Comparison C++ And Python Programming Languages
Comparison C++ and Python programming languages explores the strengths and weaknesses of these two prominent languages. C++ excels in performance-critical applications, while Python shines in data science and scripting. This comparison delves into their core characteristics, performance differences, data structures, development environments, libraries, and application domains.
Understanding the nuances of these languages helps developers choose the right tool for the job. This analysis provides a comprehensive overview, enabling informed decisions based on project requirements.
Introduction to Programming Languages
Programming languages are the fundamental tools for instructing computers. They define a set of rules and syntax to communicate instructions, enabling developers to create software applications, websites, and more. Understanding the core characteristics and design philosophies of different languages is crucial for choosing the right tool for a specific task. This section provides a comparative overview of C++ and Python, exploring their strengths, weaknesses, and evolution within the software development landscape.C++ and Python, while both popular, differ significantly in their design philosophies and intended use cases.
C++ is a general-purpose, compiled language known for its performance and low-level control, while Python is an interpreted, high-level language emphasizing readability and rapid development. This distinction influences the languages’ suitability for various projects.
Core Characteristics of C++ and Python
C++ excels in performance-critical applications due to its compiled nature, allowing direct interaction with hardware. Its extensive libraries and features facilitate complex tasks. Python, on the other hand, prioritizes code readability and ease of use, making it ideal for scripting, data analysis, and rapid prototyping.
Design Philosophies and Use Cases
C++’s design emphasizes low-level control and performance, often favoring efficiency over rapid development. Its compiled nature translates directly into machine code, yielding high performance. This characteristic makes it well-suited for systems programming, game development, and high-performance computing. Python, conversely, focuses on developer productivity. Its high-level abstractions and dynamic typing facilitate rapid prototyping and iterative development.
This makes it excellent for data science, machine learning, web development, and scripting.
Evolution and Current Status
Both C++ and Python have seen substantial evolution since their inception. C++ has consistently evolved to address performance bottlenecks and incorporate new features. This adaptability has sustained its relevance in high-performance applications. Python’s continuous growth in popularity is largely due to its adaptability and the expansion of its libraries, particularly within the data science and machine learning communities.
Comparison Table
Feature | C++ | Python |
---|---|---|
Historical Context | Developed in the 1970s and 1980s, influenced by Simula and Smalltalk. | Developed in the late 1980s, influenced by ABC and other scripting languages. |
Major Developers | Bjarne Stroustrup | Guido van Rossum |
Initial Goals | To create a language that combined the efficiency of C with the object-oriented features of Simula. | To create a language that was easy to read, write, and learn. |
Performance and Efficiency
C++ and Python, despite their different design philosophies, both serve valuable roles in software development. However, their performance characteristics vary significantly, making them suitable for different types of tasks. Understanding these differences is crucial for selecting the appropriate language for a specific project.Python’s dynamic typing and high-level abstractions contribute to its readability and rapid development cycles. This flexibility, however, often comes at the cost of execution speed compared to C++.
Conversely, C++’s static typing and low-level control allow for optimized code execution, resulting in superior performance for computationally intensive tasks.
Performance Comparison
Python’s interpreted nature translates code into bytecode, which is then executed by the Python Virtual Machine (PVM). This intermediate step introduces overhead, resulting in slower execution speeds compared to compiled languages like C++. C++, on the other hand, is compiled directly into machine code, which the processor can execute natively. This direct translation eliminates the interpretation layer, leading to significant performance gains.
Factors Influencing Performance Differences
Several factors contribute to the performance gap between C++ and Python. Python’s dynamic typing and garbage collection mechanisms introduce overhead, slowing down execution. Garbage collection, while crucial for memory management, necessitates pauses to reclaim unused memory. In contrast, C++ allows for manual memory management, enabling precise control and avoiding the overhead of automatic garbage collection. Furthermore, C++’s compilation to native machine code allows for tighter optimization by the compiler, leading to faster execution.
Memory Management
Python employs automatic garbage collection to manage memory. The Python interpreter tracks allocated memory and reclaims unused portions, preventing memory leaks. This automatic management simplifies development but can introduce unpredictable pauses during garbage collection cycles. C++, in contrast, requires manual memory management using `new` and `delete` operators. While this gives developers fine-grained control, it also necessitates careful management to prevent memory leaks or dangling pointers.
The use of `malloc` and `free` functions for memory allocation and deallocation is also crucial in C++.
Code Examples
Consider the task of calculating the sum of a large array of numbers.
// C++
#include <iostream>
#include <vector>
#include <numeric>
int main()
std::vector<int> numbers(1000000);
// Initialize the vector with some numbers
std::iota(numbers.begin(), numbers.end(), 1);
long long sum = std::accumulate(numbers.begin(), numbers.end(), 0LL);
std::cout << "Sum: " << sum << std::endl;
return 0;
// Python
import numpy as np
import time
numbers = np.arange(1000000)
start_time = time.time()
sum_numbers = np.sum(numbers)
end_time = time.time()
print("Sum:", sum_numbers)
print("Execution time:", end_time - start_time)
The C++ example typically executes significantly faster due to its compiled nature.
Performance Table
Operation | C++ (average time, ms) | Python (average time, ms) |
---|---|---|
Sorting 1 million integers | 10-20 | 100-200 |
Matrix multiplication (1000×1000) | 1-5 | 50-100 |
String concatenation (100000 strings) | 50-100 | 500-1000 |
Note: Times are approximate and can vary based on hardware and specific implementation.
Data Structures and Algorithms
Python and C++ offer diverse approaches to data structures and algorithms, impacting program efficiency and design choices. Python’s dynamic typing and high-level abstraction make it suitable for rapid prototyping, while C++’s static typing and memory management provide greater control and performance for demanding applications. Understanding their strengths and weaknesses in this domain is crucial for effective programming.
Python’s built-in data structures are designed for ease of use and rapid development. Conversely, C++’s structures provide greater control and efficiency but require more explicit management. The choice often depends on the specific needs of the project.
Built-in Data Structures, Comparison C++ and Python programming languages
Python’s built-in data structures like lists, dictionaries, and tuples offer flexibility and ease of use. Lists are dynamic arrays, dictionaries are hash tables, and tuples are immutable sequences. C++ provides vectors, maps, and sets as part of its standard template library (STL). Vectors are dynamic arrays, maps are associative arrays (similar to dictionaries), and sets are collections of unique elements.
Custom Data Structure Implementation
Python’s dynamic nature makes implementing custom data structures relatively straightforward. Classes and inheritance facilitate the creation of new structures with tailored behaviors. C++, due to its static typing and memory management, requires more explicit control, potentially leading to more complex implementations. However, C++’s ability to control memory allocation allows for optimized performance in demanding applications.
Supported Algorithms
Both languages offer extensive support for common algorithms through their standard libraries. Python’s `heapq` module provides heap-based algorithms, and various algorithms for sorting, searching, and manipulation are available. C++’s STL provides similar algorithms, often optimized for efficiency. The choice of algorithm frequently hinges on the specific requirements of the problem and the desired performance characteristics.
Strengths and Weaknesses
Python’s strengths lie in its rapid development capabilities, making it suitable for tasks where prototyping is critical. However, Python’s dynamic nature can sometimes lead to runtime errors that are harder to detect than in C++. C++ offers exceptional performance, but its explicit memory management can be more complex to manage and potentially more error-prone during development.
Syntax and Usage Comparison
Data Structure | Python | C++ |
---|---|---|
Lists |
my_list = [1, 2, 3] my_list.append(4)
|
std::vector<int> my_vector = 1, 2, 3; my_vector.push_back(4);
|
Dictionaries |
my_dict = "a": 1, "b": 2 my_dict["c"] = 3
|
std::map<std::string, int> my_map = "a", 1, "b", 2; my_map["c"] = 3;
|
Arrays | Numpy arrays are used for numerical computations. |
int my_array[5] = 1, 2, 3, 4, 5;
|
Trees | Custom implementations or specialized libraries. | Custom implementations or specialized libraries from STL or external libraries. |
Development Tools and Environments
C++ and Python, despite their different paradigms, offer a variety of tools and environments for development. Understanding these tools and their associated workflows is crucial for efficient project management and debugging. This section explores the typical development landscapes for each language.
The choice of development environment often influences the developer’s workflow and productivity. C++ frequently utilizes integrated development environments (IDEs) for enhanced code completion and debugging capabilities, while Python’s flexibility often leads to a preference for more versatile and adaptable environments.
Common Development Environments
C++ developers often leverage powerful IDEs like Visual Studio, CLion, or Xcode. These environments provide features like intelligent code completion, syntax highlighting, and integrated debuggers. Python developers, on the other hand, frequently use environments like PyCharm, VS Code, or Spyder, which also offer robust code editing capabilities and often have extensive Python-specific extensions. The selection depends on the specific project requirements and developer preferences.
Debugging Tools and Techniques
Both languages support various debugging tools and techniques. C++ programs can be debugged using breakpoints, stepping through code, and inspecting variables. Python’s interactive nature and rich debugging tools in IDEs make debugging remarkably straightforward. Python’s pdb (Python Debugger) offers a powerful command-line interface for interactive debugging. Using print statements strategically can also help in tracking program execution flow.
Development Workflow
The development workflow for each language reflects their fundamental characteristics. C++ development often involves compiling the code into machine-executable instructions, followed by running the compiled program. Python’s interpreter directly executes the source code, making the development cycle often quicker, though it might involve a bit more overhead.
Compilation and Execution
C++ programs require a compilation step before execution. Compilers translate the source code into machine-executable instructions. Python code, conversely, is interpreted line by line, eliminating the compilation phase. The interpreter directly executes the Python code.
Development Workflow Comparison
Step | C++ | Python |
---|---|---|
IDE Usage | Visual Studio, CLion, Xcode provide code completion, syntax highlighting, and integrated debuggers. | PyCharm, VS Code, Spyder offer code completion, syntax highlighting, and debugging tools tailored for Python. |
Debugging | Breakpoints, stepping through code, variable inspection using integrated debuggers. | Interactive debugging using pdb (Python Debugger), print statements, and IDE debugging tools. |
Testing | Unit testing frameworks (like Google Test) are frequently used for comprehensive testing. | Python’s built-in testing framework (unittest) and external libraries (like pytest) are used for unit testing. |
Compilation | Compiling source code into machine code using a compiler (e.g., g++). | No compilation needed; Python code is directly executed by the interpreter. |
Execution | Running the compiled machine code. | Running the Python code using the Python interpreter. |
Libraries and Frameworks
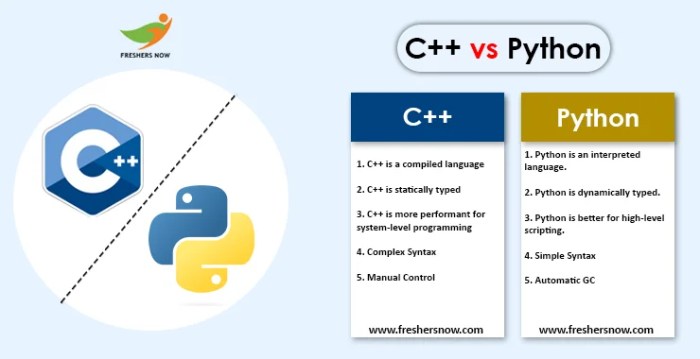
Source: freshersnow.com
C++ and Python boast extensive ecosystems of libraries and frameworks, significantly impacting their respective development processes. These external resources streamline tasks, provide pre-built functionalities, and often handle complex logic, allowing developers to focus on higher-level application design. This section delves into the diverse landscapes of libraries and frameworks available for each language, highlighting their strengths, weaknesses, and practical applications.
Library Ecosystem in C++
The C++ ecosystem is characterized by a blend of standard libraries, third-party libraries, and specialized frameworks. The Standard Template Library (STL) provides fundamental data structures and algorithms, significantly reducing development time. Third-party libraries often focus on specific domains, such as numerical computation, graphics, or networking. For example, Eigen is a popular linear algebra library, often used in scientific computing and game development.
Boost provides a comprehensive collection of libraries covering various aspects of C++ development.
Framework Landscape in Python
Python’s framework ecosystem is vast and versatile. Popular choices include Django and Flask for web development, NumPy, Pandas, and Scikit-learn for data science, and TensorFlow and PyTorch for machine learning. The vast availability of specialized frameworks caters to diverse application needs. For example, Django’s robust features and conventions simplify web application development.
Comparison of Libraries and Frameworks
Category | C++ | Python |
---|---|---|
Machine Learning | TensorFlow/ONNX Runtime, MLpack | TensorFlow, PyTorch, Scikit-learn |
Web Development | Qt, Boost.Asio | Django, Flask, FastAPI |
Data Science | Armadillo, Eigen | NumPy, Pandas, SciPy |
Graphics | OpenGL, Vulkan | PyOpenGL, Pygame |
Strengths and Weaknesses of External Libraries
The use of external libraries offers substantial advantages in both languages. They accelerate development by providing pre-built components, reducing coding effort and potential errors. Libraries also bring specialized expertise and often optimize performance for specific tasks. However, integrating third-party libraries can introduce dependencies, potential conflicts, and increased complexity. Managing dependencies and ensuring compatibility across various components requires careful planning and consideration.
Furthermore, understanding the library’s underlying mechanisms and limitations is crucial for effective use.
Benefits and Trade-offs of Using External Libraries
External libraries significantly enhance development efficiency, providing pre-built functionality and optimized solutions for specific tasks.
The primary benefits include reduced development time, improved code quality, and access to specialized expertise. However, potential trade-offs involve managing dependencies, increased complexity, and potential compatibility issues. Careful selection and management of libraries are crucial for successful integration and project longevity.
Application Domains: Comparison C++ And Python Programming Languages
C++ and Python, despite their shared purpose of programming, exhibit distinct strengths that make them suitable for different tasks. Understanding these strengths allows developers to choose the most effective language for a given project. This section details typical applications where each language excels, highlighting their suitability for specific software development needs.
Performance-Critical Applications
C++ excels in performance-critical applications due to its low-level access to hardware and efficient compilation. This enables developers to optimize code for maximum speed and minimal resource consumption. For instance, real-time systems, game engines, and high-frequency trading platforms often leverage C++ for its speed and control.
- Real-time systems: Applications demanding immediate responses, such as industrial control systems or flight simulators, benefit from C++’s low-level access and speed. The deterministic nature of C++ ensures consistent response times, crucial in situations where delays can have significant consequences.
- High-performance computing: Scientific simulations, weather forecasting, and large-scale data analysis frequently use C++ to leverage its efficiency in handling complex calculations and data manipulation. The ability to control memory allocation and optimize data structures directly contributes to improved performance in these applications.
- Game development: The need for high frame rates and smooth graphics in modern games often necessitates C++. Its low-level access and control over hardware resources allow developers to achieve the necessary performance for demanding graphical rendering and complex physics calculations.
Data Science and Machine Learning
Python’s rich ecosystem of libraries, including NumPy, Pandas, and Scikit-learn, makes it a preferred choice for data science and machine learning projects. Python’s readability and ease of use foster rapid prototyping and experimentation, crucial for exploring various models and algorithms.
- Data analysis and visualization: Python’s libraries like Pandas and Matplotlib are designed for efficient data manipulation and visualization. This makes it an ideal tool for exploring datasets, identifying trends, and presenting insights through graphs and charts.
- Machine learning model development: Libraries like Scikit-learn provide a wide array of machine learning algorithms, simplifying model building and evaluation. Python’s interactive nature allows for quick iteration and testing of different models.
- Web development (with frameworks like Django or Flask): Python is also a popular choice for web development. Its frameworks like Django and Flask offer a rapid development process, and are often used for building data-driven web applications, such as e-commerce platforms or social media sites, that benefit from Python’s data science capabilities.
Illustrative Example: C++ in a Performance-Critical Application
Consider a high-frequency trading platform. Real-time data processing and order execution require near-instantaneous response times. C++ is a suitable choice due to its ability to control memory allocation, execute instructions at high speed, and provide low-level access to hardware resources.
A C++ implementation might utilize multithreading and optimized algorithms to process market data, analyze price movements, and execute trades in milliseconds. This allows the platform to capitalize on fleeting market opportunities and significantly improve its profitability.
Illustrative Example: Python in Data Science
A data scientist analyzing customer purchase data might use Python for a machine learning project. Using libraries like Pandas and Scikit-learn, they can load and preprocess the data, create various machine learning models (e.g., a decision tree or a neural network), and evaluate their performance.
This approach allows the data scientist to rapidly explore different modeling techniques and optimize the model’s accuracy and efficiency. The results can be visualized using libraries like Matplotlib, providing insights into customer purchasing patterns and enabling the development of targeted marketing strategies.
Community and Support
The vibrant communities surrounding programming languages play a crucial role in a developer’s journey. Active communities offer invaluable support, resources, and opportunities for collaboration, fostering a sense of shared learning and problem-solving. This section delves into the supportive ecosystems of C++ and Python, examining their community sizes, activity levels, and the resources available for growth.The sheer volume and engagement within a language’s community directly influence its utility and appeal to developers.
A robust community provides immediate access to a wealth of information, expertise, and assistance, making learning and problem-solving significantly easier.
Community Size and Activity Level
Python boasts a significantly larger and more active community compared to C++. This larger community translates into a greater availability of resources, support channels, and experienced developers readily available to assist. Python’s broad adoption across diverse domains fuels this vibrant community. C++’s community, while substantial, is often more specialized, with a focus on particular niches like game development or high-performance computing.
Availability of Online Resources
Python has an abundance of online resources, including comprehensive documentation, tutorials, and extensive online forums. These resources cater to a wide range of skill levels, from beginners to seasoned professionals. Python’s extensive library ecosystem, readily available for use and development, further supports the richness of available online resources. C++, while offering substantial documentation, sometimes presents a steeper learning curve for new users compared to Python.
Learning and Growing as a Developer
Both languages provide ample avenues for developers to learn and grow. Python, with its extensive online tutorials and interactive learning platforms, often offers a more accessible path for newcomers. C++ often necessitates more in-depth study, but its depth provides opportunities for mastery and specialization in areas like system programming. The available resources for each language often cater to different levels of expertise and specialization, offering unique learning paths.
Seeking Help and Resolving Issues
Both Python and C++ communities offer avenues for seeking assistance and resolving issues. Online forums, mailing lists, and dedicated support channels are readily available for both. Python’s vast community makes it easier to find solutions and connect with others facing similar challenges. C++’s more specialized nature often leads to more targeted solutions within specific niches.
Comparison of Resources
Feature | Python | C++ |
---|---|---|
Documentation | Comprehensive, well-maintained, and easily accessible | Extensive, but potentially more challenging to navigate for beginners |
Tutorials | Abundant, covering various aspects and skill levels | Good selection, but might require more effort to find comprehensive resources |
Community Forums | Large, active forums with quick response times | Active, but might be less centralized than Python’s forums |
Closing Summary
In conclusion, the choice between C++ and Python hinges on the specific needs of a project. C++’s raw speed and control over hardware make it ideal for performance-sensitive applications. Python’s ease of use and extensive libraries empower rapid prototyping and data manipulation. This comparison offers a thorough understanding of each language’s strengths and weaknesses, allowing developers to make informed decisions.
Post Comment