlin’s null safety feature helps prevent common runtime errors. Furthermore, Kotlin interoperates seamlessly with existing Java codebases, allowing for gradual adoption within existing projects.
Setting Up a Basic Android Project with Kotlin
To create a new Android project with Kotlin, use Android Studio. Select “Empty Compose Activity” as the project template. This template provides a basic structure with a user interface built using Jetpack Compose. Ensure you’ve chosen Kotlin as the programming language. The project will be initialized with necessary files and dependencies.
Example Project: Basic Layout and Button Functionality
This example demonstrates a simple Android project with a button. The layout (XML or Compose) defines a screen with a button. The Kotlin code handles button clicks. This involves creating an `Activity` or a `Fragment` (for more complex structures) that listens for button clicks. When the button is clicked, it updates a TextView to display a message.“`kotlin// Example Kotlin code snippet for button click handlingbutton.setOnClickListener textView.text = “Button Clicked!”“`This code snippet shows a basic implementation.
In a real-world application, you’d likely include more robust error handling, dynamic updates, and potentially data fetching from a backend.
Kotlin vs. Java Syntax Comparison
The following table compares Kotlin and Java syntax for a few core Android concepts.
Feature | Kotlin | Java |
---|---|---|
Data Class | “`kotlindata class User(val name: String, val age: Int)“` | “`javaclass User private String name; private int age; // Constructor, getters, and setters“` |
Looping (For Each) | “`kotlinval numbers = listOf(1, 2, 3, 4, 5)numbers.forEach number -> println(number) “` | “`javaList |
Null Safety | “`kotlinvar name: String? = nullif (name != null) println(name.length)“` | “`javaString name = null;if (name != null) System.out.println(name.length()); else // Null check required“` |
This table highlights the conciseness and safety improvements that Kotlin offers. Kotlin’s data classes automate the boilerplate code for creating data objects, reducing the amount of repetitive code compared to Java. Kotlin’s forEach loop simplifies iteration over collections. Kotlin’s null safety features, as demonstrated, prevent common null pointer exceptions that Java developers frequently encounter.
Core Kotlin Concepts for Android
Kotlin’s concise syntax and powerful features make it a popular choice for Android development. Understanding its core concepts is crucial for writing clean, efficient, and maintainable Android applications. This section delves into essential Kotlin concepts, emphasizing their practical application within the Android ecosystem.Kotlin’s core features streamline development, enabling robust and error-resistant code. This section details key concepts, from data classes to coroutines, highlighting their usefulness in creating Android applications.
Data Classes
Data classes are a special type of class designed for representing data. They automatically generate boilerplate code, including constructors, getters, setters, `equals()`, `hashCode()`, and `toString()` methods. This significantly reduces development time and effort.
- Use Case: Representing data objects like user profiles, products, or addresses. For example, a `User` data class might contain fields for name, email, and age.
- Example:
“`kotlin
data class User(val name: String, val email: String, val age: Int)val user = User(“Alice”, “alice@example.com”, 30)
println(user) // Output: User(name=Alice, email=alice@example.com, age=30)
“`
Functions
Functions are fundamental building blocks in Kotlin. They encapsulate blocks of code that perform specific tasks. Kotlin’s functions offer features like named parameters, default values, and lambda expressions, making them flexible and expressive.
- Use Case: Performing calculations, processing data, or interacting with UI elements. For instance, a function to calculate the area of a rectangle.
- Example:
“`kotlin
fun calculateArea(length: Int, width: Int = 10): Int
return length
– widthval area = calculateArea(5) // Uses default width
println(area) // Output: 50val area2 = calculateArea(5, 20) // Uses specified width
println(area2) // Output: 100
“`
Lambda Expressions
Lambda expressions are anonymous functions. They are concise ways to represent small, single-purpose blocks of code. Lambda expressions are frequently used in collections and other functional operations.
- Use Case: Implementing callbacks, processing data in collections, and handling UI events. A lambda expression can easily filter or map items in a list.
- Example:
“`kotlin
val numbers = listOf(1, 2, 3, 4, 5)
val evenNumbers = numbers.filter it % 2 == 0
println(evenNumbers) // Output: [2, 4]
“`
Null Safety
Kotlin’s null safety features help prevent common errors arising from null values. The language enforces the explicit handling of null values, improving code robustness.
- Use Case: Preventing crashes and ensuring data integrity by explicitly checking for null values before using them.
- Example:
“`kotlin
fun greet(name: String?): String
return name?.let “Hello, $it!” ?: “Hello, there!”println(greet(“Alice”)) // Output: Hello, Alice!
println(greet(null)) // Output: Hello, there!
“`
UI Element Handling
Kotlin’s interoperability with Android’s UI elements is seamless. Using Kotlin, developers can easily interact with views, set properties, and handle events.
- Use Case: Creating and manipulating UI elements like buttons, text views, and images, responding to user interactions.
- Example:
“`kotlin
// Example using a Button
val button = findViewById
Coroutines
Kotlin coroutines provide a structured way to handle asynchronous operations. They simplify asynchronous programming, making it easier to write concurrent code.
- Use Case: Downloading data, interacting with APIs, and performing other long-running tasks without blocking the main thread. Coroutines allow for non-blocking operations, essential for responsive user interfaces.
- Example:
“`kotlin
import kotlinx.coroutines.*fun fetchData(url: String): String = runBlocking
withContext(Dispatchers.IO)
// Simulate network request
delay(1000)
return@withContext “Data from $url”// Example usage
runBlocking
val data = fetchData(“https://example.com”)
println(data)“`
Exception Handling
Kotlin’s exception handling mechanism allows for graceful management of errors. Using `try-catch` blocks, developers can handle potential exceptions effectively.
- Use Case: Preventing crashes, logging errors, and providing user-friendly feedback when something goes wrong.
- Example:
“`kotlin
fun processData(input: String)
try
val number = input.toInt()
println(“Processed number: $number”)
catch (e: NumberFormatException)
println(“Invalid input: $e.message”)processData(“123”) // Output: Processed number: 123
processData(“abc”) // Output: Invalid input: For input string: “abc”
“`
Kotlin Type System
Kotlin’s type system plays a vital role in Android development. It ensures type safety, allowing for better code maintainability and reducing potential errors. Type inference further enhances code readability.
- Use Case: Ensuring type correctness, improving code reliability, and facilitating maintainability.
- Example: The type system helps to ensure that data is handled correctly, and potential errors are identified early in the development process.
Android UI Development with Kotlin
Building user interfaces (UIs) is a crucial aspect of Android app development. Kotlin, with its concise syntax and powerful features, simplifies this process. This section will delve into the essentials of Android UI development, covering layouts, views, event handling, and data binding. We’ll explore different layout types, demonstrate common UI components, and provide best practices for creating accessible and user-friendly interfaces.This section provides a practical guide for constructing compelling Android interfaces.
We’ll illustrate how to efficiently design and implement UI elements using Kotlin, ensuring your apps offer a smooth and intuitive user experience.
Layout Types in Android
Understanding the various layout types is essential for structuring your UI effectively. Different layouts cater to different design needs, and choosing the right one can significantly impact performance and maintainability.
- LinearLayout: This layout arranges views in a single row or column. It’s ideal for simple linear arrangements where components need to be stacked horizontally or vertically. Its simplicity makes it a go-to choice for basic layouts.
- RelativeLayout: This layout allows precise positioning of views relative to other views. It offers greater flexibility for complex layouts, but can become cumbersome for intricate designs. It is helpful for aligning elements based on each other’s position.
- ConstraintLayout: This layout provides the most control over view positioning. It uses constraints to define the relationship between views, offering advanced layout management. It’s highly versatile and is often the preferred choice for complex and dynamic layouts. This layout type is particularly well-suited for optimizing the UI, especially for layouts that need a more precise control over the positioning and relationships between views.
Common UI Components
Android provides a rich set of UI components to build diverse interfaces. Knowing how to use them effectively is crucial for creating functional and visually appealing apps.
- TextView: Displays text on the screen. Customize its appearance (font, size, color) and use it to display information to the user.
- Button: Allows users to trigger actions. Handle button clicks to initiate specific tasks.
- EditText: Enables users to input text. Retrieve and process the inputted text.
- ImageView: Displays images. Load and display images from various sources, adapting them to the UI design.
- RecyclerView: Displays lists of items that can be dynamically populated and updated. Use it to present large datasets in an efficient manner.
- ProgressBar: Indicates progress during tasks. Use it to inform users about the progress of a process.
Handling User Input
User input is a cornerstone of interactive applications. Effectively handling user input ensures smooth interaction and allows the app to respond to user actions.
- Buttons: Attach click listeners to buttons to execute actions when pressed. Use `setOnClickListener` to respond to button clicks.
- Text Fields: Attach `onTextChanged` listeners to text fields to react to changes in the input. This is essential for handling real-time updates.
- Other UI elements: Other components such as images or custom views also can be equipped with listeners to react to user interactions.
Data Binding
Data binding is a powerful technique for connecting data to the UI. This approach simplifies the process of updating UI elements when data changes, reducing boilerplate code.
- Simplified UI Updates: Data binding automatically updates UI elements when the underlying data changes, reducing the need for manual updates. This makes the code cleaner and more maintainable.
- Enhanced Efficiency: By binding data directly to UI elements, you can avoid tedious manual updates. This translates into more efficient code.
- Improved Maintainability: Data binding contributes to more maintainable code as changes to the data source automatically reflect in the UI, making it easier to manage and modify the application.
Data Handling and Persistence in Android
Storing and retrieving data efficiently is crucial for any Android application. This section explores various methods for persisting data, focusing on Kotlin and Android best practices. We’ll cover data modeling, different storage mechanisms, and the importance of data validation to ensure robust and reliable applications.
Data Modeling in Kotlin
Effective data modeling is fundamental to managing application data. A well-structured data model ensures data integrity, reduces redundancy, and facilitates efficient querying and manipulation. Kotlin’s data classes, with their automatic implementation of equals(), hashCode(), toString(), and other methods, simplify the creation of robust data models.“`kotlindata class User(val id: Int, val name: String, val email: String)“`This concise definition creates a User data class with essential attributes.
Data classes in Kotlin automatically generate necessary methods for comparison and representation, making your code cleaner and more readable.
Data Storage Mechanisms
Android offers various mechanisms for storing data. Choosing the right approach depends on the nature of the data, required performance, and complexity of the application.
- SharedPreferences: A simple, lightweight solution for storing small amounts of key-value pairs. Suitable for preferences, settings, and user-specific configurations. However, it’s limited in handling complex data structures and is not ideal for large datasets.
- Room: A powerful, object-relational mapping (ORM) library that simplifies interacting with SQLite databases. It provides an abstraction layer, allowing developers to interact with the database using Kotlin data classes. This approach is ideal for handling structured data and ensuring data integrity. Room handles database creation, updates, and queries, making it efficient for applications requiring database interactions.
Using Room for Database Creation
Room facilitates creating and interacting with SQLite databases in a clean and efficient manner. It leverages Kotlin data classes to map to database tables. This example demonstrates creating a simple user database:“`kotlin// User entity@Entitydata class UserEntity( @PrimaryKey(autoGenerate = true) val id: Int = 0, val name: String, val email: String)// DAO interface@Daointerface UserDao @Insert fun insertUser(user: UserEntity) @Query(“SELECT
FROM userentity”)
fun getAllUsers(): List
Data Validation
Validating data is critical to ensure data integrity and prevent unexpected behavior in your application. Data validation should be performed at multiple points, including input fields, database interactions, and application logic. This approach prevents data corruption and application crashes, leading to a more robust application.
Comparison of Data Storage Mechanisms
Mechanism | Data Type | Scalability | Complexity |
---|---|---|---|
SharedPreferences | Key-value pairs | Low | Low |
Room (SQLite) | Structured data (SQL) | Medium to High | Medium |
JSON | Text-based | High | Medium to High |
This table summarizes the key differences between the discussed storage mechanisms. Consider the application’s needs and complexity when selecting a storage method. Room is generally preferred for structured data requiring relational integrity, while SharedPreferences is suitable for simpler configurations. JSON can be a versatile option for large datasets, but often requires more complex parsing and handling.
Efficient Data Handling Techniques, Kotlin Android beginner’s guide
Efficient data handling techniques involve optimizing database queries, caching frequently accessed data, and using asynchronous operations to avoid blocking the main thread. These techniques contribute to a smooth user experience and ensure responsiveness. Asynchronous operations using Kotlin coroutines improve efficiency and avoid blocking the UI thread, ensuring a better user experience.
Networking and API Integration
Connecting your Android app to external APIs is crucial for fetching data, interacting with services, and providing dynamic content. This process involves making HTTP requests to retrieve data and handling responses effectively. Understanding how to integrate and work with APIs is fundamental for building robust and feature-rich Android applications.
Making HTTP Requests with Retrofit
Retrofit is a popular library for simplifying HTTP requests in Android. It provides a type-safe way to define API endpoints and handle responses. Using Retrofit’s annotations, you can map HTTP methods (GET, POST, PUT, DELETE) to specific functions in your code. This approach enhances code readability and maintainability.
Handling JSON Responses
APIs often return data in JSON format. Retrofit allows you to define classes that correspond to the JSON structure. This mapping makes parsing JSON responses straightforward and type-safe. The library handles the deserialization automatically, eliminating the need for manual JSON parsing. Example:“`kotlindata class User(val id: Int, val name: String)// …
in your Retrofit service@GET(“users/id”)suspend fun getUser(@Path(“id”) id: Int): Response
Error Handling and Response Parsing
Robust error handling is essential for network calls. Retrofit provides `Response` objects, which allow you to check for success or failure. This ensures the app handles network issues gracefully.“`kotlintry val response = getUser(1) if (response.isSuccessful) val user = response.body() // Access the User object // …
use the user data else // Handle the error (e.g., log the error, show a message to the user) val errorBody = response.errorBody()?.string() Log.e(“Error”, “Error fetching user: $errorBody”) catch (e: Exception) // Handle network exceptions (e.g., connection problems) Log.e(“Error”, “Network error: $e.message”)“`Error handling should consider different scenarios: network connectivity issues, API errors, and invalid JSON responses.
Integrating Third-Party APIs
Integrating third-party APIs involves defining the API’s endpoints, creating a Retrofit service interface, and making the necessary requests. The specific steps will vary depending on the API’s documentation and structure. Thorough documentation and API specifications are essential to ensure accurate integration. Carefully examine the API’s rate limits and request restrictions to avoid service disruptions or account limitations.
Basic Network Call and Data Parsing Example
“`kotlin// … Retrofit setup (omitted for brevity)// Example API callsuspend fun fetchUserData(userId: Int): User? val response = apiService.getUser(userId) return if (response.isSuccessful) response.body() else // Handle the error (e.g., log it, display an error message) null “`
Networking Library Comparison
Library | Pros | Cons |
---|---|---|
Retrofit | Type-safe, easy to use, efficient, widely adopted | Steeper learning curve compared to simpler libraries |
OkHttp | Powerful, flexible, gives granular control | Requires more manual setup and error handling |
Volley | Lightweight, efficient for simple requests | Less powerful for complex APIs, fewer features than Retrofit |
This table summarizes the advantages and disadvantages of commonly used networking libraries. Choose the library that best suits the complexity of your application’s needs.
Testing and Debugging in Kotlin Android
Testing and debugging are crucial components of the Android development lifecycle. Thorough testing ensures your application functions as expected and reduces bugs in production. Effective debugging helps pinpoint and fix issues rapidly. These practices are essential for building robust and reliable Android applications.Effective testing and debugging strategies are vital for ensuring high-quality Android applications. By incorporating robust testing procedures, developers can proactively identify and resolve potential issues early in the development cycle, ultimately leading to a more polished and reliable user experience.
Different Testing Approaches for Android Applications
Different testing approaches target various aspects of your application. Unit tests isolate individual components, while integration tests verify interactions between components. Instrumentational tests simulate user behavior and interactions within the Android environment. Choosing the right approach depends on the specific component or functionality being tested.
Writing Unit Tests
Unit tests are focused on verifying the functionality of individual units (e.g., functions, classes) in isolation. This isolation prevents external dependencies from influencing the test results. This approach allows for quicker identification and resolution of issues.
Example of a Unit Test
“`kotlinimport org.junit.Testimport org.junit.Assert.*class Calculator fun add(a: Int, b: Int): Int return a + b class CalculatorTest @Test fun testAdd() val calculator = Calculator() val result = calculator.add(2, 3) assertEquals(5, result) “`This example demonstrates a unit test for the `add` function in a `Calculator` class.
The `@Test` annotation marks the `testAdd` function as a test case. The `assertEquals` function verifies that the result of the `add` function is as expected. Junit is a popular testing framework.
Writing Integration Tests
Integration tests verify the interaction between different components of an application. They confirm that these components work together seamlessly. This approach helps ensure that your application behaves as expected under real-world conditions.
Debugging Kotlin Android Code
Debugging tools help identify and resolve issues within your code. Stepping through code line by line, inspecting variables, and setting breakpoints are essential debugging techniques. These tools are crucial for understanding the execution flow and identifying the root cause of errors. Understanding the debug logs produced by the Android application is an essential part of debugging.
Tips for Writing Maintainable and Testable Kotlin Android Code
Writing maintainable and testable code is crucial for long-term project success. Keep your code modular and well-structured, making it easier to understand and modify. Favor smaller, focused functions to promote reusability. Proper use of classes and interfaces improves the organization and clarity of your codebase. The use of interfaces and abstract classes encourages the separation of concerns.
Organizing Tests for a Complex Android Application
A well-organized testing strategy is crucial for managing tests in complex applications. Group tests by modules or features to manage test execution and maintainability. Separate unit tests from integration tests and UI tests to streamline the testing process. Using a testing framework (like JUnit) allows for easy setup and organization. The use of test runners allows for efficient and effective execution of tests.
Consider using a test runner for different test types.
Advanced Kotlin Features for Android
Kotlin offers a rich set of advanced features that significantly enhance Android development. These features, including coroutines, flows, sealed classes, reflection, and custom views, provide powerful tools for building robust, efficient, and maintainable applications. Mastering these techniques will empower you to tackle complex tasks with elegance and precision.
Coroutines for Asynchronous Tasks
Coroutines provide a powerful and elegant way to handle asynchronous tasks in Kotlin. They are lightweight, non-blocking concurrency primitives that simplify the management of background threads and improve the responsiveness of your application. Using coroutines avoids the complexities of traditional threads and callbacks, leading to cleaner and more maintainable code.Implementing a background task with coroutines involves leveraging the `CoroutineScope` and suspending functions.
A suspending function pauses the execution of the coroutine until the task completes. The `CoroutineScope` provides the context for the coroutine’s execution.
Example of a Background Task with Coroutines
“`kotlinimport kotlinx.coroutines.*suspend fun downloadImage(imageUrl: String): Bitmap? // Simulate network request delay(2000) // Simulate network delay return // Return a Bitmap or nullfun fetchDataFromNetwork(imageUrl: String) CoroutineScope(Dispatchers.IO).launch val image = withContext(Dispatchers.Default) downloadImage(imageUrl) if (image != null) // Update UI with downloaded image // …
“`This example showcases how to download an image using a coroutine. The `downloadImage` function simulates a network request and suspends the coroutine. The `withContext` function ensures that the blocking operation happens on the appropriate dispatcher. This pattern prevents blocking the main thread and ensures smooth UI interactions.
Flows for Handling Streams of Data
Kotlin’s Flow API facilitates the handling of streams of data. Flows are cold, asynchronous sequences of values. They are a powerful tool for managing data streams, enabling the creation of reactive programming patterns. Flows are composable, making it easy to chain multiple transformations and operations.Flows are particularly well-suited for handling data updates from sources like network APIs, sensors, or user interactions.
They enable a declarative style of programming, simplifying the expression of data transformations and handling.
Sealed Classes for Type Safety
Sealed classes in Kotlin are a powerful tool for defining a set of mutually exclusive types. They enhance type safety by ensuring that all possible types are accounted for. This feature prevents unexpected runtime errors caused by missing cases.The use of sealed classes promotes maintainability and code readability. They help ensure the exhaustive handling of different states or scenarios, eliminating potential issues in a structured and explicit manner.
Reflection Capabilities for Advanced Features
Kotlin’s reflection capabilities allow introspection of classes, methods, and properties at runtime. This flexibility enables advanced features like dynamic code generation, custom serialization, and implementing powerful generic solutions. Reflection allows for creating dynamically configurable applications.
Implementing a Custom View in Kotlin
Creating a custom view in Kotlin involves extending the `View` class. You define the view’s appearance, behavior, and interactions by overriding methods like `onDraw` and `onTouchEvent`. This process allows for creating views that meet specific application requirements.A custom view often requires defining attributes, handling events, and implementing logic for drawing and updating the view. This enables you to tailor the visual and interactive aspects of the view to your specific application needs.
Ultimate Conclusion
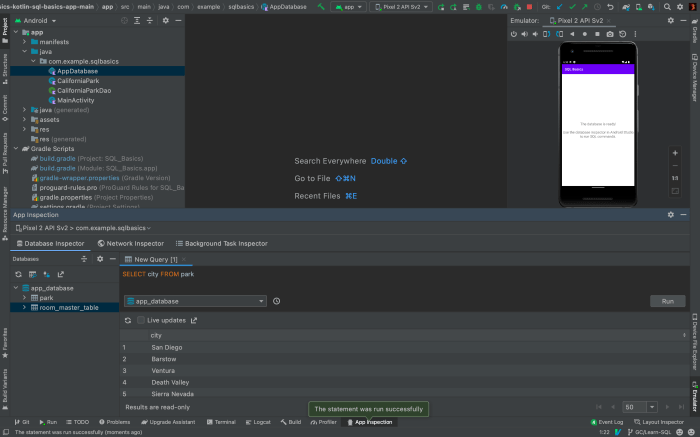
Source: githubusercontent.com
In conclusion, Kotlin Android beginner’s guide has provided a thorough exploration of the fundamentals of Android app development with Kotlin. From setting up your project to integrating APIs, we’ve covered a wide spectrum of essential skills. We’ve delved into Kotlin’s core features, Android UI development, data handling, networking, and testing. Now you’re well-equipped to embark on your Android development journey.
Happy coding!
Post Comment