Javascript Vs Typescript Key Differences And Comparison
JavaScript vs TypeScript key differences and comparison delves into the nuances of these two prominent JavaScript ecosystems. JavaScript, a versatile scripting language, powers interactive web elements, while TypeScript builds upon JavaScript with static typing, enhancing code organization and maintainability. Understanding their core distinctions is crucial for developers seeking to choose the best tool for a project.
This exploration will examine the typing systems, object-oriented programming support, development workflows, community resources, performance, use cases, and practical examples to provide a comprehensive understanding of each language’s strengths and weaknesses.
Introduction to JavaScript and TypeScript
JavaScript is a dynamic, interpreted programming language primarily used for front-end web development. It powers the interactive elements of websites, enabling users to interact with web pages in real-time. Its flexibility and widespread adoption have made it a cornerstone of modern web development. TypeScript, on the other hand, is a superset of JavaScript, adding static typing to enhance code maintainability and scalability.TypeScript builds upon JavaScript’s strengths while addressing its limitations, particularly in larger projects.
The relationship between the two languages is symbiotic, with TypeScript providing a more structured and organized approach to JavaScript development, enabling developers to write cleaner and more robust applications.
JavaScript Definition
JavaScript is a dynamic, weakly-typed, prototype-based scripting language primarily used for front-end web development. It is widely adopted and versatile, capable of handling diverse tasks beyond basic web interactions, including server-side programming (Node.js) and mobile app development (React Native).
TypeScript Definition
TypeScript is a typed superset of JavaScript. It extends JavaScript by adding optional static typing, interfaces, classes, and modules, which promote code organization and reduce runtime errors. These features enhance maintainability and scalability, particularly in larger projects.
Relationship between JavaScript and TypeScript
TypeScript is a superset of JavaScript. This means that any valid JavaScript code is also valid TypeScript code. TypeScript compiles down to JavaScript, allowing developers to leverage the vast existing ecosystem of JavaScript libraries and tools.
Core Philosophy Behind TypeScript’s Design Choices
TypeScript’s design prioritizes developer productivity and maintainability. The addition of static typing aims to catch errors during development, reducing the likelihood of bugs in production code. The language’s design choices are driven by the desire to create a language that allows for the development of large-scale, maintainable applications while still retaining the dynamism and flexibility of JavaScript.
Historical Context of Both Languages
JavaScript, introduced in 1995, quickly became the dominant language for front-end development. Its initial design prioritized speed and simplicity, leading to a flexible but sometimes less structured approach. TypeScript, developed by Microsoft in 2012, emerged as a response to the challenges of managing larger JavaScript projects. The historical context highlights the evolution of front-end development, with TypeScript addressing the growing need for more structured approaches to maintainability and scalability.
Key Features Comparison
Feature | JavaScript | TypeScript | Description |
---|---|---|---|
Typing | Dynamic | Static | JavaScript is dynamically typed, meaning type checking occurs at runtime. TypeScript, however, is statically typed, enabling type checking during development. |
Classes | Prototypal | Classical | JavaScript employs a prototypal inheritance model, while TypeScript supports classical class-based inheritance. |
Modules | Limited or ES6 modules | Modules | JavaScript’s module support was initially limited, but ES6 modules improved this. TypeScript provides a robust module system for organizing code. |
Interfaces | Not available | Available | TypeScript introduces interfaces to define contracts for classes, promoting code consistency and maintainability. |
Typing System
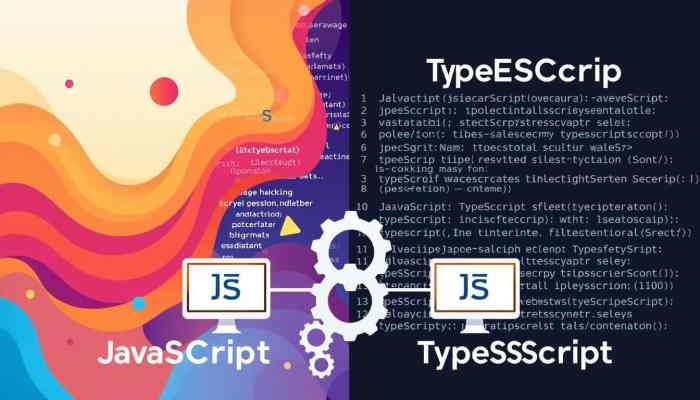
Source: futureappstudios.com
JavaScript’s dynamic typing and TypeScript’s static typing represent a fundamental difference in how they handle data types. This difference directly impacts the way developers write, maintain, and debug code. Understanding the nuances of each system is crucial for making informed choices when selecting a language for a project.TypeScript’s static typing approach, borrowing from languages like Java and C++, demands explicit declarations of variable types.
This foresight allows the compiler to catch potential type-related errors during development, rather than at runtime. JavaScript, on the other hand, relies on dynamic typing, inferring types at runtime. While this flexibility can be appealing for rapid prototyping, it can lead to runtime errors that are harder to track down.
Fundamental Differences
JavaScript’s dynamic typing system allows variables to hold different data types throughout their lifespan. This flexibility comes at a cost; type-related errors are often discovered during runtime, potentially causing unexpected behavior. TypeScript’s static typing demands explicit type declarations, which informs the compiler about the expected types. This enables the compiler to flag type errors during development, significantly improving the quality of the code.
Benefits of TypeScript’s Static Typing
Static typing in TypeScript offers several advantages, including improved code maintainability and a reduction in runtime errors. The compiler’s type checking catches errors early in the development process, which often leads to fewer bugs in production code. The enhanced code clarity provided by explicit types also simplifies the process of understanding and modifying existing code.
Drawbacks of TypeScript’s Static Typing
While static typing is generally beneficial, it does have some drawbacks. The need for explicit type declarations can sometimes increase the amount of boilerplate code, and it can introduce a slight learning curve for developers transitioning from dynamically typed languages like JavaScript. There’s also a potential performance overhead due to the compilation step involved.
Flexibility Comparison
JavaScript’s dynamic typing offers remarkable flexibility, allowing for quick prototyping and rapid development. This freedom can be advantageous in scenarios where the exact data types aren’t immediately apparent or where the structure of the data is subject to change. However, this flexibility can lead to hidden bugs that are only exposed during runtime. TypeScript’s static typing, while more restrictive, promotes code reliability and allows developers to catch errors early in the development cycle, reducing the risk of runtime surprises.
Improved Code Maintainability and Reduced Errors
TypeScript’s static typing significantly enhances code maintainability. The explicit type declarations provide a clear understanding of the intended use of variables and functions, which reduces the chance of misunderstanding or accidental misuse. This enhanced clarity directly contributes to the overall maintainability of the codebase, making it easier to understand, modify, and extend. The early detection of type errors by the TypeScript compiler minimizes the risk of runtime exceptions and helps to produce more robust and reliable applications.
Impact on Development Workflow
The presence of a type system fundamentally alters the development workflow. In TypeScript, developers spend more time during the early stages of development, as the compiler will provide feedback on type-related issues. This upfront investment in type checking, however, significantly reduces the time spent debugging and resolving runtime errors. The result is a more efficient and less error-prone development cycle.
Type System Comparison Table
Feature | JavaScript (Dynamic Typing) | TypeScript (Static Typing) | Example |
---|---|---|---|
Type Declaration | Implicit | Explicit | JavaScript: let age = 30; TypeScript: let age: number = 30; |
Type Checking | Runtime | Compile Time | JavaScript: Error may occur at runtime if age is used inappropriately. TypeScript: Error is caught during compilation if age is used incorrectly. |
Error Detection | Later | Earlier | JavaScript: Error may be found late in the development process. TypeScript: Errors are found during development. |
Maintainability | Potentially lower | Higher | JavaScript: Code may be harder to maintain with dynamic typing. TypeScript: Explicit types improve code clarity and maintainability. |
Object-Oriented Programming (OOP) Support
JavaScript, while capable of object-oriented programming, doesn’t enforce strict adherence to OOP principles. TypeScript, being a superset of JavaScript, builds upon this foundation by providing static typing and class-based structure, thereby enhancing OOP capabilities significantly. This difference affects how objects and classes are implemented and used.Both languages support creating objects and classes, but TypeScript’s type system allows for greater control and safety in object interactions.
This results in more robust and maintainable code, particularly in larger projects.
Class Definition and Implementation
JavaScript uses prototypes to achieve object-oriented behavior. Classes in JavaScript are essentially syntactic sugar over prototypes, offering a more structured way to define objects. This approach is less strict than TypeScript’s classes. TypeScript, conversely, defines classes using a syntax similar to other OOP languages like Java or C++. This enables compile-time checking of class structures and method signatures, preventing common errors before runtime.
Object Instantiation and Usage
JavaScript objects are instantiated through constructors, often defined as functions. Methods are added to the prototype of the constructor. TypeScript’s class-based approach provides a clearer separation of concerns. Objects are instantiated from class definitions, enforcing the structure and type safety inherent in the class design.
TypeScript’s Enhanced OOP Capabilities
TypeScript’s static typing significantly enhances OOP capabilities. By specifying types for class members, TypeScript helps prevent common errors. This compile-time checking allows developers to catch potential issues early in the development process, leading to more robust and maintainable code. The strict typing extends to method signatures, parameter types, and return types, leading to better code clarity and reduced ambiguity.
Examples of OOP in Both Languages
JavaScript
“`javascriptfunction Car(make, model) this.make = make; this.model = model;Car.prototype.honk = function() console.log(“Beep!”);;const myCar = new Car(“Toyota”, “Camry”);myCar.honk();“`
TypeScript
“`typescriptclass Car make: string; model: string; constructor(make: string, model: string) this.make = make; this.model = model; honk(): void console.log(“Beep!”); const myCar = new Car(“Toyota”, “Camry”);myCar.honk();“`
Comparison Table
Feature | JavaScript | TypeScript | Explanation |
---|---|---|---|
Class Definition | Function-based prototype | Explicit class syntax | TypeScript’s class syntax provides better structure and type safety. |
Type Safety | Dynamic | Static | TypeScript’s static typing allows compile-time error detection. |
Method Signatures | Flexible | Enforced | TypeScript enforces the types of parameters and return values of methods. |
Example | Prototype-based approach | Class-based approach | TypeScript’s approach promotes better code organization and maintainability. |
Development Workflow and Tools: JavaScript Vs TypeScript Key Differences And Comparison
The choice between JavaScript and TypeScript significantly impacts the development workflow. Understanding the nuances of each language’s tooling ecosystem is crucial for project success. This section details the typical development workflows, common tools, and the impact on project scalability and maintainability. It also clarifies the differences in compilation processes.JavaScript’s flexibility often translates to quicker initial development, but this can lead to challenges in larger projects.
TypeScript, with its static typing, promotes early error detection and improved code maintainability. This often requires a slightly more structured approach in the initial setup, but results in more robust and maintainable codebases over time.
Typical Development Workflows
JavaScript development often involves iterative prototyping and rapid changes. Developers frequently use a live reload mechanism and rely on browser developer tools for debugging. This dynamic approach, while suitable for smaller projects, can lead to inconsistent code quality and difficulties in scaling.TypeScript projects, on the other hand, tend to use a more structured approach. The compilation step inherent in TypeScript ensures that code quality is maintained, making debugging easier in larger projects.
This structured workflow can be beneficial for projects with complex requirements or multiple developers.
Common Tools and Libraries
JavaScript development leverages a vast array of tools and libraries, often chosen based on project needs. Popular choices include Node.js for server-side development, React, Angular, and Vue.js for front-end frameworks, and numerous libraries for specific tasks. The variety can be overwhelming, and choosing the right tools can be a significant undertaking.TypeScript projects also utilize a rich ecosystem of tools and libraries.
While many libraries from the JavaScript world are also used in TypeScript, the tooling often emphasizes compatibility and interoperability with the TypeScript compiler.
Impact of Tooling on Project Scalability and Maintainability
The tools used profoundly affect a project’s scalability and maintainability. JavaScript projects can quickly become difficult to maintain if not properly structured. TypeScript, with its static typing and tooling support, helps prevent common errors and makes the codebase easier to navigate and understand as the project grows. This results in a more sustainable long-term development process.
Code Compilation Processes
JavaScript is interpreted, meaning code is executed directly by the browser or runtime environment. This allows for rapid development but can hinder the identification of errors until runtime. In contrast, TypeScript uses a compilation step. The TypeScript compiler translates TypeScript code into JavaScript code, allowing for static analysis and early error detection. This approach significantly improves code quality and maintainability.
Popular Development Tools
Tool | Functionality | JavaScript | TypeScript |
---|---|---|---|
Node.js | JavaScript runtime environment | Yes | Yes |
npm (or yarn) | Package manager | Yes | Yes |
Webpack | Module bundler | Yes | Yes |
VSCode (with extensions) | Integrated Development Environment | Yes | Yes (enhanced support for TypeScript) |
React, Angular, Vue | Front-end frameworks | Yes | Yes |
Community and Ecosystem
Both JavaScript and TypeScript boast vibrant and active communities, contributing significantly to their respective ecosystems. This translates to readily available resources, libraries, and support for developers working with either language. The sheer volume of community involvement and readily available resources impacts the ease of finding solutions to common problems, shaping the overall developer experience.
Community Size and Activity
JavaScript’s community is exceptionally large and active, encompassing a broad spectrum of developers at various skill levels. This massive community fosters a collaborative environment, leading to a wealth of readily accessible resources. TypeScript, while smaller than JavaScript’s community, is still quite active, driven by its focus on static typing and improved developer experience. The combination of dedicated developers and a strong focus on developer experience results in a significant pool of expertise readily available for support.
Available Resources and Libraries
JavaScript boasts an immense collection of libraries and frameworks, catering to diverse needs, from front-end development with React and Angular to back-end development with Node.js. The extensive ecosystem provides a wide array of tools and solutions for almost any programming task. TypeScript’s ecosystem, while smaller, is rapidly growing, offering libraries and tools that complement JavaScript’s ecosystem, facilitating seamless integration with existing JavaScript projects.
This interconnectedness of the two ecosystems contributes to the overall strength and dynamism of the development community.
Support and Documentation
JavaScript boasts comprehensive documentation and extensive online resources, including forums, tutorials, and example repositories. This abundant documentation, combined with a vast and active community, makes it easy for developers to find solutions and answers to their questions. TypeScript, though younger in comparison, is rapidly building a substantial support structure. TypeScript’s documentation is generally well-regarded for its clarity and helpfulness, making it easier to navigate the nuances of the language and its ecosystem.
Ease of Finding Solutions
Given the massive size and activity of the JavaScript community, finding solutions to common problems is usually straightforward. The abundance of resources and the rapid response from the community ensures a quick turnaround time. TypeScript, while growing, benefits from the rich ecosystem of JavaScript, allowing developers to leverage a considerable portion of the JavaScript resources and solutions. This integration and support contribute to a relatively efficient process for resolving issues.
Summary Table, JavaScript vs TypeScript key differences and comparison
Feature | JavaScript | TypeScript | Comparison |
---|---|---|---|
Community Resources | Vast and active, numerous forums, tutorials, and examples | Growing and active, with increasing documentation and support | JavaScript has a significantly larger community and more readily available resources. |
Libraries and Frameworks | Extensive ecosystem, covering front-end (React, Angular), back-end (Node.js), and more | Growing ecosystem, with libraries and tools complementing JavaScript projects | JavaScript’s ecosystem is significantly larger and more established. |
Documentation | Comprehensive and well-maintained, covering various aspects of the language and ecosystem | Clear and helpful, focusing on the language’s features and best practices | JavaScript has a broader and more extensive documentation base. |
Problem-solving ease | Generally straightforward due to the large and active community | Increasingly easier, leveraging the JavaScript ecosystem | JavaScript’s ease of problem-solving is more established due to its size. |
Performance and Scalability
JavaScript’s dynamic nature often makes it quick to develop, but this flexibility can sometimes lead to performance bottlenecks in larger applications. TypeScript, with its static typing, aims to mitigate these issues by catching potential errors during development, leading to more robust and potentially faster code. Understanding the performance characteristics of each language is crucial for choosing the right tool for a given project.
Performance Characteristics Comparison
JavaScript’s runtime environment is optimized for speed and efficiency in many cases, particularly for smaller, less complex applications. Its dynamic typing allows for rapid prototyping and iteration, but this flexibility can impact performance when dealing with large datasets or intricate computations. TypeScript, with its static typing, enables the compiler to perform more optimizations, potentially resulting in slightly faster execution times in certain scenarios.
However, the additional compilation step might introduce a slight overhead during development.
Impact of Static Typing on Performance
TypeScript’s static typing allows the compiler to perform more aggressive optimizations. By knowing the types of variables at compile time, the compiler can generate more efficient code. This often leads to reduced runtime overhead and faster execution, especially when dealing with complex algorithms or large data structures. However, this process does add a small overhead during the development phase due to the compilation step.
The gains in performance during runtime are often greater than the initial compilation time overhead.
Scalability Aspects of Applications
JavaScript’s flexibility often makes it well-suited for scaling in many projects. However, the lack of compile-time type checking can lead to subtle bugs that might manifest themselves as performance problems in large applications. TypeScript, with its static typing, allows developers to catch potential errors early, resulting in more robust code and better scalability. The increased code maintainability and early error detection contribute to easier scaling as the application grows.
Factors Influencing Performance Differences
Several factors influence the performance difference between JavaScript and TypeScript. These include the complexity of the application, the specific algorithms used, and the size of the dataset being processed. In addition, the quality of the code itself, including efficient algorithms and optimized data structures, is a critical factor for both languages. The specific implementation details of the code, including how it interacts with external libraries or APIs, can also influence performance.
For instance, if a large portion of the code relies on external libraries that are not optimized, this can impact performance regardless of the language used.
Benchmark Results and Performance Metrics
A definitive comparison requires benchmarks. Unfortunately, a single, universally accepted benchmark doesn’t exist. Performance depends heavily on the specific use case. However, in general, for projects with a high level of complexity and reliance on computationally intensive operations, TypeScript’s static typing can offer noticeable performance improvements. For smaller, less complex projects, the difference might be negligible.
Category | JavaScript | TypeScript | Notes |
---|---|---|---|
Basic Operations | Fast | Fast | Both languages perform comparable basic operations. |
Complex Calculations | Potentially slower due to runtime type checking | Potentially faster due to compile-time type checking | TypeScript’s static typing enables more optimization in complex scenarios. |
Large Datasets | Performance might degrade | Performance may improve with careful implementation | Static typing can help prevent runtime errors that can impact performance with large datasets. |
Real-world Applications | Proven scalability for many projects | Can improve scalability through earlier error detection | TypeScript aids scalability by enabling early bug identification. |
Use Cases and Applicability
Choosing between JavaScript and TypeScript often hinges on project requirements and desired outcomes. JavaScript’s flexibility and broad ecosystem make it ideal for rapid prototyping and smaller projects. TypeScript, on the other hand, provides enhanced maintainability and scalability for larger, complex applications. The trade-offs involve balancing initial development speed against long-term code maintainability and potential future growth.JavaScript’s dynamism and inherent ease of use are attractive for quick development cycles, particularly in smaller web applications or interactive elements.
TypeScript’s static typing, while demanding a slightly steeper learning curve, provides long-term advantages in larger projects, reducing errors and improving maintainability as the codebase expands.
JavaScript Preferred Use Cases
JavaScript shines in situations requiring rapid development and a flexible approach. This often translates to projects where the initial scope is uncertain or where rapid iteration is paramount.
- Prototyping and Proof-of-Concept (PoC) projects: JavaScript’s quick setup and straightforward syntax allow for rapid prototyping, enabling developers to quickly validate ideas and demonstrate functionality.
- Small-scale applications: Simple web pages, interactive elements, or small-scale single-page applications (SPAs) often benefit from JavaScript’s straightforward implementation and vast library support. Its lightweight nature avoids the overhead of TypeScript’s static typing.
- Front-end development with existing JavaScript frameworks: Frameworks like React, Angular, and Vue.js are deeply integrated with JavaScript. In projects leveraging these frameworks, JavaScript’s compatibility and ease of integration are often the preferred choice.
- Dynamic content updates: JavaScript excels at dynamically updating web pages without requiring a full page reload. This makes it suitable for applications like chat applications, real-time dashboards, or collaborative editing tools.
TypeScript Preferred Use Cases
TypeScript’s static typing and robust tooling become invaluable as projects grow in size and complexity. These advantages lead to better maintainability, reduced errors, and improved overall code quality.
- Large-scale applications: Complex web applications, enterprise-level software, or any project expected to have a long lifespan and many developers benefit from TypeScript’s structure and type safety.
- Team projects: When multiple developers work on a project, TypeScript’s strong typing enhances collaboration and prevents ambiguity, fostering a consistent codebase.
- Complex data structures: TypeScript’s robust type system enables handling complex data structures with confidence, minimizing errors that can arise from unexpected data types in large-scale applications.
- Maintaining codebases: When existing JavaScript codebases need to be updated, maintained, or expanded, TypeScript provides a structured approach to introducing type safety without completely rewriting the code.
Trade-offs and Project Requirements
The choice between JavaScript and TypeScript often involves balancing initial development speed with long-term maintainability.
- Initial development time: JavaScript’s simpler syntax often leads to faster initial development, while TypeScript’s type system requires more upfront time investment.
- Maintainability: TypeScript’s type system contributes to easier maintenance and code understanding, particularly in large projects. This is offset by a slightly increased initial learning curve.
- Team expertise: If a team lacks experience with TypeScript, JavaScript might be the more accessible option initially. However, in the long term, the advantages of TypeScript’s maintainability can outweigh the initial learning curve.
Influence of Project Requirements
Specific project requirements significantly impact the language choice. Factors like the project’s size, expected lifespan, team experience, and the need for scalability play critical roles.
- Project Size: For smaller projects, JavaScript’s simplicity often outweighs the extra complexity of TypeScript.
- Scalability Needs: If the project is expected to grow significantly, TypeScript’s enhanced type system can improve scalability and maintainability.
- Team Experience: If the team has prior experience with JavaScript, the transition to TypeScript might be smoother.
- Maintainability Goals: If long-term maintainability is crucial, TypeScript’s type system and tooling offer significant advantages.
Use Case Suitability Table
This table summarizes the suitability of each language for different use cases.
Use Case | JavaScript | TypeScript | Suitability Rationale |
---|---|---|---|
Small, simple web apps | High | Medium | Faster development, easier learning curve |
Large-scale, complex apps | Low | High | Improved maintainability, reduced errors |
Applications requiring real-time updates | High | Medium | JavaScript’s efficiency for dynamic content |
Projects with extensive data handling | Medium | High | TypeScript’s type safety improves data management |
Practical Examples
JavaScript and TypeScript, while sharing a core language foundation, differ significantly in their approach to type safety and development workflow. Understanding these differences becomes clear through practical examples, highlighting how type annotations in TypeScript can enhance code maintainability and reduce errors. This section will demonstrate these concepts through simple functions, complex application comparisons, and a direct comparison table.
Simple Function Examples
JavaScript’s flexibility allows for functions without explicit type declarations. This can lead to potential runtime errors if the input data doesn’t match the expected type. TypeScript’s type system ensures that the function’s input and expected output types are specified.
javascript
// JavaScript function
function add(a, b)
return a + b;
console.log(add(5, 3)); // Output: 8
console.log(add("hello", " world")); // Output: hello world
typescript
// TypeScript function
function add(a: number, b: number): number
return a + b;
console.log(add(5, 3)); // Output: 8
// console.log(add("hello", " world")); // Error: Type 'string' is not assignable to type 'number'
The TypeScript example explicitly defines `a` and `b` as numbers, and the function’s return type as a number. This prevents the common error of passing strings to the function, catching potential issues at compile time.
Complex Application Comparison
Consider a simple to-do list application. A JavaScript implementation might rely on flexible data structures, but this flexibility can lead to issues with maintainability and scalability as the application grows. TypeScript, on the other hand, provides compile-time checks and allows for more organized data structures, enhancing the overall maintainability.
Comparison Table
This table demonstrates the difference in handling a common function in both languages.
Task | JavaScript | TypeScript | Explanation |
---|---|---|---|
Calculate the area of a rectangle |
|
|
TypeScript’s type annotations (e.g., `length: number`, `width: number`) help catch errors earlier by specifying the expected data types. |
Validate user input |
|
|
TypeScript’s type system (e.g., `input: string`) clarifies the expected data type, enabling compile-time validation and preventing runtime errors. |
Final Review
In conclusion, TypeScript offers enhanced code organization and maintainability through its static typing, making it suitable for larger projects requiring robust codebases. JavaScript remains the preferred choice for its speed and flexibility, making it ideal for smaller projects or rapid prototyping. The choice between them depends on the project’s specific needs and the developer’s preferences.
Both languages are valuable assets in the web development landscape.
Post Comment