How To Prepare Coding Interview
How to prepare coding interview covers the essential steps to excel in technical interviews. This guide delves into the structure of coding interviews, from understanding common question types to mastering essential problem-solving techniques. It equips you with the strategies and resources needed to confidently tackle coding challenges and land your dream job.
This comprehensive resource details the crucial elements of coding interview preparation, including analyzing interview structure, understanding different question types (algorithm, data structure, system design), and employing effective problem-solving strategies. It also highlights the importance of consistent practice, using various online platforms and personal projects, and the value of focusing on underlying logic.
Understanding Coding Interview Structure
Coding interviews are designed to assess a candidate’s problem-solving skills, technical knowledge, and ability to adapt to new challenges. A structured approach to understanding the typical stages and types of questions asked is crucial for effective preparation. This section provides a comprehensive overview of the coding interview process.The interview process typically involves multiple stages, each designed to evaluate different aspects of the candidate’s abilities.
These stages can range from initial screening calls to final technical interviews with senior engineers. Successful navigation of each stage requires a well-rounded understanding of the candidate’s strengths and weaknesses in different technical areas.
Typical Stages of a Coding Interview
A typical coding interview sequence usually begins with an initial phone screen, followed by one or more technical interviews. These technical interviews often include coding challenges, system design questions, and behavioral questions. The final stage, if applicable, might involve a panel interview with senior engineers or a final coding round.
Common Types of Coding Interview Questions
Coding interviews encompass a wide range of question types, each designed to assess specific skills. Algorithm questions focus on efficiency, data structure questions evaluate knowledge of data structures, and system design questions gauge the ability to design and scale systems. The combination of these types provides a comprehensive assessment of the candidate’s capabilities.
Importance of Problem-Solving Strategies
A critical aspect of success in coding interviews is mastering problem-solving strategies. Approaches like breaking down complex problems into smaller, more manageable sub-problems, using appropriate data structures, and focusing on efficiency are key to achieving a successful outcome. This systematic approach allows candidates to methodically analyze and tackle the challenges posed.
Examples of Coding Interview Questions
To illustrate the different types of questions, here are examples across various programming languages:
- Algorithm (Python): Given an array of integers, find the largest sum of a contiguous subarray. This question assesses the candidate’s understanding of efficient algorithms like Kadane’s algorithm.
- Data Structure (Java): Implement a stack data structure. This question probes the candidate’s ability to implement a fundamental data structure from scratch.
- System Design (C++): Design a scalable system for a social media platform. This type of question evaluates the candidate’s capacity to consider factors like scalability, performance, and security.
Comparison of Question Types
The table below compares and contrasts the different types of coding interview questions:
Question Type | Description | Example Question | Programming Language | Difficulty Level |
---|---|---|---|---|
Algorithm | Focuses on efficient problem-solving techniques. | Given an array of integers, find the largest sum of a contiguous subarray. | Python | Medium |
Data Structure | Tests understanding of data structures. | Implement a queue data structure. | Java | Medium |
System Design | Assess ability to design large-scale systems. | Design a scalable system for a video streaming platform. | C++ | Hard |
Essential Preparation Strategies: How To Prepare Coding Interview
A strong foundation in coding interview preparation involves a multifaceted approach, encompassing consistent practice, a deep understanding of underlying logic, and strategic planning. Successful candidates consistently apply these principles, tailoring their approach to their individual strengths and weaknesses. This section delves into the core strategies for effective coding interview preparation.Effective coding interview preparation requires more than just memorizing algorithms.
It demands a proactive and structured approach that prioritizes consistent practice, understanding, and targeted strategies. Candidates who successfully navigate these interviews demonstrate a mastery of fundamental concepts, coupled with the ability to apply them to novel problems.
Creating a Comprehensive Practice Plan
A well-defined practice plan is crucial for success in coding interviews. It should Artikel specific goals, timelines, and the methods used to achieve them. A plan that adapts to your learning pace and incorporates consistent practice is essential.
Regular Coding Problem Practice, How to prepare coding interview
Regular practice is paramount in mastering coding interview techniques. Practicing coding problems not only enhances your problem-solving skills but also familiarizes you with the types of questions you might encounter in interviews. This familiarity builds confidence and reduces anxiety on the day of the interview.
Methods for Practicing Coding Problems
Numerous methods can be employed to hone your coding skills. Online platforms offer a wealth of practice problems, and personal projects provide valuable experience in tackling real-world challenges. Mock interviews simulate the interview environment, allowing you to refine your approach and manage time effectively.
Understanding Underlying Logic
Focusing on understanding the underlying logic behind solutions is vital. Simply memorizing solutions without comprehending the reasoning behind them will not lead to long-term improvement. Understanding the logic empowers you to adapt your approach to similar problems and troubleshoot issues more effectively.
Developing a Study Schedule
A structured study schedule, considering your learning pace and time constraints, is crucial. Break down your preparation into manageable chunks, ensuring consistent effort throughout the process. A realistic schedule will help you stay motivated and avoid burnout. Consider allocating dedicated time slots for practice, review, and problem-solving.
Problem-Solving Patterns
Identifying and practicing problem-solving patterns can significantly enhance your efficiency. Recognizing recurring patterns in coding problems allows you to develop templates for solutions, leading to quicker and more organized approaches.
Coding Practice Platforms
Various online platforms provide valuable resources for coding practice. Each platform has its own strengths and caters to different learning styles.
Platform | Strengths | Target Audience |
---|---|---|
LeetCode | Extensive question bank, ranked problems, detailed solutions | Beginners to Advanced |
HackerRank | Diverse problem sets, coding challenges, competitive environment | Beginners to Advanced |
Codewars | Focus on challenges, coding kata, community interaction | Beginners to Advanced |
Mastering Data Structures and Algorithms
A strong understanding of data structures and algorithms is crucial for success in coding interviews. These fundamental concepts underpin many programming tasks, and interviewers often assess your ability to apply them efficiently and effectively. This section will delve into key data structures, common algorithms, and their real-world applications, emphasizing the importance of time and space complexity analysis.
Key Data Structures
Data structures are specialized formats for organizing, processing, retrieving, and storing data. They are fundamental to efficient algorithm design. Understanding the characteristics of different data structures allows you to choose the most appropriate one for a specific problem.
- Arrays: Arrays are linear collections of elements of the same data type, accessed by their index. They offer fast random access but are less flexible for dynamic resizing. Consider using arrays when you know the size of the data beforehand and need to access elements quickly.
- Linked Lists: Linked lists store elements in nodes connected by pointers. Each node holds data and a reference to the next node. They are more flexible for dynamic resizing but offer slower random access compared to arrays. Linked lists are suitable when you anticipate frequent insertions or deletions.
- Trees: Trees are hierarchical data structures with a root node and branches extending downward. They are useful for representing hierarchical relationships and enabling efficient search and traversal. Binary trees, for instance, have a maximum of two children per node, making them suitable for various algorithms.
- Graphs: Graphs represent relationships between objects (nodes) using edges. They are valuable for modeling networks, social interactions, and various real-world scenarios where relationships are crucial. Understanding graph traversal algorithms is essential for coding interview questions involving graph manipulation.
Common Algorithms
Algorithms are step-by-step procedures for solving specific problems. Their efficiency is often measured by their time and space complexity. Knowing common algorithms allows you to apply them effectively in various scenarios.
- Sorting Algorithms: Sorting algorithms arrange elements in a specific order (ascending or descending). Examples include bubble sort, merge sort, quick sort, and insertion sort. The choice of algorithm depends on the size of the data and the desired performance characteristics.
- Searching Algorithms: Searching algorithms locate a specific element within a data structure. Linear search checks each element sequentially, while binary search is more efficient on sorted data, halving the search space with each step. The optimal choice depends on whether the data is sorted and the size of the data set.
- Dynamic Programming: Dynamic programming breaks down complex problems into smaller, overlapping subproblems and stores the solutions to avoid redundant calculations. It’s particularly effective for optimization problems and finding the shortest path, for instance, in a network.
Real-World Applications
Data structures and algorithms are fundamental to numerous real-world applications. From social media platforms that use graphs to manage user relationships to e-commerce websites that utilize sorting algorithms for product recommendations, these concepts are vital.
- Databases: Databases rely heavily on data structures like trees and hash tables to efficiently store and retrieve data. Efficient query processing relies on well-designed algorithms.
- Operating Systems: Operating systems use algorithms for tasks like scheduling processes, managing memory, and file system management.
- Machine Learning: Machine learning algorithms often involve sophisticated data structures and algorithms to train models and make predictions.
Time and Space Complexity Analysis
Time complexity measures the execution time of an algorithm, while space complexity quantifies the memory used. Analyzing these complexities is essential for selecting efficient algorithms for a given problem.
- Understanding Big O Notation: Big O notation provides a way to express time and space complexity in terms of input size (n). This allows for comparing the efficiency of algorithms as the input grows. Common notations include O(1), O(log n), O(n), O(n log n), O(n^2), and O(2^n).
- Choosing the Right Algorithm: The choice of algorithm depends on the specific needs of the problem. Consider the time and space complexity of different algorithms to select the most efficient one for a given input size.
Sorting Algorithm Comparison
Choosing the appropriate sorting algorithm depends on factors like the size of the dataset and the required performance characteristics.
Algorithm | Time Complexity (Best Case) | Time Complexity (Average Case) | Time Complexity (Worst Case) | Space Complexity |
---|---|---|---|---|
Bubble Sort | O(n) | O(n^2) | O(n^2) | O(1) |
Merge Sort | O(n log n) | O(n log n) | O(n log n) | O(n) |
Effective Problem-Solving Techniques
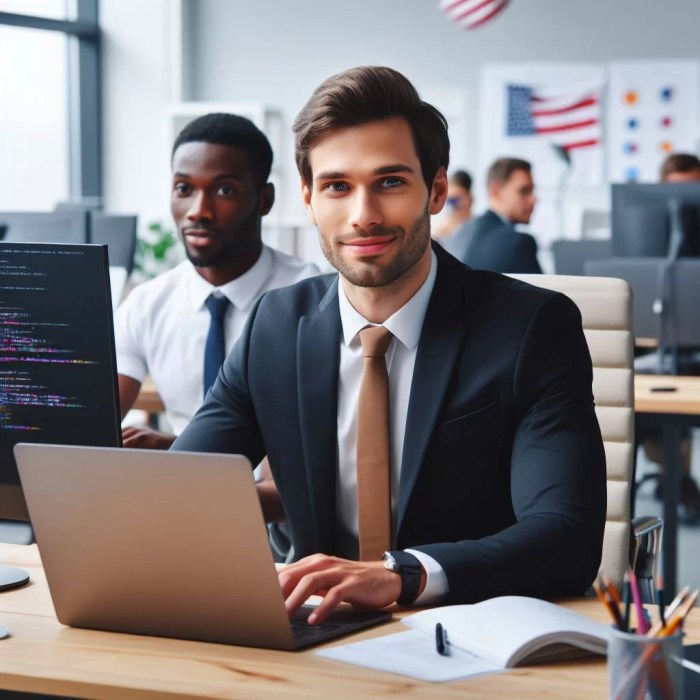
Source: learncodingusa.com
Successfully navigating coding interview problems hinges on more than just technical proficiency. A structured approach to problem-solving, coupled with effective debugging strategies, significantly improves your chances of success. This section Artikels key techniques to approach coding interview problems effectively.A strong problem-solving approach involves breaking down complex problems into smaller, more manageable components. This allows for a systematic understanding and implementation of solutions, minimizing the risk of overlooking crucial details.
By understanding the underlying structure of problems and identifying patterns, you can design efficient and robust algorithms.
Approaching Coding Interview Problems
A systematic approach to problem analysis is crucial for success. Begin by thoroughly understanding the problem statement, paying close attention to the inputs, outputs, and any constraints. Sketching out potential scenarios and edge cases helps clarify the requirements and identify potential challenges. Once the problem is well-defined, consider possible solutions, focusing on efficiency and correctness.
Breaking Down Complex Problems
Decomposing complex problems into smaller, more manageable sub-problems is a cornerstone of effective problem-solving. This decomposition process often involves identifying the key components of the problem and isolating them for independent consideration. This iterative approach allows you to focus on individual parts, ensuring that each component is well-understood before integrating them into a complete solution.
Identifying Patterns and Relationships
Recognizing patterns and relationships in problems is essential for designing efficient solutions. Experience with various problem types will help you develop an intuition for identifying recurring themes. Look for common patterns in input data, relationships between variables, or the structure of the problem itself. This pattern recognition can significantly reduce the time required for developing a solution.
Pseudo-coding and Solution Design
Pseudo-coding, or outlining the solution in plain language before writing actual code, significantly improves the clarity and efficiency of your solutions. This pre-implementation step allows for critical thinking and identification of potential pitfalls, often leading to a more elegant and optimized solution. Design your solution carefully, considering different approaches and potential performance implications.
Debugging Techniques
Effective debugging is an integral part of the coding process. A methodical approach is critical for identifying and resolving errors in code. This involves analyzing the code for logical errors, examining input and output values, and using debugging tools to step through the code and identify problematic lines. Employing print statements or logging can assist in understanding the flow of execution and identifying the source of issues.
Handling Time Constraints
Time constraints are a common challenge in coding interviews. A strategic approach is crucial. Prioritize understanding the problem and identifying the most efficient solution before diving into implementation. Avoid getting bogged down in minor details, and focus on the core logic of the solution. If you encounter roadblocks, don’t hesitate to ask clarifying questions.
Handling Unexpected Input
Coding interviews often test your ability to handle various input scenarios, including edge cases and invalid data. Explicitly consider the range of potential inputs and devise strategies to handle unexpected input values. Input validation is critical to prevent errors and ensure robustness. For instance, checking for null values, empty strings, or values outside the specified range is crucial.
Mock Interviews and Feedback
Mock interviews are crucial for honing your coding interview skills. They provide a realistic simulation of the interview process, allowing you to practice under pressure and receive valuable feedback on your performance. This practice is essential for identifying and addressing weaknesses before the actual interview.Effective preparation for mock interviews involves understanding the interview structure, reviewing common coding interview questions, and practicing your problem-solving approach.
Active participation and a willingness to receive and implement feedback are vital components of this process.
Importance of Mock Interviews
Mock interviews provide a safe environment to practice coding under pressure. They offer an opportunity to address weaknesses and refine your approach to solving problems, which are critical for success in real interviews. The feedback you receive is invaluable in pinpointing areas requiring improvement, such as clarity of thought, code efficiency, or time management.
Preparing for Mock Interviews
Thorough preparation is key to maximizing the benefits of mock interviews. Review common interview questions and practice coding problems in advance. Familiarize yourself with the tools and platforms that will be used during the interview. Be prepared to discuss your thought process and explain your reasoning clearly. Focus on expressing your understanding of the problem and the solution’s logic clearly and concisely.
Being prepared with relevant examples of your past projects will strengthen your confidence and allow you to articulate your abilities more effectively.
Utilizing Feedback Effectively
Feedback from mock interviews is a powerful tool for improvement. Actively listen to the feedback provided, and analyze the specific areas where you need to strengthen your skills. Don’t just accept the feedback; critically assess its validity. Determine whether the suggestions are constructive and actionable. Take notes on the feedback and create a plan to address the areas identified.
Consider implementing the feedback immediately, perhaps by reviewing past mistakes and understanding their root causes.
Recording and Reviewing Mock Interviews
Recording your mock interviews allows for a detailed review and analysis of your performance. This enables you to identify areas for improvement, such as clarity in communication, efficient coding practices, or time management strategies. Reviewing the recording helps you observe your body language, vocal tone, and confidence level. Pay attention to how you articulate your solutions and address challenges.
Reviewing recorded interviews allows you to pinpoint areas where you may be lagging and to actively address those shortcomings.
Common Mistakes in Coding Interviews and How to Avoid Them
Understanding common coding interview mistakes can help you avoid repeating them. A structured approach to identifying and resolving these issues will greatly increase your chances of success.
Mistake | Explanation | How to Avoid |
---|---|---|
Incorrect data structure selection | Using an inappropriate data structure for the problem, leading to inefficient solutions. | Carefully analyze the problem requirements and choose the optimal data structure based on the operations needed (e.g., searching, sorting, insertion, deletion). |
Inefficient algorithm selection | Using an algorithm with high time/space complexity, resulting in a slow or memory-intensive solution. | Understand different algorithms and their complexities (e.g., time and space). Consider the potential impact of different algorithms on the performance of your solution. |
Incorrect time complexity analysis | Failing to accurately analyze the time complexity of your solution, leading to an inaccurate assessment of its performance. | Thoroughly understand the time complexity of different operations and algorithms. Practice analyzing the time complexity of your solutions before implementing them. |
Summary
In conclusion, mastering coding interviews requires a multifaceted approach. By understanding the interview structure, practicing consistently, and focusing on problem-solving techniques, you can significantly enhance your chances of success. This guide provides a roadmap to prepare effectively, equipping you with the necessary knowledge and skills to ace your coding interview.
Post Comment