Common Mistakes Beginner Programmers Make
Common mistakes beginner programmers make are surprisingly frequent and often stem from misunderstandings of fundamental programming concepts. This guide delves into various pitfalls, from basic syntax errors to more complex issues related to algorithm design and debugging. Learning to identify and avoid these errors is crucial for effective programming and accelerating your learning journey.
We’ll explore common misconceptions about programming fundamentals, syntax, and structure. We’ll also look at debugging techniques, logical errors, data structures, problem-solving approaches, using libraries and frameworks, version control, and testing. This comprehensive overview aims to empower you with the knowledge to confidently navigate these challenges and become a more proficient programmer.
Fundamentals of Programming
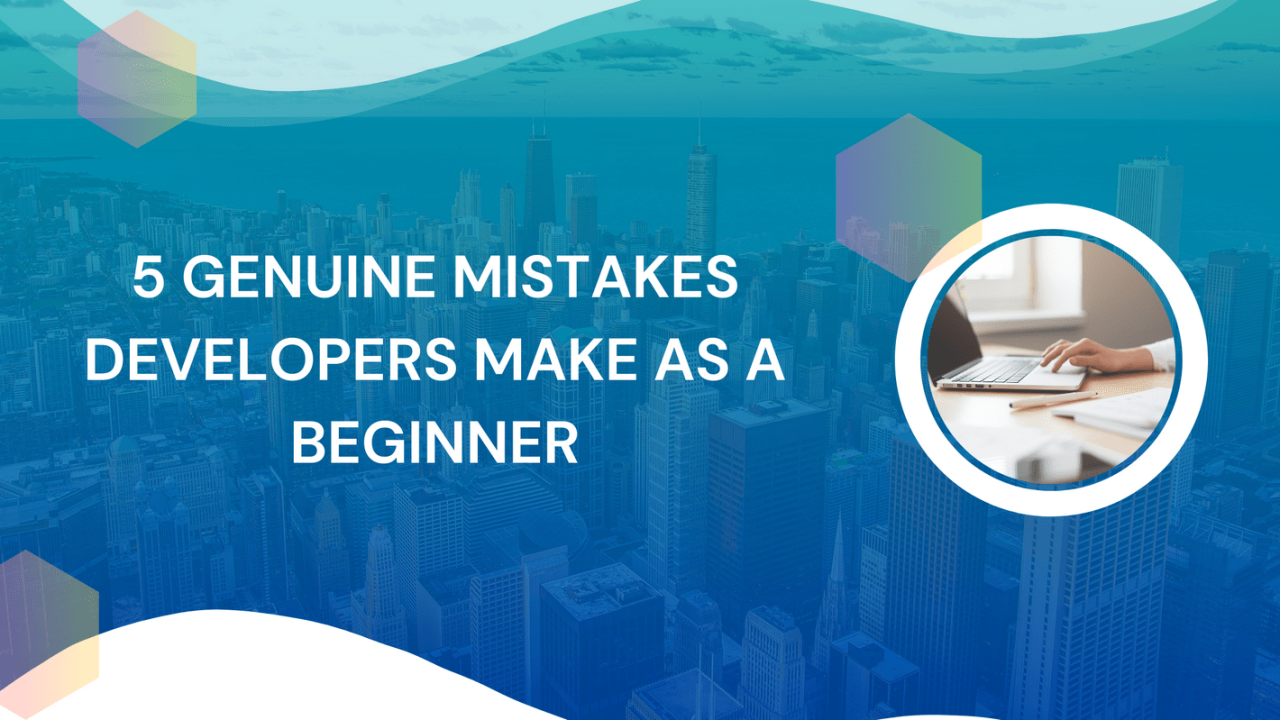
Source: showwcase.com
A strong foundation in programming basics is crucial for any aspiring programmer. Understanding fundamental concepts like variables, data types, and operators, and the different approaches to problem-solving, is essential for building robust and efficient code. Mastering these principles avoids common pitfalls and paves the way for more complex programming endeavors.
Common Misconceptions about Programming Basics
Many beginners harbor misconceptions about programming fundamentals. These misconceptions, if left unaddressed, can hinder progress and lead to frustration.
- Thinking programming is solely about memorizing syntax. While syntax is essential, true programming proficiency stems from understanding the underlying logic and problem-solving approach. Focusing solely on rote memorization often results in a lack of flexibility and adaptability in dealing with varied coding challenges.
- Believing that all programming languages are identical. While many languages share similarities, each has its own syntax, nuances, and capabilities. A rigid mindset that one language is universally applicable can lead to difficulties in adapting to different programming environments and tasks.
- Ignoring the importance of clear documentation and comments. Effective documentation is key to understanding and maintaining code, especially in collaborative environments. Neglecting to document code adequately can lead to significant problems during code maintenance or future modifications.
- Underestimating the significance of debugging. Debugging is an integral part of the programming process. Effective debugging techniques, including systematic testing and careful analysis of error messages, are critical for identifying and correcting errors.
Importance of Fundamental Concepts
Variables, data types, and operators are fundamental building blocks in programming. Understanding them is crucial for creating programs that accurately process and manipulate data.
- Variables act as named containers for data. They store values of different types, such as numbers, text, or booleans. Incorrect variable declaration or usage can lead to errors in data storage and retrieval.
- Data types define the kind of data a variable can hold. Using the wrong data type can lead to unexpected results or errors, such as attempting to perform mathematical operations on text data.
- Operators perform operations on data stored in variables. Misusing operators, such as applying an incorrect arithmetic operator to a string variable, will cause unexpected results.
Examples of Errors from Incorrect Application
Inaccurate application of fundamentals can lead to numerous errors.
- Trying to perform arithmetic operations on strings, such as adding “10” and “20” without converting them to numbers, results in string concatenation instead of addition. This will lead to an error if not anticipated.
- Incorrect data type assignments can cause a program to fail or produce unexpected outputs. Attempting to store a decimal number in an integer variable can truncate the decimal part.
- Improper variable initialization leads to undefined values. Using a variable without assigning a value can result in unpredictable program behavior.
Comparing Programming Paradigms
Different programming paradigms offer distinct approaches to problem-solving.
Paradigm | Typical Beginner Mistakes |
---|---|
Procedural | Focusing solely on sequential steps without considering modularity or reusability. Poor function design can lead to complex, difficult-to-maintain code. |
Object-Oriented | Difficulty in identifying objects and classes, leading to incorrect class hierarchies or inadequate data encapsulation. Incorrect instantiation or method invocation can cause errors. |
Functional | Struggling to understand the concepts of immutability and pure functions. Incorrect use of higher-order functions or improper handling of side effects can lead to unexpected program behavior. |
Syntax and Structure
Mastering syntax and structure is crucial for any programmer. Correct syntax ensures your code is understood by the compiler or interpreter, while proper structure makes the code readable, maintainable, and less prone to errors. This section delves into common pitfalls beginners face with syntax, illustrating the impact of errors and offering best practices.
Common Syntax Errors
Understanding the syntax of a programming language is fundamental. Novice programmers frequently encounter errors due to incorrect syntax. These errors can range from simple typos to more complex issues, affecting various programming languages like Python, JavaScript, and Java. For example, a missing colon in a Python conditional statement or an incorrect use of parentheses in a JavaScript function call can lead to unexpected behavior.
Impact of Incorrect Syntax
Incorrect syntax can lead to a variety of issues, from simple error messages to program crashes. Missing semicolons in JavaScript or Java can cause parsing errors, leading to the code not running at all or running with unexpected results. Indentation errors in Python, crucial for defining code blocks, result in errors like `IndentationError`. These errors can be challenging to diagnose, especially for beginners, because they might not immediately reveal the true source of the problem.
Code Formatting and Readability
Code formatting and readability are paramount for debugging and maintenance. Well-formatted code is easier to understand and debug, significantly reducing the time spent on error identification. Consistent use of indentation, proper spacing, and a clear structure makes the code more accessible. A simple example is using meaningful variable names to avoid ambiguity. For example, `userAge` is better than `age`.
Best Practices for Clean and Well-Structured Code
Proper formatting and structuring of code are crucial for both readability and maintainability. This table highlights common pitfalls and best practices.
Pitfall | Best Practice | Example (Python) |
---|---|---|
Missing colons (:) after statements like `if`, `for`, `while` | Always include colons to indicate the start of a code block. |
if x > 5 print("x is greater than 5") <-- Incorrect if x > 5: print("x is greater than 5") <-- Correct |
Incorrect indentation | Use consistent indentation (typically 4 spaces) to define code blocks. |
def my_function(): print("Hello") <-- Incorrect indentation def my_function(): print("Hello") <-- Correct indentation |
Unnecessary semicolons (;) | Avoid unnecessary semicolons, particularly in languages like Python where they're not required. |
print("Hello"); <-- Unnecessary semicolon in Python print("Hello") <-- Correct Python syntax |
Inconsistent naming conventions | Use consistent naming conventions for variables, functions, and classes. |
myVariable = 10 MyFunction() my_variable = 10 my_function() |
Debugging and Troubleshooting
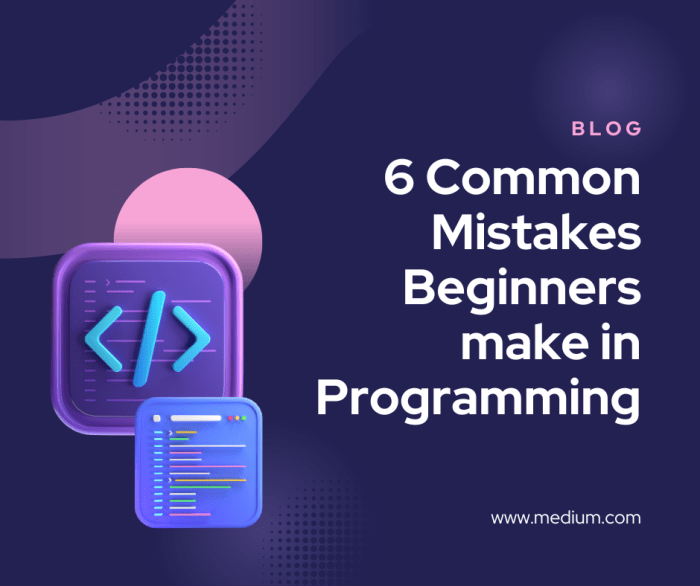
Source: medium.com
Debugging is a crucial aspect of programming. It's the process of identifying and resolving errors (bugs) in your code.
A significant portion of a programmer's time is often dedicated to debugging, highlighting the importance of developing robust debugging skills early on. Effective debugging leads to more reliable and efficient software.
Effective debugging requires a structured approach and a keen eye for detail. A systematic method for identifying and resolving errors is essential. This section will detail common debugging strategies, tools, and pitfalls beginners encounter, along with techniques for pinpointing the source of errors.
Debugging Process
A methodical debugging process helps in isolating the root cause of errors. The process involves systematically examining the code, testing assumptions, and refining the approach. A common approach is to follow a series of steps:
- Identify the problem: Carefully review the program's output or behavior. Note exactly what is wrong, what the expected output should be, and what the actual output is. This precise definition of the problem is critical for focusing the debugging efforts.
- Isolate the error: Narrow down the area of the code where the error is occurring. Use print statements or logging to trace the flow of execution and identify the point where the program deviates from the expected behavior. Start with the most likely area.
- Analyze the error: Examine the error messages, stack traces, and any other relevant information. If there are error messages, note the specific error types and the lines of code where they occur. Understand the context surrounding the error.
- Develop a solution: Based on the analysis, devise a potential fix for the error. Consider alternative approaches if the initial solution doesn't work. Be mindful of potential side effects.
- Test the solution: Implement the proposed solution and thoroughly test the program to ensure the bug is resolved. Run various test cases to verify the fix.
Common Debugging Strategies
Various strategies are used to pinpoint the source of errors in code. These techniques range from simple to sophisticated, each offering unique advantages in different situations.
- Print statements (or logging): Inserting print statements (or logging calls) at strategic points in the code to display the values of variables and intermediate results. This allows tracing the execution flow and identifying the problematic step. Print statements provide an immediate way to inspect variables and their values at specific points in the code's execution.
- Step-by-step execution (debuggers): Using a debugger to step through the code line by line, examining the values of variables at each step. Debuggers allow you to examine variables, evaluate expressions, and set breakpoints to halt execution at specific points in the code.
- Testing and Unit testing: Writing specific tests for sections of code (unit tests) to isolate potential bugs. Unit tests verify the functionality of individual parts of the program, enabling quick identification of errors. Unit tests help to ensure that each module of code works as intended.
Common Beginner Errors and Solutions
Beginners often encounter specific types of errors during debugging. Understanding these common errors and their solutions will help in the troubleshooting process.
- Incorrect variable usage: Using variables before they are initialized, or referencing variables outside their scope. Ensure that variables are declared and initialized correctly, and are used within their defined scope.
- Type mismatch errors: Trying to perform operations on variables of incompatible types. Carefully check data types and use appropriate conversion functions if necessary. Ensure that the data types of variables involved in operations are compatible.
- Logical errors: Mistakes in the logic of the program, leading to unexpected behavior. These errors can be hard to spot, and often require careful analysis of the code's flow and expected results. A detailed review of the program's logic is often required to identify and fix logical errors.
Methods for Pinpointing Root Cause
Pinpointing the root cause of errors requires a systematic and analytical approach. This often involves tracing the program's execution and examining the values of variables at critical points.
- Review the code carefully: Step through the code line by line, and look for inconsistencies, missing steps, or areas where assumptions may be incorrect. This careful review is often the first step in finding the root cause.
- Use debugging tools: Employ debugging tools to monitor the flow of execution, and to inspect the values of variables. Tools like debuggers can provide valuable insight into the program's behavior.
- Simplify the code: If the code is complex, temporarily remove parts of it to isolate the problem area. This helps in understanding the specific part of the code causing the issue.
Logic and Algorithm Design
A fundamental aspect of programming is crafting algorithms that translate problems into executable steps. Effective algorithm design is crucial for producing robust, efficient, and maintainable code. This section delves into common logical pitfalls beginner programmers encounter and underscores the importance of meticulous algorithm design for successful programming.
Common Logical Errors
Logical errors in programs are subtle flaws in the program's logic that don't prevent compilation but lead to incorrect results. Novice programmers often make these errors due to incomplete or inaccurate understanding of the problem's requirements.
- Incorrect Conditional Statements: Using incorrect or incomplete conditions in `if-else` statements can lead to unintended outcomes. For example, failing to account for all possible cases in a nested `if-else` structure might result in a program that doesn't handle some input correctly.
- Off-by-One Errors: These errors typically arise when dealing with loops or arrays. For instance, iterating one element past the end of an array, or starting the loop from the wrong index, can cause data corruption or unexpected behavior.
- Incorrect Loop Termination Conditions: Misinterpreting or misapplying loop termination conditions, like `while` or `for` loops, can cause infinite loops or the program to skip crucial iterations.
- Incorrect Variable Usage: Misusing or misunderstanding variable types, scopes, and assignments can introduce subtle errors in calculations and data manipulation. For instance, forgetting to initialize a variable or using the wrong variable type for a particular calculation can produce erroneous results.
- Incorrect Data Structures: Using an unsuitable data structure for a given task can lead to inefficient algorithms. Choosing a linked list for an operation that would benefit from an array, for example, will introduce performance issues.
Importance of Clear Algorithm Design
Algorithm design, the process of formulating a step-by-step solution to a problem, is critical to preventing logical errors. A well-defined algorithm translates the problem's requirements into a precise set of instructions, minimizing ambiguity and increasing the likelihood of a correct solution.
- Reduced Errors: A well-structured algorithm acts as a blueprint for the program, ensuring that all possible scenarios are considered and accounted for. This reduces the probability of errors during the programming phase.
- Improved Code Readability: A clear algorithm is easily translated into understandable code. This improves the maintainability and reduces the likelihood of introducing new errors when modifying the code later.
- Enhanced Code Efficiency: An optimized algorithm can often translate to more efficient code. This is crucial in tasks where performance is paramount. For example, finding the shortest path between two points in a map will benefit from algorithms that efficiently traverse the map, compared to algorithms that might require many redundant checks.
Approaches to Problem Solving with Algorithms
Different approaches to problem-solving using algorithms exist, each with its own strengths and weaknesses. The choice of approach often depends on the specific nature of the problem.
- Iterative Approach: This approach involves repeating a set of steps until a desired outcome is achieved. This is often used for problems that can be broken down into a series of repetitive operations. For instance, calculating the factorial of a number is a task well-suited to an iterative approach.
- Recursive Approach: This approach involves defining a problem in terms of itself. It's particularly useful for problems with inherent recursive structures, like traversing a tree or solving a mathematical equation recursively. The Fibonacci sequence is a common example of a recursive problem.
- Divide and Conquer Approach: This method breaks down a complex problem into smaller, more manageable sub-problems. The solution to the original problem is then derived by combining the solutions to the sub-problems. Merge sort is a classic example of this approach.
Impact of Poor Algorithm Design
Poor algorithm design can significantly affect code performance and reliability. The choice of algorithm directly influences how efficiently the program processes data and how accurately it produces results.
- Performance Degradation: Inefficient algorithms can lead to slow execution times, especially when dealing with large datasets. For instance, a program that searches for a specific element in an unsorted list using a linear search will take significantly longer than one using a binary search on a sorted list.
- Reduced Reliability: Poor algorithm design can lead to incorrect results or even program crashes. For instance, an algorithm that incorrectly handles boundary conditions can lead to unexpected output.
- Increased Complexity: Poor algorithm design often leads to complex and hard-to-understand code, making it difficult to maintain and debug. This is because the algorithm itself is convoluted and doesn't cleanly reflect the problem's solution.
Data Structures and Algorithms: Common Mistakes Beginner Programmers Make
Choosing the right data structure is crucial for efficient program execution. The performance of an algorithm often hinges on the underlying data structure. Understanding how different structures store and retrieve data is essential for writing optimized and maintainable code. Selecting an appropriate structure can significantly impact factors like speed, memory usage, and overall program efficiency.
The selection of a data structure depends on the specific needs of the task. Consider factors such as the frequency of data access (reading or writing), the expected size of the dataset, and the types of operations you anticipate performing (insertions, deletions, searches). An unsuitable choice can lead to significant performance bottlenecks, making the program sluggish or even unusable for large datasets.
Selecting the Right Data Structure
Proper data structure selection is paramount for program performance. An algorithm operating on an inappropriate data structure can result in inefficiencies that impact program execution time and memory usage. A well-chosen data structure can optimize resource usage, leading to a smoother and faster program.
Common Data Structure Mistakes
Beginners often make mistakes when selecting and utilizing data structures, leading to performance issues. Understanding these common errors can prevent costly inefficiencies.
- Using the wrong data structure for the task. For example, if you need frequent random access to elements, an array is often a better choice than a linked list. An array provides direct access to any element using its index, while a linked list requires traversal to find a particular element. This can lead to substantial time differences, especially when dealing with large datasets.
- Ignoring the characteristics of the data structure. Linked lists excel at insertion and deletion operations, but searching is inefficient. Trees offer efficient search, but insertions and deletions can be complex. Choosing the wrong structure for the task can significantly impact program performance.
- Inadequate consideration of memory usage. Data structures like hash tables use a significant amount of memory for their internal workings. If memory is a constraint, other structures might be more appropriate. A naive approach might not consider this factor, potentially leading to program crashes.
- Overlooking the complexity of operations. Operations like searching, inserting, and deleting have different time complexities depending on the data structure. Choosing a structure where operations are unnecessarily complex can significantly impact performance. For example, a binary search tree can have O(n) time complexity for insertion and deletion in the worst case, whereas a balanced binary search tree guarantees O(log n) time complexity.
Choosing the wrong structure can dramatically affect performance, especially in large datasets.
Avoiding Common Mistakes
Careful consideration and proper analysis of the task are crucial in avoiding common data structure mistakes. Understanding the characteristics of various data structures can prevent these pitfalls.
- Thoroughly analyze the requirements of the task. Consider the types of data and operations that will be performed most frequently. This analysis will guide you in choosing the appropriate data structure.
- Research different data structures and their characteristics. Understand their strengths and weaknesses in terms of time complexity and memory usage. Understanding the trade-offs will help in selecting the best fit.
- Profiling the code to identify bottlenecks. Analyze how the program utilizes different data structures. Profiling tools can help determine areas where inefficiencies occur.
- Consider alternative data structures. If one data structure is not ideal, explore other options. For instance, if an array's performance isn't sufficient, consider using a hash table or a tree.
Problem-Solving and Approach
Effective problem-solving is crucial for success in programming. It's not just about knowing the syntax; it's about understanding the underlying logic and strategizing how to reach a solution. A methodical approach to breaking down complex problems is essential for creating efficient and maintainable code.
A robust problem-solving strategy involves understanding the problem's nuances, identifying the core requirements, and developing a structured plan to tackle the challenge. This often involves breaking down the problem into smaller, more manageable parts. This approach allows programmers to focus on specific tasks, promoting clarity and efficiency in the coding process.
Common Approaches to Problem Solving
A programmer's toolkit includes various problem-solving approaches. Understanding and applying these strategies leads to more effective and elegant solutions. These strategies often involve identifying patterns, creating algorithms, and testing different solutions.
- Decomposition: Breaking down a complex problem into smaller, more manageable sub-problems is a fundamental technique. This strategy facilitates a more focused approach to development, enabling programmers to tackle challenges in a systematic manner. For instance, creating a user interface involves numerous tasks like designing individual components, handling input, and managing data flow. Each of these sub-problems can be addressed individually.
- Pattern Recognition: Identifying recurring patterns and structures in problems can streamline the solution process. This technique is often coupled with decomposition, as identifying patterns within sub-problems can simplify their solutions. This method is valuable in situations where the problem's requirements share similar elements.
- Algorithm Design: Creating a step-by-step procedure to solve a problem, or algorithm, is vital for translating a solution into code. This often involves pseudocode or flowcharts for clarity and efficiency. For example, sorting a list of numbers might involve algorithms like merge sort or quick sort, each with specific steps and potential advantages.
Common Misconceptions
Some common misconceptions about problem-solving in programming include relying solely on memorization rather than understanding, or thinking that a single solution exists for every problem. Understanding the rationale behind each solution is crucial.
- Over-reliance on memorized solutions: Instead of simply recalling existing code, a strong programmer focuses on understanding the underlying principles and adapting those to new scenarios. This adaptable approach leads to more flexible and robust solutions.
- The illusion of a single solution: Often, multiple valid solutions exist for a given problem. A skilled programmer can evaluate these solutions based on factors like efficiency, clarity, and maintainability, and select the most appropriate one.
Breaking Down Complex Problems
Breaking down complex problems into smaller, manageable parts is essential for effective programming. This technique, known as decomposition, aids in the organization and implementation of the solution.
- Identify the core problem: Begin by pinpointing the core challenge that needs addressing. This step is vital for focusing on the necessary aspects of the problem and avoiding extraneous details.
- Decompose into sub-problems: Break the core problem into smaller, more manageable sub-problems. This process often involves identifying the various tasks that need to be performed to achieve the overall goal. For instance, creating a web application might involve tasks such as creating a database, designing user interfaces, and developing backend logic.
- Prioritize sub-problems: Arrange the sub-problems in a logical order based on their dependency and complexity. This ensures that the tasks are addressed in a coherent and efficient manner. For instance, a user interface often depends on backend logic, meaning the latter should be addressed first.
Novice vs. Experienced Programmer Approach
The following table highlights the difference in approach between a novice and an experienced programmer when confronted with a problem.
Characteristic | Novice Programmer | Experienced Programmer |
---|---|---|
Problem Understanding | Focuses on the immediate details, overlooking the bigger picture. | Analyzes the problem holistically, identifying underlying patterns and requirements. |
Solution Approach | Tries to find a single, pre-existing solution, or a solution from example code. | Develops a custom solution based on understanding the problem's core principles. |
Decomposition | May not break down complex problems into sub-problems. | Effectively breaks down complex problems into smaller, manageable parts. |
Testing | Limited testing, often relying on ad hoc methods. | Employs thorough testing strategies to validate solutions and ensure robustness. |
Using Libraries and Frameworks
Libraries and frameworks are pre-built collections of code that provide ready-made solutions for common programming tasks. Leveraging these tools significantly boosts development speed and reduces the time spent on repetitive coding. Understanding how to effectively integrate them is crucial for proficient programming.
Libraries and frameworks often offer optimized implementations of algorithms, data structures, and other functionalities. This not only saves development time but also generally leads to more robust and efficient code. However, improper integration can introduce subtle bugs or inefficiencies. Choosing the right library or framework for a specific task is essential for successful development.
Effective Library Utilization
Utilizing external libraries effectively involves a careful process of selection, installation, and integration. Understanding the library's purpose, capabilities, and limitations is crucial before implementation. Thorough documentation review is vital to grasp the API (Application Programming Interface) and understand how to interact with the library's functionalities.
Common Integration Mistakes, Common mistakes beginner programmers make
Improper installation or configuration of libraries can lead to errors. Failure to follow the installation instructions provided by the library or framework documentation can lead to incompatibility issues. Another common pitfall is not properly understanding the library's API, resulting in incorrect usage and unforeseen behavior.
Choosing the Right Library/Framework
Selecting the appropriate library or framework depends on the specific task. Consider factors such as the project's requirements, the language being used, the team's familiarity with the library, and the performance characteristics of the library.
Common Mistakes in Specific Libraries
Library/Framework | Common Mistake | Explanation |
---|---|---|
React (JavaScript) | Incorrect component structure | Creating complex components that are not reusable or following the component structure guidelines of React can lead to difficulty maintaining the code. |
NumPy (Python) | Incorrect array operations | Improper usage of array operations (e.g., incorrect indexing or dimension handling) can result in errors and incorrect calculations. |
Django (Python) | Incorrect model design | Designing models that do not reflect the database structure accurately or do not meet the project requirements can cause issues with data manipulation and integrity. |
Spring Boot (Java) | Incorrect dependency injection | Failure to correctly configure dependency injection can lead to unexpected behavior, errors in dependency resolution, and potential runtime exceptions. |
Advanced Considerations
Libraries and frameworks frequently have dependencies. Understanding these dependencies is critical to ensuring the correct functionality of the project. Proper dependency management is important to avoid conflicts or missing components. Consider version compatibility when integrating external libraries or frameworks to ensure they work seamlessly with the project's other components.
Version Control
Version control systems, like Git, are essential tools for managing code, especially in collaborative projects. They track changes to files over time, allowing developers to revert to previous versions, collaborate effectively, and manage different versions of a project. This meticulous record-keeping is crucial for maintaining a project's integrity and for efficient troubleshooting.
The Role of Version Control Systems
Version control systems meticulously record every change made to the codebase. This historical record enables developers to retrace steps, identify bugs, and revert to stable previous versions if needed. Crucially, it fosters collaboration by allowing multiple developers to work on the same project concurrently, resolving conflicts and integrating changes smoothly. This prevents the common problem of losing work or merging incompatible changes.
Common Errors Beginners Make
Beginners sometimes overlook the importance of committing changes frequently. Failing to commit regularly can lead to lost work or make it difficult to track the evolution of the project. Another common pitfall is neglecting descriptive commit messages. Vague or absent commit messages hinder understanding of changes and make it harder for others (or future you) to comprehend the project's progression.
Finally, a lack of understanding of branching strategies can lead to merging conflicts and complications when integrating new features or fixing bugs.
Importance of Commit Messages
Comprehensive commit messages are paramount for effective version control. They provide context about the changes made, enabling developers to quickly understand the rationale behind modifications. Well-structured commit messages, following conventions like the use of present tense and clear descriptions, make the code history readable and manageable. Example: "Fix: Corrected calculation error in function 'calculateArea'." Such detail helps avoid confusion and allows easier understanding for those reviewing the code or even the developer who may have forgotten the specific issue months later.
Branching Strategies
Branching strategies are essential for managing different features or bug fixes without disrupting the main codebase. A common strategy is the "feature branch" approach, where developers create a new branch for each new feature, develop it independently, and then merge it back into the main branch once complete. This isolates changes and allows for parallel development, mitigating potential conflicts.
Another strategy is the "gitflow" approach, which offers a more structured workflow, especially in larger teams.
Collaborative Coding
Version control systems facilitate seamless collaboration among developers. They allow multiple developers to work on the same project concurrently, with the system managing conflicts and merging changes. This fosters efficient teamwork, enabling teams to work simultaneously on various aspects of the project, accelerating development while maintaining a cohesive codebase. Version control ensures that every developer's contribution is integrated effectively, building upon each other's work.
Testing and Quality Assurance
Testing and quality assurance are crucial components of the software development lifecycle. Robust testing minimizes bugs and ensures that the software functions as intended, leading to a higher quality final product. Thorough testing saves time and resources in the long run by catching errors early, reducing costly rework and improving user experience.
Importance of Writing Unit Tests
Unit tests are crucial for verifying the correctness of individual units of code, such as functions or methods. They help isolate and test specific functionalities, making it easier to identify and fix errors. Writing unit tests early in the development process significantly improves code maintainability and reduces the risk of introducing regressions when making changes to the codebase.
They act as a safety net, preventing errors from creeping into larger parts of the application.
Common Mistakes in Writing Effective Unit Tests
Testing frequently involves creating test cases that demonstrate expected results for different input values. Poorly written tests often lack comprehensive coverage of various input scenarios. Testing only for happy paths (normal input) while neglecting edge cases (unusual or invalid input) can lead to hidden bugs. Unclear or overly complex test setups can make tests difficult to understand and maintain.
Furthermore, tests should be independent and not rely on other parts of the system, ensuring isolation and focused testing.
Benefits of Thorough Testing and Debugging in Preventing Errors
Thorough testing and debugging are vital for preventing errors and improving software quality. Early detection of bugs often reduces the effort and cost associated with fixing them later in the development cycle. Comprehensive testing helps identify potential issues before they impact users, leading to a more reliable and user-friendly product. Proper testing also helps in understanding the codebase better and aids in maintaining the code in the long run.
Creating and Running Test Cases
Creating effective test cases involves defining clear test inputs, expected outputs, and assertions to verify the correctness of the output. Consider various scenarios, including normal inputs, boundary conditions, and error conditions. Testing frameworks such as JUnit (Java), pytest (Python), or Mocha (JavaScript) provide structure and tools to automate test execution. These frameworks allow you to define test functions, which execute the code under test and compare the results with the expected outcomes.
These frameworks often have assertions that help verify the results, such as `assertEqual` for checking equality, `assertTrue` for checking true conditions, and `assertFalse` for checking false conditions. Test cases can be grouped into test suites to run a collection of tests. Running test cases is straightforward with the framework, and test results are usually reported in a structured format, showing whether tests passed or failed.
Tools provide detailed reports that highlight failures and the specific input values that caused the issue, which is crucial for debugging. Examples of different types of test cases include unit tests for individual functions, integration tests for interactions between modules, and system tests for the entire system. These tests should be designed to exercise various parts of the code, ensuring comprehensive coverage.
Final Review
In conclusion, avoiding common mistakes is key to success in programming. By understanding the fundamentals, mastering syntax, and refining your debugging and problem-solving skills, you can significantly enhance your programming abilities. This guide provides a structured approach to identify and correct these errors, helping you progress smoothly and efficiently in your programming journey. Remember, practice and persistence are essential components of mastering this craft.
Post Comment