Best Coding Practices Developers Should Know
Best coding practices developers should know are essential for creating high-quality, maintainable, and efficient software. This guide delves into crucial aspects of clean code, error handling, documentation, version control, testing, security, performance optimization, refactoring, and selecting appropriate tools and libraries. Understanding these practices will empower developers to write better code and contribute to successful projects.
From fundamental principles of clean code and effective error handling to the critical role of version control and collaboration, this guide explores a comprehensive spectrum of best practices. It emphasizes not just the ‘what’ but also the ‘why’ behind each practice, enabling developers to not only follow the rules but also grasp the underlying reasoning and context. The discussion includes practical examples and detailed explanations to solidify understanding and facilitate implementation.
Fundamentals of Clean Code
Clean code is a cornerstone of software development. It’s more than just readable; it’s about writing code that’s maintainable, understandable, and adaptable to future changes. This approach fosters collaboration, reduces errors, and streamlines the development process. Clean code directly translates to improved productivity and a lower cost of ownership over time.Writing clean code involves adherence to established principles and styles, fostering code clarity and maintainability.
This focus on readability and structure directly impacts the ease with which others, or even the original author at a later date, can understand and modify the code.
Principles of Clean Code
Clean code adheres to several key principles. These principles are crucial for creating software that is easily understandable, maintainable, and adaptable. They aim to ensure the code is both readable and understandable by any developer, regardless of their familiarity with the specific codebase.
- Readability: Code should be easy to understand at a glance. Meaningful variable names, well-structured functions, and clear comments are essential. Avoid cryptic abbreviations and overly complex logic. The primary focus should be on clarity and straightforwardness.
- Maintainability: Code should be easy to modify and adapt to changing requirements. Well-defined functions, modular design, and separation of concerns are crucial. Changes should be localized to specific parts of the code, minimizing the risk of introducing errors elsewhere.
- Modularity: Code should be divided into small, independent, and well-defined units. This promotes reusability and reduces complexity. Functions should perform a single, specific task. This approach improves the overall maintainability of the codebase.
- Conciseness: Code should be concise and avoid unnecessary complexity. Avoid redundant code or verbose expressions. Clean code gets to the point without unnecessary details. This efficiency is a hallmark of good design.
Code Examples and Refactoring
Consider this example of code that violates clean code principles:“`java// Bad exampleint total = 0;for (int i = 0; i < 10; i++) total += i - 2; System.out.println("The total is: " + total); //Refactored Example int calculateTotal() int sum = 0; for (int i = 0; i < 10; i++) sum += i - 2; return sum; int total = calculateTotal(); System.out.println("The total is: " + total); ``` The refactored code is more readable and maintainable, encapsulating the calculation logic into a function called `calculateTotal`.
Code Formatting Styles
Consistent code formatting is crucial for readability and maintainability. Different programming languages and communities have established styles like PEP 8 for Python and Google Java Style Guide. These styles define conventions for indentation, spacing, naming, and commenting, promoting consistency across the codebase.
Style Guide | Pros | Cons |
---|---|---|
PEP 8 (Python) | Promotes readability and consistency in Python code. Extensive community support and readily available tools for automated formatting. | Might feel slightly restrictive for very specific cases, but it promotes consistency across a project. |
Google Java Style Guide | Provides clear guidelines for formatting Java code, fostering consistency across projects. | Can be perceived as inflexible by some developers. It’s important to adhere to it strictly for consistency. |
Other Style Guides | Tailored to specific languages and communities, often offering specific recommendations for those languages. | Requires understanding of the specific guidelines and adhering to them for a particular project. |
Naming Conventions
Consistent naming conventions enhance code readability. These conventions apply to variables, functions, and classes.
- Variables: Use descriptive names that clearly indicate the variable’s purpose. Avoid single-letter names unless they represent common mathematical constructs. Use camelCase for variable names (e.g., `customerName`).
- Functions: Function names should be verbs or verb phrases that clearly indicate the function’s purpose. Use PascalCase for function names (e.g., `calculateTotal`).
- Classes: Class names should be nouns or noun phrases that represent the class’s purpose. Use PascalCase for class names (e.g., `Customer`).
Error Handling and Debugging
Effective error handling and debugging are crucial aspects of software development. Robust error handling prevents unexpected crashes and provides meaningful feedback to users and developers. Debugging techniques help identify and fix issues within the code, improving its reliability and maintainability. These skills are essential for building high-quality, stable software applications.Proper error handling is paramount for maintaining a user-friendly and stable application.
By anticipating and addressing potential errors, developers can prevent application crashes and ensure a smoother user experience. Efficient debugging techniques are equally important for quickly identifying and fixing problems, allowing for iterative improvements and minimizing downtime.
Strategies for Handling Potential Errors and Exceptions
Proper error handling involves anticipating potential issues and designing mechanisms to manage them gracefully. This includes identifying potential error sources, implementing appropriate exception handling, and providing informative error messages. Employing try-catch blocks in programming languages like Python or Java is a common strategy.
Writing Robust Error Handling Mechanisms
A robust error handling mechanism should prevent application crashes and provide informative error messages. Clear error messages aid in understanding the issue and its location, enabling faster resolution. The messages should be user-friendly, yet contain sufficient technical details for debugging purposes. Consider using logging to record errors for later analysis. Avoid displaying sensitive information in error messages to users.
Techniques for Debugging Code
Effective debugging relies on a combination of strategies, including logging, breakpoints, and debugging tools. Logging provides a record of events, enabling developers to trace the execution flow and identify the root cause of errors. Breakpoints allow pausing the program’s execution at specific points, enabling examination of variables and their values. Debugging tools offer additional features, such as variable inspection, call stack analysis, and stepping through code.
Debugging Tools and Their Use Cases
- Debuggers (e.g., pdb in Python, the integrated debugger in IDEs like VS Code or Eclipse): Debuggers provide a comprehensive environment for inspecting code, stepping through execution, setting breakpoints, and examining variables. They’re invaluable for identifying the source and nature of bugs in complex code.
- Logging (e.g., Python’s logging module): Logging allows recording events and errors during program execution. This helps in tracing the flow of the program, identifying problematic sections, and pinpointing the cause of issues. Different log levels (e.g., debug, info, warning, error, critical) help categorize events.
- Print statements: While less sophisticated than debuggers, print statements are a simple and effective way to inspect variable values and program flow during debugging. These can be used for simple cases or as temporary debugging aids.
Example of Error Handling
“`pythondef divide(a, b): try: result = a / b return result except ZeroDivisionError: return “Division by zero error occurred.” except TypeError: return “Invalid input type.
Both inputs must be numeric.”# Example usageprint(divide(10, 2)) # Output: 5.0print(divide(10, 0)) # Output: Division by zero error occurred.print(divide(10, “a”)) # Output: Invalid input type. Both inputs must be numeric.“`This Python code demonstrates how to handle different potential errors, preventing the program from crashing and providing specific feedback on the error type. The try-except block catches potential errors, and each except block handles a specific error type, returning a relevant message.
Code Comments and Documentation
Clear and concise code comments and documentation are crucial for maintainability and collaboration in software development. They act as a form of communication, explaining the intent behind the code, and providing context for others (and future you) to understand and modify it. Well-documented code is easier to debug, update, and extend, ultimately saving time and effort in the long run.Effective documentation goes beyond just explaining the code’s functionality.
It helps to explainwhy* certain decisions were made, the trade-offs considered, and any potential issues or limitations. This helps maintain the integrity and consistency of the codebase.
Importance of Code Comments
Comprehensive comments explain the rationale behind code choices, making it easier to understand the code’s purpose and behavior. This is particularly valuable for complex algorithms, intricate logic, or sections of code that may not be immediately obvious. Comments should not simply restate the obvious; instead, they should focus on the
- why* and
- how*.
Different Commenting Styles
Various commenting styles exist, each with its advantages. One common style uses block comments to explain a section of code, while inline comments provide concise explanations for individual lines or statements.
- Block Comments: These comments are used to describe larger blocks of code, methods, or classes. They provide an overview of the code’s functionality and any crucial considerations. This makes it easier to understand the high-level purpose of the code section. For example, a block comment might describe the purpose of a function, outlining its inputs, outputs, and any specific steps.
- Inline Comments: These comments are used to explain individual lines or small segments of code. They are often used to document non-obvious operations or unusual logic. Inline comments should be concise and to the point, avoiding redundancy. For example, an inline comment might clarify the purpose of a specific variable assignment or the reason behind a conditional statement.
Strategies for Documenting Code
Several strategies can be used to document code effectively. A common approach involves combining code comments with external documentation, such as Javadocs or similar tools. These tools generate API documentation that is easily accessible and up-to-date.
- Javadocs: Javadoc is a documentation generator for Java code. It automatically generates API documentation from special comments within the code. Javadocs are well-structured, allowing developers to quickly understand class and method parameters, return values, and exceptions. The generated HTML documentation can be easily integrated into project documentation.
- Docstrings (Python): Python uses docstrings to document code. Docstrings are enclosed within triple quotes and are used to describe functions, classes, modules, and methods. These docstrings are then processed by tools to generate documentation. This style ensures consistency and clarity.
- Code Style Guides: Adhering to consistent code style guides enhances readability and maintainability. These guides often dictate the formatting of comments and the structure of documentation. Consistent application of code style guidelines creates a standardized documentation approach.
Examples of Well-Commented and Poorly-Commented Code
Well-Commented Code (Example):“`java// Calculate the area of a rectangle.public class RectangleArea public static double calculateArea(double length, double width) // Validate inputs to prevent errors. if (length <= 0 || width <= 0) throw new IllegalArgumentException("Length and width must be positive values."); // Calculate the area. double area = length - width; return area; ``` Poorly-Commented Code (Example): “`javapublic class RectangleArea public static double calculateArea(double length, double width) double area = length – width; return area; // return area “`The well-commented code clearly explains the purpose, validation, and calculation.
The poorly-commented code lacks any explanation, making it hard to understand the intent behind the code.
Comparison of Documentation Approaches
Approach | Description | Advantages | Disadvantages |
---|---|---|---|
Javadocs | Java-specific tool for generating API documentation | Automated documentation generation, clear structure, easily integrated into project documentation. | Limited to Java projects, additional setup required. |
Docstrings | Python-specific approach for documenting code | Integrated into the code, easily accessible, good for code readability. | Requires consistent formatting, not automatically generated documentation. |
Code Comments | Inline or block comments within the code | Simple, directly related to the code. | Can become verbose, difficult to maintain consistency, not easily accessible. |
Version Control and Collaboration
Version control systems, like Git, are indispensable for managing code projects effectively. They track changes over time, allowing developers to revert to previous versions, collaborate seamlessly, and manage complex codebases efficiently. This is crucial for teams working on large projects, ensuring everyone is on the same page and preventing conflicts. Maintaining a clear history of modifications also helps in understanding the evolution of a project and identifying the source of bugs.
Importance of Version Control Systems
Version control systems, such as Git, are essential for tracking changes to source code, enabling collaboration among developers, and facilitating efficient project management. They offer a centralized repository for storing code, allowing multiple developers to work concurrently on the same project without overwriting each other’s changes. This crucial function prevents data loss and ensures that all changes are documented and traceable.
Version control systems also provide a mechanism for reverting to previous versions of the code if necessary.
Branching Strategies
Branching strategies in Git enable developers to isolate work on new features, bug fixes, or experiments without affecting the main codebase. This crucial approach allows for parallel development and helps manage complexity. Different branching strategies suit various project needs.
- Gitflow: A popular branching model that organizes branches into different categories for releases, features, and bug fixes. This structured approach is particularly helpful for large-scale projects with multiple releases, enabling clearer separation of concerns.
- GitHub Flow: A simpler, streamlined branching model suitable for smaller projects or those with frequent releases. This strategy focuses on merging changes directly into the main branch, promoting a more rapid development cycle.
- Gitlab Flow: Similar to GitHub Flow, GitLab Flow emphasizes merging changes directly into the main branch. It’s a streamlined process, beneficial for smaller projects or those prioritizing fast development cycles.
Common Git Commands
Git offers a suite of commands for managing the codebase. Mastering these commands is vital for efficient collaboration and development.
- `git init`: Initializes a new Git repository. This command sets up the necessary files and directories for tracking changes.
- `git add`: Stages changes for commit. This command prepares changes for inclusion in the next commit.
- `git commit`: Records changes to the repository. This command creates a snapshot of the current state of the project.
- `git branch`: Creates, lists, or deletes branches. This command is essential for managing different development paths.
- `git merge`: Combines changes from one branch into another. This command is used to integrate changes from a branch into the main codebase.
- `git pull`: Downloads changes from a remote repository. This command updates the local repository with changes from the remote repository.
- `git push`: Uploads local commits to a remote repository. This command synchronizes the local repository with the remote repository.
Collaboration Practices
Collaboration in a team environment using Git involves several key practices:
- Clear Communication: Open communication channels and clear guidelines for code reviews are vital for effective collaboration.
- Code Reviews: Peer reviews of code help identify potential issues, improve code quality, and promote knowledge sharing among team members.
- Conflict Resolution: Git’s merge functionality, combined with careful code review and communication, facilitates resolving conflicts that may arise from concurrent modifications.
Git Commands Table
The table below summarizes common Git commands and their functions.
Command | Description |
---|---|
`git init` | Initializes a new Git repository |
`git add` | Stages changes for commit |
`git commit` | Records changes to the repository |
`git branch` | Creates, lists, or deletes branches |
`git merge` | Combines changes from one branch into another |
`git pull` | Downloads changes from a remote repository |
`git push` | Uploads local commits to a remote repository |
Testing and Quality Assurance
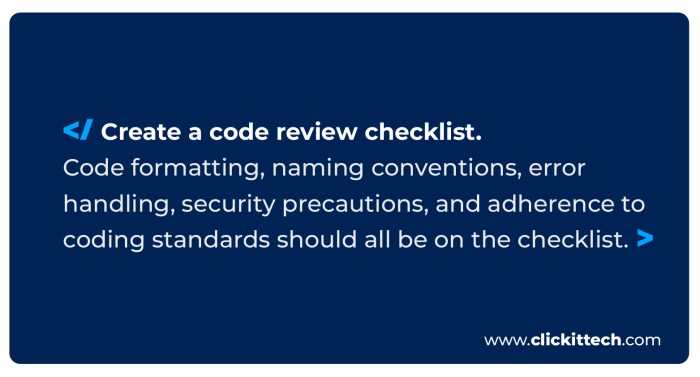
Source: clickittech.com
Thorough testing is crucial for delivering high-quality software. It ensures that the software functions as intended, meets user requirements, and is robust against potential issues. Effective testing strategies not only identify defects early in the development lifecycle but also contribute to building confidence in the final product.Robust testing practices are essential for producing reliable software. By anticipating and addressing potential problems early, developers can mitigate risks and ensure a smooth user experience.
This approach ultimately leads to a more stable and trustworthy product.
Different Types of Software Testing, Best coding practices developers should know
Various testing methodologies exist, each targeting specific aspects of the software. Understanding these distinctions is vital for crafting effective testing strategies.
- Unit Testing focuses on the smallest testable units of code, typically individual functions or methods. This level of testing verifies that each component operates as expected in isolation. Unit tests are often automated, making them efficient for frequent verification during development.
- Integration Testing validates how different modules interact with one another. It ensures that the interfaces between units are functioning correctly and that data flows smoothly between them. This process helps uncover issues related to communication and data exchange between components.
- System Testing assesses the entire software system as a whole. It verifies that the system meets its specified requirements and behaves as anticipated in a complete environment. System testing encompasses the software’s functionality, performance, and security in a comprehensive manner.
- Acceptance Testing determines whether the software satisfies the user’s needs and expectations. This final stage involves real-world scenarios and user interactions to confirm that the software meets the acceptance criteria set by stakeholders. This is often conducted by end-users or representatives.
Significance of Comprehensive Test Cases
Writing comprehensive test cases is a key aspect of ensuring software quality. These cases should cover various scenarios, including normal cases, boundary conditions, and error conditions.Comprehensive test cases ensure the software functions correctly under a range of conditions, including unexpected inputs. Thoroughness in test cases contributes to the software’s robustness and reliability.
Examples of Unit Tests
Unit testing frameworks provide tools for automating tests and ensuring their effectiveness. These frameworks help maintain test code consistency and improve the overall quality of the software.
- JUnit (Java): JUnit is a widely used unit testing framework for Java. It allows developers to define test methods within their code, asserting expected results against actual results.
import org.junit.Test; import static org.junit.Assert.*; public class CalculatorTest @Test public void testAdd() Calculator calc = new Calculator(); assertEquals(5, calc.add(2, 3));
- Pytest (Python): Pytest is a popular testing framework for Python. It provides a simple and flexible way to write and run tests.
import pytest def add(x, y): return x + y def test_add(): assert add(2, 3) == 5 assert add(-1, 1) == 0
Role of Code Reviews in Ensuring Quality
Code reviews play a crucial role in identifying potential issues and improving code quality. A thorough review can catch errors, improve design, and ensure adherence to coding standards.
Code reviews, performed by peers, can significantly improve the quality of the software. They act as a valuable checkpoint in the development process.
Examples of Test-Driven Development (TDD)
Test-driven development (TDD) is an iterative approach to software development that emphasizes writing tests before writing the code they are intended to test.
- TDD emphasizes creating tests that define the expected behavior before implementing the actual code. This approach ensures the software meets the requirements and facilitates easier debugging.
Security Best Practices
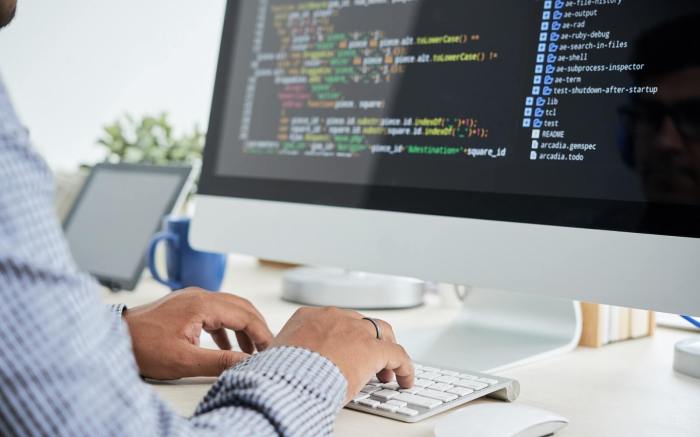
Source: cheapsslsecurity.com
Robust security is paramount in modern software development. Protecting applications and user data from malicious attacks requires a proactive approach, integrating security considerations throughout the entire development lifecycle. This involves understanding common vulnerabilities and implementing preventative measures to safeguard against threats.
Security vulnerabilities can have severe consequences, including data breaches, financial losses, and reputational damage. By understanding the potential risks and implementing secure coding practices, developers can mitigate these threats and build more resilient applications.
Common Security Vulnerabilities
Understanding common security vulnerabilities is the first step towards building secure applications. These vulnerabilities can stem from various sources, including insecure design, improper input validation, and insufficient access controls.
A thorough understanding of these vulnerabilities enables developers to implement appropriate countermeasures and build more resilient applications.
SQL Injection
SQL injection attacks exploit vulnerabilities in applications that handle user input without proper sanitization. Attackers inject malicious SQL code into input fields, manipulating database queries to gain unauthorized access or perform malicious actions.
Preventing SQL injection requires meticulous validation of user input before it’s used in database queries. Parameterized queries are a crucial technique to separate data from the query structure, mitigating the risk of injection attacks.
Cross-Site Scripting (XSS)
Cross-site scripting (XSS) attacks involve injecting malicious scripts into web pages viewed by other users. These scripts can steal sensitive information, redirect users to malicious websites, or deface web pages.
Preventing XSS involves proper encoding and escaping of user-supplied data in web pages to prevent the execution of malicious scripts. Using appropriate output encoding libraries is a key preventive measure.
Other Common Vulnerabilities
Besides SQL injection and XSS, several other vulnerabilities pose significant risks. These include insecure direct object referencing, broken authentication, and insecure deserialization. Understanding these threats is essential for building secure applications.
Secure Coding Practices for User Input
Validating and sanitizing user input is crucial for preventing security vulnerabilities. Always validate input against expected formats and ranges, and sanitize data to remove potentially harmful characters or code.
Employing input validation libraries and adhering to strict input validation rules can greatly reduce the risk of vulnerabilities. For example, if an input field expects an integer, verify that the input is indeed an integer and handle cases where the input is not a valid integer appropriately.
Secure Coding Practices for Sensitive Data
Handling sensitive data, such as passwords and credit card information, requires robust security measures. Encrypt sensitive data both in transit and at rest. Use strong encryption algorithms and securely store encryption keys.
Storing sensitive data using strong encryption algorithms and securely managing encryption keys is critical. Leveraging established encryption libraries and industry best practices ensures the security of sensitive data.
Vulnerability Countermeasures
| Vulnerability | Countermeasure |
|—|—|
| SQL Injection | Parameterized queries, input validation |
| Cross-Site Scripting (XSS) | Output encoding, input validation |
| Insecure Direct Object Referencing | Input validation, access control |
| Broken Authentication | Strong password policies, secure session management |
| Insecure Deserialization | Validate incoming data, use secure deserialization libraries |
Examples of Vulnerable and Secure Code
Example of vulnerable code (SQL Injection):
“`sql
// Vulnerable code
String query = “SELECT
– FROM users WHERE username = ‘” + username + “‘”;
“`
Secure code (using parameterized query):
“`sql
// Secure code
String query = “SELECT
– FROM users WHERE username = ?”;
PreparedStatement statement = connection.prepareStatement(query);
statement.setString(1, username);
“`
These examples demonstrate the crucial difference between vulnerable and secure code. Using parameterized queries prevents SQL injection vulnerabilities.
Performance Optimization
Optimizing code performance is crucial for delivering responsive and efficient applications. A well-optimized application provides a better user experience, reduced server load, and improved overall system stability. This section delves into strategies for boosting code performance, techniques for identifying bottlenecks, and examples of common pitfalls across various programming languages.
Effective performance optimization involves a multifaceted approach that considers algorithmic efficiency, data structures, and language-specific performance characteristics. It’s not a one-size-fits-all solution, but rather a continuous process of identifying and addressing bottlenecks to enhance application speed.
Performance Bottlenecks Identification
Identifying performance bottlenecks is a critical step in optimizing code. Profiling tools are instrumental in pinpointing sections of code that consume significant processing time. By analyzing execution traces and resource utilization, developers can isolate areas needing improvement. Careful analysis of the performance profile reveals the specific code segments that require optimization.
Profiling Techniques
Various profiling tools and techniques are available for different programming languages. These tools often provide insights into the execution time spent in different parts of the application, enabling developers to pinpoint areas needing optimization. For example, in Python, tools like cProfile and line_profiler can provide detailed information about the time spent in various functions and lines of code.
In Java, tools like JProfiler or YourKit provide comprehensive profiling capabilities. Understanding the specific tools available for the chosen language and how to utilize them effectively is crucial for successful optimization.
Code Examples Demonstrating Poor Performance and Optimization
Consider the following Python code snippet:
“`python
import time
def slow_function(n):
result = 0
for i in range(n):
for j in range(n):
result += i
– j
return result
def main():
n = 10000
start_time = time.time()
slow_function(n)
end_time = time.time()
print(f”Time taken for slow_function: end_time – start_time:.6f seconds”)
if __name__ == “__main__”:
main()
“`
This code demonstrates poor performance due to nested loops. The execution time increases dramatically with larger values of ‘n’.
Optimization:
“`python
import time
def fast_function(n):
result = 0
for i in range(n):
result += i
– i
– (n + 1) / 2
return result
def main():
n = 10000
start_time = time.time()
fast_function(n)
end_time = time.time()
print(f”Time taken for fast_function: end_time – start_time:.6f seconds”)
if __name__ == “__main__”:
main()
“`
The optimized code utilizes a mathematical formula to calculate the sum, which drastically reduces execution time compared to the nested loops. The optimization leverages the mathematical summation formula, significantly improving the efficiency.
Common Performance Pitfalls in Programming Languages
Several common performance pitfalls exist across various programming languages. Inefficient algorithms, unnecessary computations, improper data structures, and excessive memory allocation are frequent issues. For example, using inefficient sorting algorithms can significantly impact performance, especially with large datasets. Overuse of complex object graphs can lead to performance issues due to garbage collection overhead.
Comparison of Performance Optimization Techniques
Technique | Description | Advantages | Disadvantages |
---|---|---|---|
Algorithm Optimization | Improving the efficiency of the underlying algorithm | Potentially significant performance gains | Requires understanding of algorithms and data structures |
Data Structure Optimization | Selecting appropriate data structures to reduce complexity | Improved performance for specific operations | Requires understanding of different data structures |
Caching | Storing frequently accessed data for faster retrieval | Reduced latency for repeated lookups | Can increase memory consumption |
Profiling | Identifying performance bottlenecks in the code | Provides insights for targeted optimization | Requires profiling tools and knowledge |
Code Refactoring and Maintainability
Refactoring is a crucial aspect of software development, encompassing the restructuring of existing code without altering its external behavior. This process aims to improve code readability, maintainability, and overall quality. By identifying and addressing code smells, developers can enhance the long-term viability and resilience of their software projects.
Refactoring is not simply about rewriting code; it’s about improving the underlying design and structure to make it easier to understand, modify, and extend in the future. This practice is essential for long-term project success and contributes significantly to the overall efficiency of the development team.
Importance of Refactoring
Refactoring plays a pivotal role in enhancing code maintainability. Well-structured, readable code is easier to understand and modify, reducing the likelihood of introducing bugs during future changes. This, in turn, leads to faster development cycles and lower maintenance costs. Refactoring also promotes code quality by addressing potential problems early in the development process.
Common Refactoring Patterns
Refactoring encompasses a variety of techniques. These techniques can be categorized into different patterns, each designed to address specific code issues. Understanding these patterns allows developers to effectively improve their code.
Refactoring Examples
Consider the following example of a function with redundant calculations:
“`java
public int calculateArea(int length, int width)
int area = length
– width;
int perimeter = 2
– (length + width);
return area;
“`
This function calculates the area but also calculates the perimeter unnecessarily. Refactoring this function to calculate only the required value improves code efficiency and readability.
“`java
public int calculateArea(int length, int width)
int area = length
– width;
return area;
“`
This refactored version eliminates unnecessary calculations, focusing solely on the area calculation.
Role of Automated Refactoring Tools
Automated refactoring tools provide significant assistance in identifying and applying refactoring patterns. These tools can automatically detect code smells, suggest appropriate refactorings, and apply them with minimal human intervention. This significantly accelerates the refactoring process and reduces the likelihood of errors. Integrated Development Environments (IDEs) often include built-in refactoring tools that make the process seamless.
Refactoring Patterns and Use Cases
Refactoring Pattern | Description | Use Case |
---|---|---|
Extract Method | Extracts a code block into a new method, improving code organization and reducing code duplication. | When a block of code is repeated in multiple places or is too long and complex. |
Rename Method/Variable | Improves code clarity by giving more descriptive names to methods and variables. | When the current names of methods or variables are not intuitive or do not accurately reflect their purpose. |
Introduce Parameter Object | Creates a new object to encapsulate multiple parameters, improving code organization and readability. | When a method has a large number of parameters. |
Replace Conditional with Polymorphism | Replaces conditional statements with polymorphism, improving code flexibility and maintainability. | When dealing with multiple conditional branches based on different types of objects. |
Choosing the Right Tools and Libraries: Best Coding Practices Developers Should Know
Selecting appropriate tools and libraries is crucial for project success. A well-chosen set of tools can significantly improve development speed, maintainability, and overall project efficiency. Conversely, poor tool choices can lead to frustration, wasted time, and even project failure. Understanding the factors influencing selection, the benefits and drawbacks of various options, and the importance of well-maintained libraries are all vital aspects of effective software development.
Effective software development hinges on using tools and libraries that align with project requirements and team expertise. This selection process requires careful consideration of factors ranging from project scope and complexity to team familiarity and available resources. Thorough evaluation and comparison are essential to ensure the chosen tools and libraries are well-suited for the task at hand.
Factors to Consider When Selecting Tools and Libraries
A comprehensive evaluation considers several key factors. These factors include the project’s specific needs, the available resources, and the team’s familiarity with different tools.
- Project Requirements: Understanding the project’s specific functionalities and constraints is paramount. For example, a web application requiring real-time data updates might benefit from a specific framework designed for this purpose, whereas a simple desktop application might not need such advanced features.
- Performance Requirements: Consider the anticipated load and user base. A library designed for high-performance environments may be unnecessary for a small-scale project, leading to unnecessary complexity.
- Scalability Needs: The anticipated growth of the project influences the selection. A library that easily scales with increasing data volumes is crucial for future expansion.
- Team Expertise: Selecting tools and libraries that align with the team’s existing skills and knowledge base ensures smoother integration and faster development cycles. Trying to introduce tools that require significant training for the team can delay projects.
- Maintenance and Support: The availability of ongoing maintenance and support for the chosen tools and libraries is critical for long-term project stability. Tools with a strong community and active maintenance will be better suited for long-term projects.
- Cost and Licensing: Financial constraints and licensing agreements must be considered. Open-source options often offer significant cost advantages and flexibility compared to proprietary tools.
Benefits and Drawbacks of Various Tools and Libraries
Different tools and libraries offer varying advantages and disadvantages. Understanding these trade-offs is essential for informed decision-making.
- Example: A popular JavaScript framework like React might offer rapid development and a large community, but might also introduce complexity for simpler projects. A lightweight library like jQuery might be ideal for straightforward tasks but lack the features and support for larger applications.
- Frameworks vs. Libraries: Frameworks provide a structured approach to development, guiding the application’s architecture. Libraries, on the other hand, offer specific functionalities that can be integrated into existing projects. The choice between a framework and a library depends on the project’s scale and requirements.
Importance of Well-Maintained and Documented Libraries
Using well-maintained and documented libraries significantly improves development efficiency and reduces the likelihood of errors.
Well-maintained libraries provide consistent updates, address security vulnerabilities, and offer clear guidance through comprehensive documentation.
Thorough documentation is essential for understanding the library’s functionality and usage, ensuring effective integration. The lack of maintenance can introduce vulnerabilities and make future modifications or updates difficult.
Impact on Project Efficiency
The selection of the right tools and libraries directly impacts project efficiency.
- Improved Development Speed: Choosing tools that align with the project’s needs can significantly accelerate development cycles. Libraries and frameworks that support modular development, for instance, enable faster code implementation.
- Reduced Debugging Time: Well-documented libraries often provide comprehensive examples and troubleshooting resources, leading to reduced debugging time.
- Enhanced Maintainability: Libraries that support modularity and clear code structure improve the long-term maintainability of the project.
Process of Evaluating and Selecting Appropriate Tools
The process of selecting the right tools involves careful evaluation and comparison.
- Identify the project’s needs. This includes assessing the project’s scope, functionalities, performance requirements, and scalability needs.
- Research potential tools and libraries. Examine documentation, reviews, and examples to evaluate their features, capabilities, and suitability.
- Compare and contrast different options. Consider the pros and cons of each tool, weighing factors like performance, scalability, community support, and documentation.
- Evaluate the suitability for the team. Consider the team’s existing expertise and knowledge of various tools.
- Trial and test different tools. Experiment with the tools to ensure they meet the project’s needs and integrate seamlessly with the existing codebase.
Final Wrap-Up
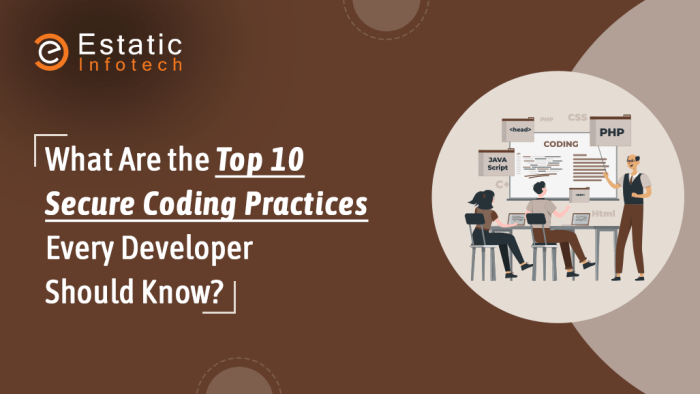
Source: estatic-infotech.com
In conclusion, mastering best coding practices is a continuous learning journey. By understanding and applying the principles discussed in this guide, developers can enhance their code quality, improve team collaboration, and build more robust and maintainable software. This comprehensive overview serves as a valuable resource for aspiring and experienced developers alike, fostering a deeper understanding of the critical aspects of professional software development.
Post Comment