How To Comment Code Effectively
How to comment code effectively is crucial for any programmer. Clear and concise comments significantly improve code readability and maintainability, especially for projects with multiple developers or those requiring future updates. This guide will explore various aspects of effective commenting, from understanding different comment types to crafting clear and descriptive content. We’ll cover best practices, common mistakes to avoid, and advanced techniques for enhancing your comments.
This comprehensive guide will equip you with the knowledge and skills to create high-quality comments that greatly benefit both yourself and other developers working with the code.
Understanding Code Comments’ Purpose
Code comments are essential for maintaining and understanding code, especially in larger projects. They act as a form of documentation, explaining the rationale behind specific code sections, clarifying complex logic, and providing context for future modifications. Effective commenting enhances code readability and aids in collaborative development.Comments serve as a vital bridge between the code’s structure and its underlying intent.
Without proper comments, even experienced developers may struggle to comprehend the intricacies of a codebase after a period of time. This is particularly crucial in team environments, where multiple developers collaborate on a project.
Primary Reasons for Writing Code Comments
Comments are not merely decorative; they serve several crucial purposes. They provide explanations for non-obvious code, document design choices, and help maintain the code’s clarity. Clear comments make it easier for others (and yourself in the future) to understand the code’s function and logic. This is especially valuable in situations where code might require modification or maintenance after a period of inactivity.
Different Types of Code Comments
Code comments come in various forms, each with its own use case. Understanding these different types allows developers to utilize comments effectively.
- Single-line Comments: These comments are used for brief explanations or notes on a single line of code. They are often used to indicate the purpose of a specific line or a small block of code. They start with two forward slashes (//) and are commonly placed on the same line as the code they describe. This makes them easily identifiable and straightforward.
- Multi-line Comments: Multi-line comments are employed for more extensive explanations or notes spanning several lines. They are particularly helpful for documenting complex logic or algorithms. They are enclosed within /*
-/ symbols. This allows for detailed descriptions without disrupting the flow of the code. - Documentation Comments: These comments are specifically designed to generate documentation automatically. Tools can parse these comments to create API documentation, class overviews, or function descriptions. They often follow a specific syntax depending on the programming language. For example, in Java, they use Javadoc format. They are indispensable for creating comprehensive and readily available documentation for a codebase.
Relationship Between Comments and Code Maintainability
Effective commenting directly impacts code maintainability. Well-maintained code is easier to understand, modify, and debug. Clear and concise comments provide crucial context for future developers or even the original author revisiting the code after a period of time. A well-commented codebase minimizes the time spent on understanding the logic and intent behind the code, significantly reducing maintenance efforts.
Examples of Code with and without Comments
The impact of comments on code readability is clearly demonstrated by comparing code with and without comments.
// Example without comments
int result = a + b
- c;
return result;
// Example with comments
// Calculate the result based on the formula: result = a + (b
- c)
int result = a + b
- c;
// Return the calculated result
return result;
The example with comments clarifies the intent and formula, making it significantly more readable than the example without comments. This is just one example, but in complex scenarios, the impact of comments on understanding becomes even more profound.
Table of Comment Types
Comment Type | Example | Description |
---|---|---|
Single-line | // This is a single-line comment | Comments on a single line, used for brief explanations. |
Multi-line | /* This is a multi-line comment that spans multiple lines -/ |
Comments spanning multiple lines, suitable for detailed explanations. |
Documentation (Example: Java Javadoc) | / * This is a Javadoc comment. * It describes the method. * @param a The first parameter. * @return The calculated result. */ public int calculate(int a)… |
Comments specifically designed for generating API documentation, enhancing code understanding through structured explanations and parameters. |
Effective Comment Structure and Style
Writing clear and concise code comments is crucial for maintainability and collaboration. Well-structured comments enhance code readability, making it easier for developers to understand the logic behind code blocks and functions, even if they weren’t involved in the original development. This section details best practices for structuring comments, offering examples, and emphasizing the importance of consistency.
Comment Style Guide
A consistent style guide for comments promotes readability and reduces confusion. This involves adhering to specific formatting rules for indentation, spacing, and overall structure. A well-defined style guide ensures that comments are easily scannable and provide consistent information throughout the codebase.
Examples of Effective Comments
Here are examples demonstrating how to effectively document code blocks and functions.
- Function Documentation:
-
// Calculates the area of a rectangle. // Args: // length: The length of the rectangle. // width: The width of the rectangle. // Returns: // The area of the rectangle. function calculateRectangleArea(length, width) return length - width;
- Code Block Documentation:
-
// This block of code iterates through an array of numbers and squares each element. const numbers = [1, 2, 3, 4, 5]; const squaredNumbers = []; for (let i = 0; i < numbers.length; i++) const squared = numbers[i] - numbers[i]; squaredNumbers.push(squared);
Importance of Consistent Formatting
Using a consistent comment formatting style enhances readability and maintainability. It allows developers to quickly scan the code and understand its purpose without needing extensive explanation. This consistency minimizes confusion and promotes a unified code style across the entire project.
Commenting Complex Logic
Commenting complex logic requires a more detailed approach than simpler code blocks. Use multiple lines to break down complex operations into smaller, more manageable steps.
- Example of Commenting Complex Logic:
-
// This section calculates the discounted price of an item. // First, it checks if a discount is applicable based on the item's category. // If applicable, it applies the discount percentage to the original price. // Finally, it returns the discounted price. function calculateDiscountedPrice(item, discountPercentage) let discountedPrice = item.price; if (item.category === 'Electronics') discountedPrice -= (1 - discountPercentage / 100); return discountedPrice;
Indentation and Spacing in Comments
Proper indentation and spacing in comments enhance readability and maintain consistency. Align comments with the corresponding code block or function, ensuring that the comment structure mirrors the code's structure.
Best Practices Table
Formatting Rule | Example | Description |
---|---|---|
Indentation | //This is a well-formatted comment // that is properly indented. |
Indentation to match code structure |
Spacing | // This comment uses proper spacing. | Consistent spacing around text. |
Clarity | //Calculates the average of the numbers. | Use clear and concise language. |
Context | //This function handles user login. | Provide context for the code's role. |
Comment Content and Clarity
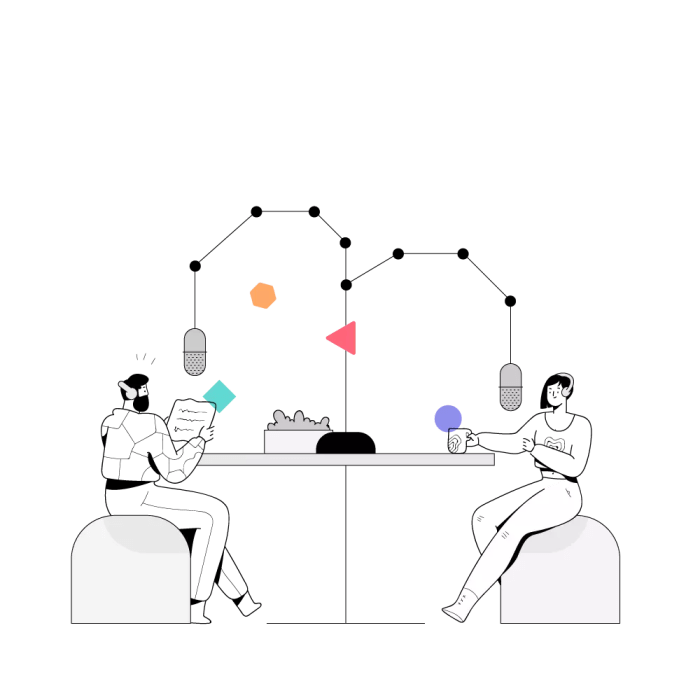
Source: sitepoint.com
Precise and descriptive language in comments is paramount for maintainability and understanding. Vague comments, while seemingly harmless, can lead to confusion and wasted time when revisiting the code. Clear comments should immediately convey the purpose and reasoning behind the code segment. This aids both the original author and other developers in comprehending the logic and design decisions.
Effective comments go beyond simple explanations; they articulate the
-why* behind the code, not just the
-what*. This deeper understanding promotes better collaboration and allows for more informed modifications and extensions. A clear comment acts as a concise summary, saving time and reducing the risk of misinterpretations.
Importance of Precise Language
Comments should be written with the same level of care and precision as the code itself. Avoid ambiguity and jargon. Use terms that are universally understood within the project's context. Substituting vague terms like "adjust" with specific terms like "scale the value by a factor of 2" significantly improves readability. This precision minimizes the potential for misinterpretations and promotes consistency in understanding.
Examples of Well-Written Comments
-
Good comment:
// Calculate the total price after applying a 10% discount.
-
Good comment:
// This loop iterates through all elements in the list, ensuring each element is processed sequentially.
-
Good comment:
// The function checks if the input string contains only digits. This is crucial for preventing errors in numerical processing.
Avoiding Vague or Ambiguous Comments
Vague comments often leave more questions than answers. They may contain overly general statements or use terms that lack specific meaning. Consider the following example:
// This part does something.
This comment offers no meaningful insight. A better approach would be to specify the action performed, such as:
// This part calculates the average of the values in the array.
Commenting on Design Choices and Trade-offs
Documenting design choices and the reasoning behind them is essential. Explaining the trade-offs made during development helps maintain the context of the code and allows for informed decisions in the future. Comments like these highlight the rationale behind certain design decisions:
// Using a hash table for this lookup is faster than a linear search, as it has a time complexity of O(1) on average. However, this comes at the cost of additional memory usage.
Example of Comments Requiring Improvement
Consider the following poorly written comment:
```java
// This section handles user input.
if (userInput.length() > 0)
//Do something with user input
```
This comment is overly general. A better comment would specify the nature of the input validation, or the intended action, like:
```java
// Validates that the user input is not empty. If not empty, process the input.
if (userInput.length() > 0)
// Process the input according to the specified logic.
```
This revised comment provides more context and clarity.
Comments for Different Code Elements
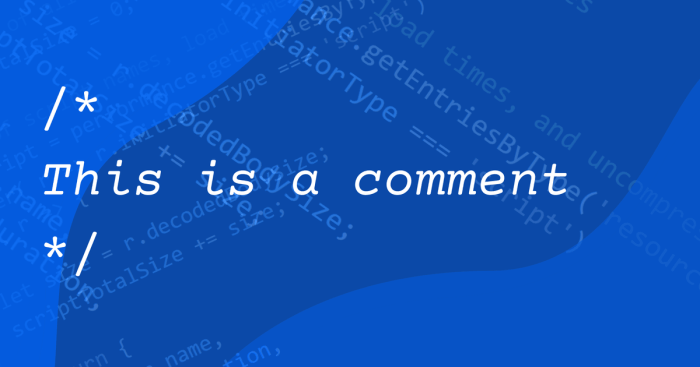
Source: openreplay.com
Effective code commenting goes beyond simply explaining what a piece of code does; it also considers the
-type* of code element being commented. Understanding the nuances of commenting variables, functions, classes, and methods leads to more maintainable and understandable codebases. This section details strategies for commenting different code elements, highlighting best practices for clarity and efficiency.
Commenting different code elements requires tailoring the approach to the specific element's purpose and scope. This allows for focused and concise explanations that improve the overall code's readability. Consistent commenting styles across a project further enhance the code's maintainability.
Commenting Variables
Variables store data, and comments should explain
-what* the data represents and
-why* it's used. Avoid simply restating the variable's type; instead, focus on its significance within the program's logic.
- Example: Instead of
// age of the user
, use// Stores the user's age in years.
- Consider context: A comment like
// user ID
might suffice if the variable is used consistently throughout the program. But for a variable only used in a specific function, a more detailed explanation might be needed.
Commenting Functions
Function comments should Artikel the function's purpose, inputs, and outputs. This includes explaining the function's expected behavior and any preconditions or postconditions.
- Example:
```java
/
* Calculates the area of a circle.
* @param radius The radius of the circle.
* @return The area of the circle.
* @throws IllegalArgumentException if radius is negative.
*/
double calculateCircleArea(double radius)
// ...function body ...
```
- Parameters, Return Values, and Exceptions: Explicitly document these elements for better understanding and error handling.
Commenting Classes
Class comments should describe the class's purpose, its key responsibilities, and the overall design. They provide a high-level overview of the class's functionality.
- Example:
```java
/
* Represents a customer in a store database.
* Contains information about the customer's name, ID, and purchase history.
*/
public class Customer
// ... class body ...```
- Focus on high-level design: Avoid diving into the implementation details within the class comment.
Commenting Methods
Method comments are similar to function comments, but focused on the method's specific task within the class. They should clarify the method's behavior, inputs, outputs, and potential exceptions.
- Example:
```java
/
* Retrieves the customer's purchase history.
* @param customerId The ID of the customer.
* @return A list of the customer's purchases.
* @throws CustomerNotFoundException if the customer ID is invalid.
*/
ListgetPurchaseHistory(int customerId)
// ...method body ...
```
- Relationship to Class: Method comments should clearly articulate how the method interacts with the class's other members and data.
Comparing Commenting Approaches
Different code structures benefit from varying levels of detail in comments. Variables often need brief, contextual explanations, while functions, classes, and methods require more comprehensive documentation, including parameters, return values, and exceptions.
- Consistency: Maintaining a consistent style for commenting across all code elements ensures readability and maintainability.
Comments for Complex Algorithms
For intricate algorithms, comments can break down complex logic into smaller, understandable steps. This approach helps in explaining the algorithm's purpose and how each step contributes to the overall solution.
- Example: A comment might explain the purpose of a loop, the criteria for a conditional statement, or the order of operations in a complex calculation.
Code Documentation and Comments
Comprehensive code documentation is crucial for maintainability and collaboration. Well-documented code is easier to understand, debug, and modify, especially for developers unfamiliar with the project. Thorough documentation significantly reduces the time and effort required to integrate new members or revisit old code.
Creating Comprehensive Code Documentation
Effective code documentation goes beyond simple comments; it's a structured approach. A good strategy involves combining comments within the code with separate, external documentation. This approach ensures that both immediate context and broader explanations are accessible. Consider using tools like Javadoc or Sphinx for automatically generating API documentation from comments, saving significant time and effort.
Effective API Documentation Using Comments
Clear and consistent API documentation is essential for external users or other developers interacting with a module. Comments should clearly describe the purpose, parameters, return values, and potential exceptions of each function or method. These comments should also ideally illustrate use cases with examples. Use a consistent style guide for comment formatting to maintain readability and reduce ambiguity.
Example (Javadoc-style):
/ * Calculates the sum of two integers. * * @param a The first integer. * @param b The second integer. * @return The sum of a and b. * @throws IllegalArgumentException if either a or b is negative. */ public int sum(int a, int b) if (a < 0 || b < 0) throw new IllegalArgumentException("Inputs cannot be negative."); return a + b;
Incorporating Comments for Other Developers
Comments should be targeted at explaining
-why* certain code decisions were made, rather than simply restating what the code does. Avoid redundant comments that simply mirror the code. Focus on providing context, rationale, or potential edge cases that might not be immediately apparent. This will help other developers understand the design choices and prevent misunderstandings.
Using comments to explain non-obvious algorithm choices or complex logic is especially helpful.
Updating Comments as Code Evolves
Maintaining accurate and up-to-date comments is crucial. As code evolves, comments must be updated to reflect those changes. Failing to keep comments current can lead to confusion and errors. Establish a process for reviewing and updating comments whenever code is modified.
Comments in Code Reviews and Collaboration
Comments play a vital role in code reviews and team collaboration. They provide opportunities for discussion and clarification of code decisions. Comments can facilitate constructive feedback and ensure that everyone is on the same page regarding the code's functionality and design. Detailed comments allow for efficient and effective discussions, fostering a shared understanding of the codebase.
Avoiding Common Commenting Mistakes
Effective code commenting is crucial for maintainability and collaboration. However, poorly written comments can detract from readability and even mislead developers. Understanding common pitfalls and how to avoid them is vital for creating comments that enhance, not hinder, code comprehension.
Inconsistent or redundant comments can be detrimental to code clarity. Comments should serve a specific purpose, providing context that is not readily apparent from the code itself. This section highlights key mistakes to avoid, emphasizing the importance of precise and purposeful commentary.
Redundant or Repetitive Comments
Redundant comments often repeat information already present in the code. They serve no additional purpose and clutter the codebase. For instance, a comment like "// variable x stores the user's age" alongside a variable declaration `int x = userAge;` is redundant. The code itself clearly defines the variable's purpose. Striking a balance between concise code and adequate explanation is essential.
Ambiguous or Unclear Comments
Comments should be unambiguous and easily understandable. Vague or overly general comments can be counterproductive. For example, a comment like "// this section does something" provides no useful information. The comment should describe
-what* the section does, not just that it does something. Precise and descriptive language is paramount for clarity.
Comments that Explain the Obvious
Avoid commenting on trivial or self-evident code. The code itself should be clear enough to be understood without further explanation. Comments should focus on non-obvious logic or implementation details, rather than summarizing the straightforward parts of the code.
Confusing Comments
Comments should not contradict the code. If a comment contradicts the code's functionality, it creates confusion and undermines the comment's value. Inaccurate or misleading comments can be more harmful than no comments at all. Always ensure that the comment accurately reflects the code's intent.
Lack of Contextual Comments
Sometimes comments lack the necessary context, making it difficult to understand the code's purpose. Without proper context, a comment may be correct in isolation but fail to explain the bigger picture. This can lead to misinterpretations and errors. Provide context that connects the comment to the surrounding code and overall functionality.
Commenting vs. Explaining Code
Comments should supplement, not replace, well-structured and readable code. Focus on making the code itself as clear as possible through good variable naming, function design, and code organization. Comments are best used to explain the
-why* behind the code, not the
-what*. Commenting should only be used when the code itself cannot effectively communicate its purpose.
Comments and Maintainability
Comments play a critical role in code maintainability. Clear, concise comments allow developers to easily understand and modify code over time. Well-maintained code, with useful comments, is easier to modify and debug, ensuring the codebase remains robust and scalable.
Advanced Commenting Techniques: How To Comment Code Effectively
Effective code comments go beyond basic explanations. Advanced techniques leverage comments to enhance comprehension, maintainability, and collaboration. These techniques address complex concepts, promote code reuse, and aid in version control. They are crucial for professional-level codebases.
By incorporating these techniques, developers can create code that is not only functional but also easily understood and modified by others, and even themselves, in the future. This contributes significantly to the overall success of software projects.
Code Examples within Comments
Comments can contain concise code examples to illustrate specific functionalities or alternative approaches. This allows readers to immediately see how a particular piece of code behaves in practice, making the explanation more concrete and easier to grasp. For instance, if a comment describes a complex algorithm, a small, illustrative example embedded within the comment can dramatically improve understanding.
Code Snippets for Complex Concepts
Illustrating complex concepts with code snippets is a powerful way to provide more in-depth explanations. Rather than just describing a complex function or algorithm, the comment can contain a complete, runnable snippet of code that demonstrates the concept's usage. This is particularly useful for functions or classes with multiple parameters or intricate logic.
External Resource Links in Comments
Incorporating links to external resources within comments is beneficial for expanding on the context of a piece of code. If a particular function relies on a third-party library or a specific algorithm, including a link to the documentation or relevant research can significantly improve understanding. This approach allows readers to quickly delve deeper into the details without interrupting the flow of the comment.
Multi-Language Commenting, How to comment code effectively
In projects that utilize multiple programming languages, comments can be written in a format that accommodates the different languages. For example, in a project using both Python and JavaScript, a comment could use Python-style syntax for explaining a Python-specific function and JavaScript-style syntax for JavaScript functions.
Comments and Version Control
Comments play a vital role in supporting version control systems. Well-maintained comments can serve as a historical record of changes and decisions made during the development process. This can be especially valuable for understanding why certain design choices were made or how the code evolved over time. The comments can act as a detailed log, facilitating easier tracking of code modifications.
For example, a comment can document the reason for a particular change or improvement implemented in a specific version of the code.
Final Review
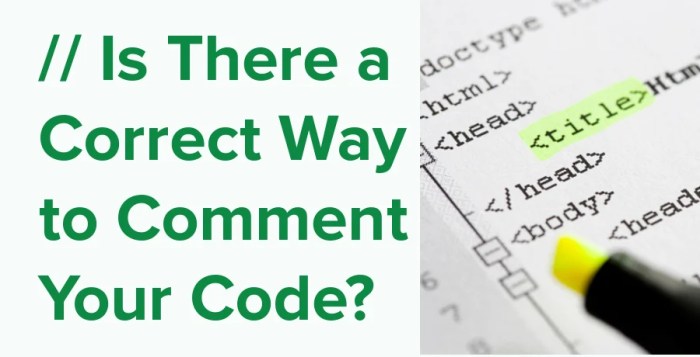
Source: ndepend.com
In conclusion, effective code commenting is a fundamental skill for any developer. By understanding the purpose, structure, and content of comments, you can significantly improve code maintainability and collaboration. This guide has provided a framework for creating clear, concise, and helpful comments across various code elements. Remember to prioritize clarity, consistency, and precision in your comments, and continuously refine your commenting approach as your projects evolve.
Post Comment