Top Projects Beginner Programmers
Top projects beginner programmers are crucial for solidifying foundational programming skills. This guide explores various beginner-friendly projects, from simple text-based games to web applications, helping you choose and execute them effectively. We’ll delve into project selection criteria, design best practices, and crucial learning resources, empowering you to embark on your programming journey with confidence.
Understanding the importance of project scope and complexity is key. Beginner projects should focus on fundamental concepts rather than overwhelming complexity. We’ll examine examples in different programming languages, like Python and JavaScript, demonstrating how to structure projects for efficiency and maintainability. A structured approach is vital, and we’ll cover the essential steps to develop, test, and deploy these projects successfully.
Introduction to Beginner Programming Projects
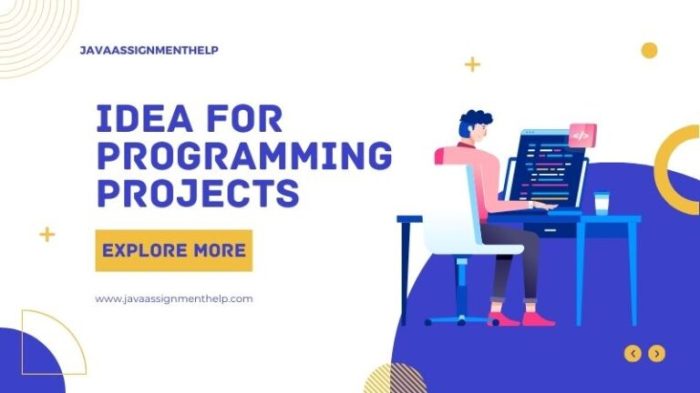
Source: javaassignmenthelp.com
A “top” beginner programming project is one that effectively introduces fundamental programming concepts while being engaging and achievable. These projects often involve building something tangible, allowing learners to see the practical application of their knowledge. They’re designed to build confidence and a sense of accomplishment, which is crucial for maintaining motivation in the early stages of learning.Beginner projects are essential for solidifying programming fundamentals.
They provide a hands-on environment where learners can experiment with different concepts and develop problem-solving skills. Through these projects, learners build a solid foundation for more complex programming tasks in the future. The initial projects are like building blocks; the stronger the foundation, the more complex structures you can create later.
Defining Characteristics of Good Beginner Projects
Good beginner projects exhibit several key characteristics. They should be relatively straightforward to understand and implement. The project’s scope should be manageable within the time constraints of a learner. Crucially, they should allow for gradual progression and complexity, enabling learners to build upon their initial understanding. This incremental learning is critical for building a robust knowledge base.
Types of Beginner Programming Projects
Different project types offer varied learning experiences for beginners. Understanding the strengths of each type can help learners select a project that aligns with their interests and learning style.
Project Type | Focus | Example | Learning Outcomes |
---|---|---|---|
Game Development | Logic, control structures, user interaction | Simple text-based adventure game, or a basic platformer | Developing conditional logic, loops, functions, and event handling. |
Web Development | HTML, CSS, JavaScript, basic front-end design | A personal website or a simple web application with forms | Learning about web technologies, creating user interfaces, handling user input. |
Data Analysis | Data manipulation, visualization, basic statistics | Analyzing a dataset of personal information or tracking user statistics | Learning how to read, clean, and manipulate data, creating charts and graphs. |
Example Project: A Simple Text-Based Game
A simple text-based adventure game is an excellent choice for beginners. It introduces fundamental concepts like conditional statements, loops, and user input. By creating a game, learners can apply programming concepts in a fun and engaging way. For instance, a simple text-based adventure game could prompt the user with choices, leading to different outcomes based on the user’s input.
This encourages the learner to think through different scenarios and code accordingly.
Project Selection Criteria
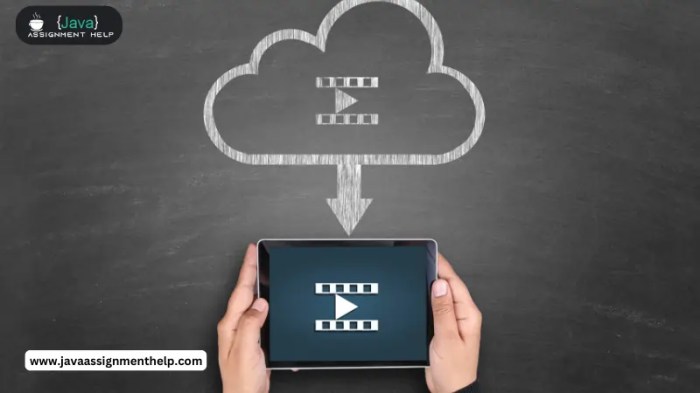
Source: javaassignmenthelp.com
Choosing the right project is crucial for a beginner programmer’s learning journey. Selecting projects that are appropriately challenging yet achievable fosters a sense of accomplishment and encourages continued engagement. A well-structured project also provides a practical application of learned concepts, reinforcing understanding and building confidence.Selecting projects that align with a beginner’s skillset and interests is vital. Projects should be motivating and encourage persistence, rather than discouraging the learner.
Careful consideration of project scope, complexity, structure, and documentation is essential for a rewarding and effective learning experience.
Project Scope and Complexity
The scope of a project, encompassing the features and functionalities to be implemented, significantly impacts its suitability for beginners. A project with an overly broad scope might be overwhelming, while one that’s too narrow might not offer sufficient opportunities for learning. Careful consideration of the project’s features is essential to ensuring the project is appropriately sized for a beginner.
Striking a balance between complexity and comprehensibility is vital. A project’s complexity should match the learner’s current skill level, allowing for a sense of accomplishment without becoming frustrating.
Project Structure and Modularity
A well-structured project is easily understandable and maintainable. Projects should be divided into smaller, manageable modules. This modularity promotes organization and facilitates the understanding of different components of the project. It allows for incremental development and helps in debugging, as issues are localized to specific modules. This structured approach makes the code more understandable and adaptable, allowing for potential future enhancements.
Beginner-Friendly Project Ideas
Projects should be relevant to a beginner’s interests. Here are some example ideas categorized by programming language:
- Python: Simple text-based games (e.g., number guessing game, hangman), basic data analysis tasks (e.g., analyzing a dataset to find trends), or creating a simple to-do list application.
- JavaScript: Interactive web page elements (e.g., creating a dynamic form or interactive quiz), or basic web applications (e.g., a simple calculator or a basic blog). A JavaScript project might also involve creating a simple animation or game using a library like p5.js.
- Java: Simple console-based applications (e.g., a basic calculator or a simple inventory management system), or small desktop applications using JavaFX.
Programming Language Suitability
Python is often considered beginner-friendly due to its clear syntax and extensive libraries. JavaScript is well-suited for front-end web development, offering opportunities to create interactive web pages. Java is a robust language suitable for developing applications with more complex requirements.
Clear Project Documentation
Clear project documentation is essential. This includes a description of the project’s goals, functionalities, and design decisions. It also includes details about the technologies used and the steps required for the project to run successfully. Well-documented projects facilitate understanding, collaboration, and maintenance.
Project Categories and Example Languages
Project Category | Example Languages |
---|---|
Text-based Games | Python, JavaScript |
Web Applications | JavaScript |
Data Analysis | Python |
Simple Desktop Applications | Java |
Project Examples and Ideas
Getting started with programming can be exciting, but choosing the right project can make all the difference. This section offers a range of beginner-friendly project ideas, categorized for clarity. These examples cover fundamental programming concepts, gradually increasing in complexity, making it easier for novices to grasp the essence of programming.Choosing a project that aligns with your interests and learning objectives is key to maintaining motivation and achieving a rewarding learning experience.
Starting with smaller projects and gradually increasing the complexity will help build your confidence and programming skills.
Example Beginner Projects
These projects are designed to build foundational programming skills. They introduce core concepts like variables, loops, and conditional statements.
- A simple calculator: This project allows you to practice basic arithmetic operations. You can extend this to include more complex calculations, like square roots or percentages, as your skills improve.
- A to-do list application: This project teaches you how to manage data, store tasks, and organize them. You can add features like marking tasks as complete or setting deadlines as you become more comfortable.
- A basic text-based game (like a number guessing game or a simple adventure game): These games help you understand how to handle user input and control program flow. This can be an excellent starting point to introduce decision-making logic and interactivity.
Projects Involving Basic Algorithms
These projects delve into fundamental algorithmic concepts, introducing you to problem-solving strategies.
- Sorting a list of numbers: This is a classic algorithm project. You can experiment with different sorting algorithms (like bubble sort, insertion sort, or selection sort) and compare their efficiency.
- Searching for an element in a list: Implement search algorithms like linear search and binary search to practice finding specific data within a collection. This will illustrate how algorithm choice affects performance.
- Finding the greatest common divisor (GCD) of two numbers: This project involves applying mathematical concepts to develop a program that efficiently calculates the GCD.
Projects Focused on Data Structures
These projects introduce fundamental data structures, laying the groundwork for more complex programming endeavors.
- Implementing a linked list: This project demonstrates how to create and manipulate a linked list data structure. This project will illustrate how linked lists differ from arrays in terms of memory management and access patterns.
- Creating a stack or queue: A stack or queue is a specific way of organizing data. Implementing these structures helps you understand how to manage data in a last-in, first-out (LIFO) or first-in, first-out (FIFO) manner. These are crucial data structures in many algorithms and applications.
Projects with Simple User Interfaces
These projects introduce the concept of user interaction and input.
- A simple text-based menu-driven program: This project introduces the concept of presenting options to the user and handling their choices. This demonstrates the interaction between the user and the program.
- A basic graphical user interface (GUI) application (using a library like Tkinter): This project will allow you to create a more visually appealing program with buttons, text boxes, and other interactive elements.
Projects Using External APIs
These projects demonstrate how to access and use external data sources.
- A weather application: This project involves fetching weather data from an external API and displaying it to the user. This demonstrates how to make API calls and process external data.
- A currency converter: This project involves fetching currency exchange rates from an API and providing conversion information to the user. This will introduce the concept of fetching data from external resources.
Projects Integrating with Databases
These projects involve storing and retrieving data persistently.
- A simple address book: This project teaches you how to store and retrieve contact information using a database (like SQLite). This shows how to create and query a database.
- A simple inventory management system: This project teaches you how to store and manage inventory items using a database. This demonstrates data persistence and retrieval.
Project Complexity and Estimated Development Time
| Project Category | Complexity Level | Estimated Development Time (Hours) ||—|—|—|| Simple Calculator | Low | 5-10 || To-Do List | Medium | 10-20 || Basic Text-Based Game | Medium | 15-30 || Sorting Algorithm | Medium | 10-20 || Linked List Implementation | Medium-High | 20-40 || Weather Application | High | 25-50 || Simple Database Application | High | 30-60 |
Detailed Example: A Simple Text-Based Adventure Game
This example Artikels a simple text-based adventure game.“`#include A well-structured project is crucial for managing complexity and ensuring maintainability, especially as projects grow. Proper structuring allows for easier collaboration, debugging, and future modifications. Careful planning at the outset significantly reduces frustration and wasted time later on.A well-organized project also promotes a more efficient workflow, allowing programmers to focus on logic and functionality rather than getting lost in the intricacies of file organization. This focus enhances the overall coding experience. Clear project structure is vital for maintainability and collaboration. Use a hierarchical directory structure mirroring the project’s components. This improves navigation and reduces confusion. Separate data files from code files. This separation enhances code organization. Breaking down projects into smaller, well-defined tasks simplifies the development process. This approach allows for a better understanding of the project’s scope and helps to track progress. Using task management tools (or even a simple notebook) can assist in planning and organizing these tasks. Employing consistent coding styles and conventions is essential for readability and maintainability. Use meaningful variable names and comments to explain complex logic. Adhere to established coding standards to promote uniformity and ease of comprehension. A well-structured web application involves several key steps. Employing version control systems, like Git, from the beginning fosters a collaborative and organized development environment. This approach is valuable for tracking changes, managing different versions, and collaborating with others. Git’s branching capabilities are invaluable for exploring different ideas and solutions. A simple calculator application demonstrates effective project structuring.“`calculator/├── src/│ ├── calculator.py # Contains the calculator logic│ ├── utils.py # Contains utility functions│ └── __init__.py # Empty file for package structure├── data/│ └── config.json # Configuration file (optional)├── tests/│ └── test_calculator.py # Test cases for the calculator└── README.md“` Object-oriented programming (OOP) can enhance the structure and maintainability of beginner projects. Representing program components as objects promotes code modularity and reusability. Beginners can start by creating simple classes to encapsulate data and methods. Navigating the world of beginner programming can feel daunting. Fortunately, a wealth of resources and supportive communities are available to help you overcome challenges and thrive. This section Artikels valuable tools and strategies to aid your journey.Learning programming is a process of continuous exploration and refinement. Effective learning hinges on accessing reliable information, interacting with supportive communities, and leveraging practical tools. This section equips you with the necessary knowledge and resources to foster a robust learning experience. Numerous websites and platforms offer introductory programming resources. These resources often include project-based learning, allowing you to apply concepts while building something tangible. Online courses, tutorials, and documentation are excellent sources for understanding programming languages and project structures. Engaging with other programmers is crucial for learning and troubleshooting. Online communities and forums offer a supportive environment to ask questions, receive feedback, and share your progress. Comprehensive documentation and tutorials are readily available for various beginner projects. Understanding the specific documentation associated with each project greatly improves the learning process. Libraries and frameworks streamline the development process, allowing you to focus on the core logic of your project. They offer pre-built components and functionalities that can significantly accelerate project development. Mentorship plays a crucial role in guiding beginners through the complexities of programming. Experienced programmers can offer valuable insights, address challenges, and provide constructive feedback. A summary of popular online learning platforms and their project-related resources: Implementing a programming project involves translating the design into working code. This process, crucial for any project, requires meticulous attention to detail, adherence to established programming principles, and effective testing methodologies. Successful project implementation hinges on not only producing functional code but also on verifying its accuracy and robustness through comprehensive testing.The key to effective project implementation is a well-defined strategy. This includes a clear understanding of the project requirements, the chosen programming language, and the appropriate tools and libraries. The testing phase is equally important, as it ensures the project functions as intended and identifies any potential issues before deployment. The implementation process generally follows a sequence of steps. First, a comprehensive understanding of the project’s logic is essential. This includes careful consideration of the data structures and algorithms required. Second, code is written incrementally, ensuring each part functions correctly before moving on. Third, modular design is recommended, breaking down the project into smaller, manageable modules. Fourth, thorough testing is carried out at each stage. Testing is crucial for verifying the correctness and reliability of a project. Beginner projects, in particular, benefit from a structured approach to testing. Unit testing isolates individual units (functions or classes) to verify their functionality. Integration testing verifies how different units interact and work together. Debugging and error handling are essential components of any project. Debugging involves identifying and resolving errors within the code. Robust error handling anticipates potential issues and provides graceful exits or alternative solutions when errors occur. This prevents unexpected program crashes and enhances user experience. “Error handling should not only identify errors but also provide informative messages and appropriate responses to prevent further complications. Clear error messages guide the user or developer to resolve the issue effectively.” Source: medium.com Deploying and sharing your projects is a crucial step in the programming journey. It allows you to showcase your work, receive feedback, and potentially contribute to the broader programming community. This section details various deployment methods and emphasizes the importance of code quality and sharing practices.Deployment methods for beginner projects often depend on the project type. Simple projects can be shared directly, while more complex ones might need more sophisticated approaches. Understanding the appropriate deployment strategy is vital for effectively showcasing your skills. Several methods are available for deploying beginner projects, catering to different project complexities and intended audiences. Direct sharing of files, online repositories, and simple web applications are common approaches. Project Structure and Design
Best Practices for Project Structuring
Breaking Down Projects into Manageable Tasks
Creating Well-Organized Code
Designing a Basic Web Application
Step
Description
1. Define Requirements
Clearly Artikel the functionality and features of the application.
2. Design the User Interface (UI)
Create a visual representation of how users will interact with the application.
3. Design the Database (if applicable)
Structure the data storage mechanism.
4. Choose Technologies
Select programming languages, frameworks, and libraries to implement the application.
5. Implement the Front-end
Develop the user interface elements.
6. Implement the Back-end (if applicable)
Develop the server-side logic.
7. Test and Debug
Thoroughly test all functionalities to identify and fix bugs.
8. Deployment
Publish the application to a hosting platform.
Version Control Systems for Beginner Projects
Example Project File Structure (Simple Calculator)
Object-Oriented Programming in Beginner Projects
Learning Resources and Support
Reliable Resources for Learning Beginner Projects
Online Communities and Forums for Beginners
Online Tutorials and Documentation for Various Projects
Using Online Libraries and Frameworks for Project Development
Mentors and Experienced Programmers
Online Learning Platforms with Project Resources
Platform
Project Resources
Codecademy
Interactive lessons, project-based exercises, and community forums
freeCodeCamp
Open-source projects, guided tutorials, and a supportive community
Khan Academy
Interactive exercises, lessons, and practice problems covering various programming concepts
Coursera
Structured courses with project-based assignments and often expert instructors
edX
Project-based courses, peer-to-peer learning opportunities, and often guest lectures from industry professionals
Project Implementation and Testing
Step-by-Step Implementation Process
Testing Methodologies for Beginner Projects
Importance of Debugging and Error Handling
Common Beginner Programming Errors and Troubleshooting, Top projects beginner programmers
Different Approaches to Testing
Project Deployment and Sharing
Different Deployment Methods
It also helps build a portfolio and provides an easily accessible platform for sharing your work.
Code Quality and Maintainability
High-quality code is crucial for any project, especially for beginners. Clean, well-documented code is easier to understand, maintain, and debug, even if the project’s scope is small. Good coding practices contribute significantly to the project’s success.
- Meaningful Variable Names: Use names that clearly indicate the purpose of variables, functions, and classes. This improves readability and maintainability. Example: Using ‘customerName’ instead of ‘cName’ improves understanding.
- Comments: Include comments to explain complex logic or non-obvious parts of the code. Clear and concise comments enhance the code’s overall clarity.
- Modular Design: Break down large tasks into smaller, more manageable functions. This promotes code reusability and reduces complexity.
Sharing Projects Online (e.g., GitHub)
Using a platform like GitHub is a great way to share and manage your projects. It provides version control and collaboration features, which are important for any project, even if you’re working alone. It builds your online presence and makes it easy to share your work with others.
- Creating a Repository: This involves creating a new repository on GitHub to hold your project’s files. This repository serves as a central location for your project’s code and related documentation.
- Committing Changes: When you make changes to your project’s files, use Git to commit them to your repository. Commit messages should clearly describe the changes you made, aiding in understanding and tracking progress.
- Pushing Changes: Pushing your commits to the repository uploads them to GitHub. This makes the changes publicly accessible (or private if chosen). This is an essential step for sharing your project with others.
Creating a Simple Web Application and Deploying It
A simple web application can be deployed using platforms like GitHub Pages, Netlify, or Vercel. These platforms provide a straightforward way to get your application live without needing to manage servers. These are suitable choices for beginners to start building and deploying web applications.
- Choosing a Framework: Select a suitable framework for building the application. For example, you could use HTML, CSS, and JavaScript for a simple web page.
- Using a Deployment Platform: Use platforms like GitHub Pages to deploy the application’s files. This often involves following the platform’s specific instructions for setting up your repository.
- Testing and Debugging: Thoroughly test your application before deploying it. Ensure the application functions as expected across different browsers and devices.
Using Git for Version Control and Sharing
Git is a powerful version control system. It’s fundamental for managing changes to your project’s code over time, enabling you to revert to previous versions if needed. It also facilitates collaboration.
- Basic Git Commands: Learn essential Git commands like `git init`, `git add`, `git commit`, `git push`, and `git pull`. These commands are crucial for managing your project’s code using Git.
- Understanding Branches: Branches allow you to work on different features or bug fixes independently without affecting the main codebase. They’re crucial for managing complexity in a project.
Presenting Project Findings and Results
Presenting project findings and results effectively is crucial for communicating your work to others. A well-structured presentation can highlight the project’s strengths and clearly convey its significance.
- Documentation: Create detailed documentation to explain the project’s purpose, design, implementation, and results. Clear and comprehensive documentation helps others understand your work.
- Visualizations: Use graphs, charts, or other visual aids to present complex data effectively. Visualizations make data easier to understand and interpret.
Deployment Platforms for Beginner Projects
This table Artikels various deployment platforms suitable for beginner projects, highlighting their features and ease of use.
Platform | Ease of Use | Features | Suitable for |
---|---|---|---|
GitHub Pages | High | Free, simple, integrated with GitHub | Static websites, personal projects |
Netlify | High | Handles deployments automatically, various integrations | Static websites, simple web apps |
Vercel | High | Fast deployments, supports various frameworks | Web apps, dynamic websites |
Firebase Hosting | Medium | Database integration, mobile app deployment | Mobile apps, web apps with backend integration |
Conclusion: Top Projects Beginner Programmers
In conclusion, this guide provides a comprehensive overview of top projects suitable for beginner programmers. We’ve covered crucial aspects like project selection, design, implementation, and deployment, equipping you with the knowledge and resources to create impactful projects. By choosing appropriate projects and following best practices, you can not only enhance your programming skills but also develop a strong foundation for future endeavors.
Post Comment